使用Python多线程一直运行,可以使用线程的无限循环、主线程等待、守护线程等方式、合理管理线程生命周期。其中一种常见的方法是使用无限循环和主线程等待来保持线程的持续运行。通过在子线程中使用无限循环,可以确保线程持续执行任务,同时使用主线程等待来确保主线程不会在子线程完成之前退出。
一、无限循环
无限循环是保持线程持续运行的最简单方法之一。通过在子线程中使用while True
循环,我们可以确保线程一直运行,直到明确终止它。
import threading
def worker():
while True:
print("Thread is running")
time.sleep(1) # 添加延迟以避免过度占用CPU
thread = threading.Thread(target=worker)
thread.start()
这种方法虽然简单,但需要小心管理线程的生命周期,以避免资源泄漏或线程无法终止的问题。
二、主线程等待
主线程等待是另一种确保多线程程序持续运行的方法。通过使用thread.join()
,我们可以让主线程等待子线程完成,从而避免主线程过早退出。
import threading
import time
def worker():
while True:
print("Thread is running")
time.sleep(1) # 添加延迟以避免过度占用CPU
thread = threading.Thread(target=worker)
thread.start()
thread.join() # 主线程等待子线程完成
这种方法确保主线程在子线程完成之前不会退出,从而保证了多线程程序的持续运行。
三、守护线程
守护线程(Daemon Thread)是另一种管理线程生命周期的方式。设置线程为守护线程后,主线程退出时,守护线程会自动结束。
import threading
import time
def worker():
while True:
print("Thread is running")
time.sleep(1) # 添加延迟以避免过度占用CPU
thread = threading.Thread(target=worker)
thread.daemon = True # 设置为守护线程
thread.start()
time.sleep(5) # 主线程等待一段时间后退出
print("Main thread exiting")
守护线程适用于那些不需要显式终止的后台任务,但需要注意的是,一旦主线程退出,所有守护线程会立即终止。
四、使用threading.Event
控制线程
使用threading.Event
可以更灵活地控制线程的启动和停止。通过在无限循环中检查事件对象的状态,可以实现线程的有序启动和停止。
import threading
import time
def worker(stop_event):
while not stop_event.is_set():
print("Thread is running")
time.sleep(1) # 添加延迟以避免过度占用CPU
print("Thread stopping")
stop_event = threading.Event()
thread = threading.Thread(target=worker, args=(stop_event,))
thread.start()
time.sleep(5) # 主线程等待一段时间
stop_event.set() # 触发事件,通知子线程停止
thread.join() # 等待子线程完成
print("Main thread exiting")
这种方法通过事件对象实现了对线程的灵活控制,适用于需要有序启动和停止的场景。
五、使用concurrent.futures.ThreadPoolExecutor
concurrent.futures.ThreadPoolExecutor
提供了更高级的线程池管理功能。通过线程池,可以方便地管理多个线程,并确保线程池在主线程退出时自动关闭。
from concurrent.futures import ThreadPoolExecutor
import time
def worker():
while True:
print("Thread is running")
time.sleep(1) # 添加延迟以避免过度占用CPU
with ThreadPoolExecutor(max_workers=1) as executor:
future = executor.submit(worker)
time.sleep(5) # 主线程等待一段时间后退出
print("Main thread exiting")
线程池适用于需要管理多个线程的场景,并且提供了便捷的线程管理功能。
六、线程安全和资源管理
在多线程编程中,确保线程安全和合理管理资源至关重要。可以使用threading.Lock
或threading.RLock
来保护共享资源,避免线程竞争和数据不一致的问题。
import threading
import time
lock = threading.Lock()
def worker():
while True:
with lock:
print("Thread is running")
time.sleep(1) # 添加延迟以避免过度占用CPU
thread = threading.Thread(target=worker)
thread.start()
thread.join() # 主线程等待子线程完成
通过使用锁,可以确保对共享资源的访问是线程安全的,从而避免数据不一致和竞争条件。
七、使用queue.Queue
进行线程间通信
queue.Queue
提供了线程安全的队列,可以方便地在线程之间传递数据。通过队列,可以实现生产者-消费者模型,确保线程之间的通信和协作。
import threading
import queue
import time
q = queue.Queue()
def producer():
while True:
item = "data"
q.put(item)
print("Produced:", item)
time.sleep(1) # 添加延迟以避免过度占用CPU
def consumer():
while True:
item = q.get()
print("Consumed:", item)
q.task_done()
time.sleep(1) # 添加延迟以避免过度占用CPU
thread_producer = threading.Thread(target=producer)
thread_consumer = threading.Thread(target=consumer)
thread_producer.start()
thread_consumer.start()
thread_producer.join()
thread_consumer.join()
通过使用队列,可以实现线程间的数据传递和任务分配,从而提高程序的灵活性和可维护性。
八、使用threading.Timer
实现定时任务
threading.Timer
可以实现定时任务,通过定时器,可以在指定时间间隔内执行任务。
import threading
def periodic_task():
print("Periodic task executed")
timer = threading.Timer(1, periodic_task)
timer.start()
timer = threading.Timer(1, periodic_task)
timer.start()
定时器适用于需要定期执行任务的场景,可以方便地实现定时任务调度。
九、使用asyncio
实现异步任务
asyncio
是Python中的异步编程库,提供了协程和事件循环的支持。通过asyncio
,可以实现高效的异步任务调度和管理。
import asyncio
async def worker():
while True:
print("Asyncio task running")
await asyncio.sleep(1) # 异步睡眠,避免过度占用CPU
async def main():
task = asyncio.create_task(worker())
await asyncio.sleep(5) # 主任务等待一段时间后退出
task.cancel() # 取消子任务
asyncio.run(main())
asyncio
适用于需要高并发和高性能的异步任务调度场景,提供了灵活的异步编程支持。
十、使用multiprocessing
实现进程间并行
multiprocessing
模块提供了多进程支持,通过多进程,可以实现进程间的并行执行,适用于需要充分利用多核CPU的场景。
import multiprocessing
import time
def worker():
while True:
print("Process is running")
time.sleep(1) # 添加延迟以避免过度占用CPU
process = multiprocessing.Process(target=worker)
process.start()
process.join() # 主进程等待子进程完成
多进程适用于需要充分利用多核CPU的高并发场景,通过多进程,可以实现进程间的并行执行。
以上是使用Python多线程一直运行的多种方法和技巧。根据具体需求,可以选择合适的方法来实现多线程程序的持续运行。通过合理管理线程生命周期、确保线程安全和资源管理,可以提高多线程程序的性能和可靠性。
相关问答FAQs:
如何在Python中实现多线程的长期运行?
在Python中,可以使用threading
模块来创建和管理线程。为了让线程持续运行,可以在线程函数中使用一个无限循环,并在适当的时机使用条件变量或事件来控制线程的终止。同时,确保合理使用time.sleep()
来避免CPU占用过高。可以通过设置一个标志位来结束线程,从而实现线程的长期运行和安全退出。
多线程在Python中有哪些常见的应用场景?
多线程在Python中适用于I/O密集型任务,如网络请求、文件读写和数据库操作。在这些场景下,多线程可以显著提高程序的响应速度和并发处理能力。对于CPU密集型任务,Python的GIL(全局解释器锁)可能会限制多线程的性能,因此在这些情况下,可以考虑使用多进程或异步编程。
如何处理Python多线程中的共享数据问题?
在Python的多线程环境中,多个线程可能会访问和修改共享数据,这可能导致数据不一致。为了避免这种情况,可以使用threading.Lock
来锁定共享资源,确保在同一时间只有一个线程可以访问该资源。还可以使用threading.RLock
,它允许同一线程多次获得锁,适用于递归场景。同时,使用条件变量和事件可以帮助线程之间进行更复杂的同步。
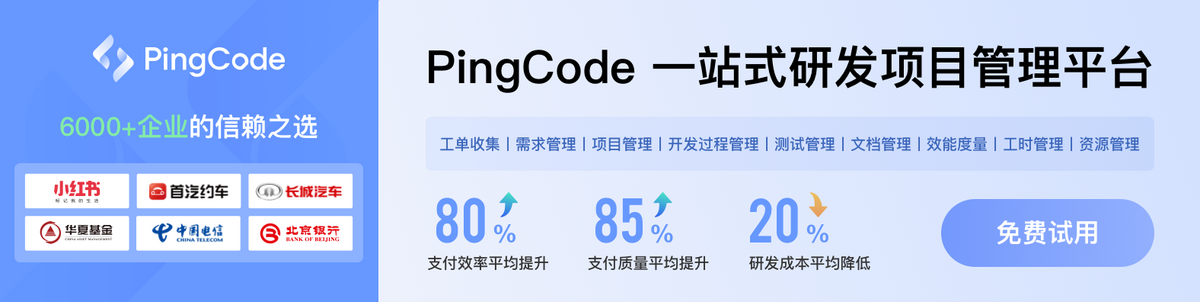