在Python中统计每个单词的个数是一个常见的问题,可以通过多种方法来实现。使用字典、collections模块、正则表达式是一些常见的方法。以下将详细介绍如何使用这些方法来统计每个单词的个数,并提供相关的代码示例。
一、使用字典
字典是一种非常适合存储键值对的数据结构,我们可以利用它来统计每个单词出现的次数。
1、代码示例
def count_words(text):
words = text.split()
word_count = {}
for word in words:
word = word.lower() # 将单词转换为小写,确保统计不区分大小写
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
return word_count
text = "This is a test. This test is only a test."
word_count = count_words(text)
print(word_count)
2、详细描述
上面的代码首先将输入的文本按照空格分割成单词列表,然后通过循环遍历每个单词,将其转换为小写以确保统计不区分大小写。接着,利用字典的键值对存储每个单词的出现次数。如果单词已经在字典中,则将对应的值加1;如果单词不在字典中,则将其添加到字典中并将值设为1。
二、使用collections模块
collections
模块提供了一个Counter
类,可以更加方便地统计单词的个数。
1、代码示例
from collections import Counter
def count_words(text):
words = text.split()
word_count = Counter(words)
return word_count
text = "This is a test. This test is only a test."
word_count = count_words(text)
print(word_count)
2、详细描述
在上述代码中,我们首先将文本按照空格分割成单词列表,然后直接使用Counter
类来统计每个单词的出现次数。Counter
类是collections
模块中的一个子类,专门用于计数的容器。它的使用非常简单,只需将单词列表传递给Counter
类即可得到一个包含单词计数的字典。
三、使用正则表达式
正则表达式是一种强大的字符串处理工具,可以用来匹配文本中的单词。
1、代码示例
import re
from collections import Counter
def count_words(text):
words = re.findall(r'\b\w+\b', text.lower())
word_count = Counter(words)
return word_count
text = "This is a test. This test is only a test."
word_count = count_words(text)
print(word_count)
2、详细描述
在这个示例中,我们使用正则表达式r'\b\w+\b'
来匹配文本中的单词。\b
表示单词边界,\w+
表示一个或多个字母或数字。我们将文本转换为小写后,通过re.findall
函数找到所有匹配的单词,然后使用Counter
类来统计每个单词的出现次数。
四、处理复杂文本
在实际应用中,文本可能包含标点符号、特殊字符等复杂情况。我们可以通过预处理文本来处理这些复杂情况。
1、代码示例
import re
from collections import Counter
def preprocess_text(text):
text = re.sub(r'[^\w\s]', '', text) # 去除标点符号
return text.lower()
def count_words(text):
text = preprocess_text(text)
words = text.split()
word_count = Counter(words)
return word_count
text = "Hello, world! This is a test. This test is only a test."
word_count = count_words(text)
print(word_count)
2、详细描述
在这个示例中,我们定义了一个preprocess_text
函数,用来去除文本中的标点符号并将其转换为小写。我们使用正则表达式r'[^\w\s]'
匹配非字母数字和空格的字符,并将其替换为空字符串。然后,我们将预处理后的文本传递给count_words
函数,统计每个单词的出现次数。
五、处理大文本数据
对于大文本数据,我们可以使用生成器来处理文本,避免一次性加载所有数据到内存中。
1、代码示例
import re
from collections import Counter
def preprocess_text(text):
text = re.sub(r'[^\w\s]', '', text) # 去除标点符号
return text.lower()
def count_words(file_path):
word_count = Counter()
with open(file_path, 'r') as file:
for line in file:
line = preprocess_text(line)
words = line.split()
word_count.update(words)
return word_count
file_path = 'large_text_file.txt'
word_count = count_words(file_path)
print(word_count)
2、详细描述
在这个示例中,我们定义了一个count_words
函数,接受文件路径作为参数。我们使用生成器逐行读取文件内容,并对每行进行预处理,去除标点符号并转换为小写。然后,我们将每行的单词列表传递给Counter
类的update
方法来更新单词计数。这样可以有效地处理大文本数据,避免一次性加载所有数据到内存中。
六、统计结果的可视化
我们可以使用matplotlib
库将单词统计结果进行可视化,生成柱状图或词云等图表。
1、代码示例(柱状图)
import re
from collections import Counter
import matplotlib.pyplot as plt
def preprocess_text(text):
text = re.sub(r'[^\w\s]', '', text) # 去除标点符号
return text.lower()
def count_words(text):
text = preprocess_text(text)
words = text.split()
word_count = Counter(words)
return word_count
def plot_word_count(word_count):
words, counts = zip(*word_count.items())
plt.figure(figsize=(10, 5))
plt.bar(words, counts)
plt.xlabel('Words')
plt.ylabel('Counts')
plt.title('Word Count')
plt.xticks(rotation=90)
plt.show()
text = "Hello, world! This is a test. This test is only a test."
word_count = count_words(text)
plot_word_count(word_count)
2、详细描述
在这个示例中,我们定义了一个plot_word_count
函数,用于生成单词计数的柱状图。我们使用matplotlib
库来绘制图表,将单词和对应的计数分别作为x轴和y轴的数据。通过调用plt.bar
函数生成柱状图,并设置图表的标签和标题。最后,通过plt.show
函数显示图表。
3、代码示例(词云)
import re
from collections import Counter
from wordcloud import WordCloud
import matplotlib.pyplot as plt
def preprocess_text(text):
text = re.sub(r'[^\w\s]', '', text) # 去除标点符号
return text.lower()
def count_words(text):
text = preprocess_text(text)
words = text.split()
word_count = Counter(words)
return word_count
def plot_wordcloud(word_count):
wordcloud = WordCloud(width=800, height=400, background_color='white').generate_from_frequencies(word_count)
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
text = "Hello, world! This is a test. This test is only a test."
word_count = count_words(text)
plot_wordcloud(word_count)
4、详细描述
在这个示例中,我们定义了一个plot_wordcloud
函数,用于生成单词计数的词云图。我们使用wordcloud
库中的WordCloud
类来生成词云图,并将单词计数结果传递给generate_from_frequencies
方法。然后,通过matplotlib
库来显示词云图,设置图像的尺寸和背景颜色,并通过plt.imshow
函数显示词云图。
七、多语言支持
对于包含多种语言的文本,我们可以使用nltk
库进行分词处理,确保统计结果准确。
1、代码示例
import re
from collections import Counter
import nltk
nltk.download('punkt')
def preprocess_text(text):
text = re.sub(r'[^\w\s]', '', text) # 去除标点符号
return text.lower()
def count_words(text):
text = preprocess_text(text)
words = nltk.word_tokenize(text)
word_count = Counter(words)
return word_count
text = "Hello, world! 你好,世界!This is a test. 这是一个测试。"
word_count = count_words(text)
print(word_count)
2、详细描述
在这个示例中,我们使用nltk
库中的word_tokenize
函数进行分词处理,以支持多种语言的文本。首先,我们对文本进行预处理,去除标点符号并转换为小写。然后,我们调用word_tokenize
函数对文本进行分词,得到包含所有单词的列表。最后,我们使用Counter
类统计每个单词的出现次数。
八、总结
通过本文的介绍,我们了解了如何使用Python统计每个单词的个数,包括使用字典、collections模块、正则表达式等方法。同时,我们还介绍了如何处理复杂文本、处理大文本数据、统计结果的可视化以及多语言支持等方面的内容。希望这些方法和示例能够帮助你更好地理解和应用Python进行单词计数。
相关问答FAQs:
如何使用Python快速统计文本中的单词数量?
可以利用Python的内置方法和库来轻松统计文本中的单词数量。首先,可以使用split()
方法将文本拆分为单词,然后使用len()
函数计算单词的总数。示例代码如下:
text = "这是一段测试文本"
word_count = len(text.split())
print(word_count)
以上代码将返回文本中的单词总数。
有哪些Python库可以帮助统计单词频率?
Python中有多个库可以帮助实现单词频率统计。例如,collections.Counter
是一个非常有用的工具。它可以轻松地统计文本中每个单词的出现次数,示例代码如下:
from collections import Counter
text = "这是一段测试文本,测试文本很简单"
word_count = Counter(text.split())
print(word_count)
这段代码会输出每个单词及其对应的出现次数。
如何处理文本中的标点符号和大小写?
在统计单词个数时,标点符号和大小写可能会影响结果。可以使用str.lower()
方法将文本转换为小写,并使用re
库的正则表达式来去除标点符号。例如:
import re
text = "这是一段测试文本,测试文本很简单!"
cleaned_text = re.sub(r'[^\w\s]', '', text.lower())
word_count = len(cleaned_text.split())
print(word_count)
这种方法可以确保统计结果更加准确和一致。
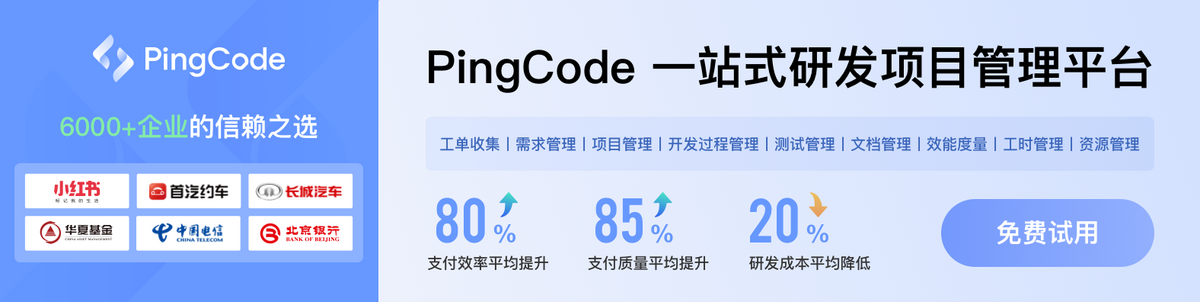