Python获取响应正文中的函数可以通过解析响应内容、使用正则表达式或其他文本解析方法来实现。 例如,使用requests
库获取响应内容,然后使用re
库解析出函数定义。这是其中一种方法,接下来详细介绍这种方法。
一、解析响应内容
在获取响应正文内容之前,我们需要先获取响应。这里可以使用Python的requests
库来发送HTTP请求并获取响应。
import requests
url = "http://example.com"
response = requests.get(url)
content = response.text
通过上述代码,我们获取了指定URL的响应内容,并将其存储在变量content
中。
二、使用正则表达式提取函数
接下来,使用正则表达式提取响应正文中的函数定义。假设我们要提取所有以def
关键字定义的Python函数:
import re
pattern = r'def\s+(\w+)\s*\(.*?\):'
functions = re.findall(pattern, content)
在上述代码中,我们定义了一个正则表达式pattern
,用于匹配函数定义。re.findall
函数会返回一个包含所有匹配项的列表,即所有函数名。
三、逐步解析响应内容
我们可以进一步解析函数的完整定义,而不仅仅是函数名。下面是一个示例代码,展示如何提取完整函数定义:
import re
def extract_functions(content):
pattern = re.compile(r'def\s+(\w+)\s*\(.*?\):\s*(.*?)(?=\ndef|\Z)', re.S)
matches = pattern.findall(content)
return matches
url = "http://example.com"
response = requests.get(url)
content = response.text
functions = extract_functions(content)
for func_name, func_body in functions:
print(f"Function Name: {func_name}")
print(f"Function Body: {func_body}")
print("-" * 40)
在上述代码中,正则表达式模式包含了函数定义的关键部分,并使用re.S
标志来确保点号.
可以匹配换行符。extract_functions
函数会返回一个包含所有匹配项的列表,每个匹配项是一个元组,包含函数名和函数体。
四、处理不同类型的响应内容
根据响应内容的不同类型,可能需要进行额外的处理。例如,如果响应是HTML页面,我们可以使用BeautifulSoup
库来解析HTML,并提取出嵌入的Python代码:
from bs4 import BeautifulSoup
import requests
import re
def extract_python_functions_from_html(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
script_tags = soup.find_all('script', type='text/python')
python_code = "\n".join(tag.string for tag in script_tags if tag.string)
return extract_functions(python_code)
url = "http://example.com"
response = requests.get(url)
html_content = response.text
functions = extract_python_functions_from_html(html_content)
for func_name, func_body in functions:
print(f"Function Name: {func_name}")
print(f"Function Body: {func_body}")
print("-" * 40)
在上述代码中,我们使用BeautifulSoup
库解析HTML响应,并提取所有类型为text/python
的<script>
标签内容。然后,调用extract_functions
函数提取函数定义。
五、处理其他类型的响应内容
除了HTML页面,可能还需要处理其他类型的响应内容,例如JSON响应。对于JSON响应,可以使用json
库解析响应内容,并提取嵌入的Python代码:
import json
import requests
import re
def extract_python_functions_from_json(json_content):
python_code = "\n".join(json_content.values())
return extract_functions(python_code)
url = "http://example.com"
response = requests.get(url)
json_content = response.json()
functions = extract_python_functions_from_json(json_content)
for func_name, func_body in functions:
print(f"Function Name: {func_name}")
print(f"Function Body: {func_body}")
print("-" * 40)
在上述代码中,我们假设JSON响应包含Python代码作为值。通过json
库解析响应内容,并提取所有嵌入的Python代码。然后,调用extract_functions
函数提取函数定义。
六、处理复杂的响应内容
对于更复杂的响应内容,可能需要结合多种方法来提取函数定义。例如,可能需要结合HTML解析、正则表达式、字符串操作等方法。以下是一个示例,展示如何处理复杂的响应内容:
from bs4 import BeautifulSoup
import requests
import re
def extract_functions(content):
pattern = re.compile(r'def\s+(\w+)\s*\(.*?\):\s*(.*?)(?=\ndef|\Z)', re.S)
matches = pattern.findall(content)
return matches
def extract_python_functions_from_complex_html(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
script_tags = soup.find_all('script', type='text/python')
python_code = "\n".join(tag.string for tag in script_tags if tag.string)
additional_code = ""
for tag in soup.find_all('code'):
additional_code += tag.get_text() + "\n"
combined_code = python_code + "\n" + additional_code
return extract_functions(combined_code)
url = "http://example.com"
response = requests.get(url)
html_content = response.text
functions = extract_python_functions_from_complex_html(html_content)
for func_name, func_body in functions:
print(f"Function Name: {func_name}")
print(f"Function Body: {func_body}")
print("-" * 40)
在上述代码中,我们结合了HTML解析和字符串操作,从响应内容中提取嵌入的Python代码。然后,调用extract_functions
函数提取函数定义。
七、优化代码
为了提高代码的可读性和可维护性,可以将上述代码进行封装和优化。例如,定义一个通用的extract_functions_from_content
函数,根据响应内容的类型调用相应的解析函数:
from bs4 import BeautifulSoup
import requests
import re
import json
def extract_functions(content):
pattern = re.compile(r'def\s+(\w+)\s*\(.*?\):\s*(.*?)(?=\ndef|\Z)', re.S)
matches = pattern.findall(content)
return matches
def extract_python_functions_from_html(html_content):
soup = BeautifulSoup(html_content, 'html.parser')
script_tags = soup.find_all('script', type='text/python')
python_code = "\n".join(tag.string for tag in script_tags if tag.string)
return extract_functions(python_code)
def extract_python_functions_from_json(json_content):
python_code = "\n".join(json_content.values())
return extract_functions(python_code)
def extract_functions_from_content(content, content_type):
if content_type == 'html':
return extract_python_functions_from_html(content)
elif content_type == 'json':
return extract_python_functions_from_json(content)
else:
raise ValueError(f"Unsupported content type: {content_type}")
url = "http://example.com"
response = requests.get(url)
content_type = response.headers.get('Content-Type')
if 'html' in content_type:
content_type = 'html'
elif 'json' in content_type:
content_type = 'json'
else:
raise ValueError(f"Unsupported content type: {content_type}")
content = response.text if content_type == 'html' else response.json()
functions = extract_functions_from_content(content, content_type)
for func_name, func_body in functions:
print(f"Function Name: {func_name}")
print(f"Function Body: {func_body}")
print("-" * 40)
在上述代码中,我们定义了一个通用的extract_functions_from_content
函数,根据响应内容的类型调用相应的解析函数。通过这种方式,可以轻松扩展支持更多类型的响应内容。
总结:
通过上述方法,我们可以在Python中获取响应正文中的函数。具体步骤包括:获取响应内容、使用正则表达式或其他文本解析方法提取函数定义,以及处理不同类型的响应内容。根据具体需求,可以结合多种方法进行解析和提取。通过优化和封装代码,可以提高代码的可读性和可维护性。
相关问答FAQs:
如何在Python中提取HTTP响应的正文内容?
在Python中,您可以使用requests
库来发送HTTP请求并获取响应的正文内容。使用response.text
可以获取到响应的文本。具体代码示例如下:
import requests
response = requests.get('https://api.example.com/data')
content = response.text
print(content)
这个方法适用于大多数文本格式的响应,例如HTML或JSON。
如何处理获取的响应正文中的JSON数据?
如果HTTP响应的正文是JSON格式,您可以使用response.json()
方法直接将其解析为Python字典。这使得后续的数据处理更加方便。例如:
import requests
response = requests.get('https://api.example.com/data')
data = response.json()
print(data['key']) # 获取JSON中的特定字段
这种方式可以轻松访问JSON结构中的各个元素。
在获取HTTP响应正文时,如何处理异常情况?
在进行HTTP请求时,可能会遇到各种异常情况,例如连接错误或请求超时。为此,您可以使用try-except
语句来捕获这些异常并进行处理。例如:
import requests
try:
response = requests.get('https://api.example.com/data')
response.raise_for_status() # 检查请求是否成功
content = response.text
except requests.exceptions.RequestException as e:
print(f'请求失败: {e}')
这种方式可以确保在发生错误时,您的程序不会崩溃,并可以输出错误信息以供调试。
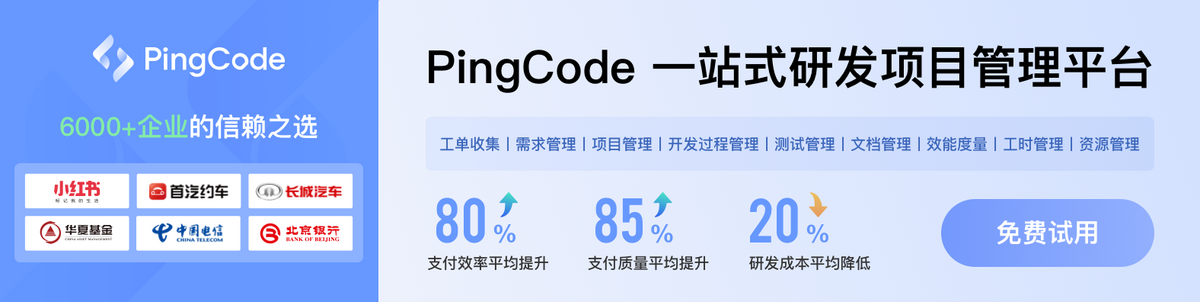