Python将a转化为b的方法包括使用字典、列表、字符串替换、正则表达式、函数映射等。 其中,使用字典进行映射转换是最常用且高效的方法之一。通过创建一个字典,将字符'a'映射到字符'b',可以快速实现转换。此外,还可以利用Python的字符串方法和正则表达式进行复杂的模式匹配和替换操作。
一、使用字典进行映射转换
字典是一种键值对数据结构,可以用来存储映射关系。通过查找字典中的键,可以快速获取对应的值,从而实现a到b的转换。
def convert_a_to_b(input_string):
conversion_dict = {'a': 'b'}
result = ''.join([conversion_dict.get(char, char) for char in input_string])
return result
input_string = "apple and banana"
converted_string = convert_a_to_b(input_string)
print(converted_string) # 输出: bpple bnd bnbnb
在这个示例中,我们定义了一个转换字典conversion_dict
,将字符'a'映射到字符'b'。然后,通过列表解析和join
方法,将输入字符串中的每个字符进行转换。
二、使用字符串的replace
方法
Python的字符串方法replace
可以用于简单的字符串替换操作。对于单个字符的替换,这种方法非常直观且易于使用。
input_string = "apple and banana"
converted_string = input_string.replace('a', 'b')
print(converted_string) # 输出: bpple bnd bnbnb
在这个示例中,replace
方法直接将所有的'a'替换为'b',实现了字符串的转换。
三、使用正则表达式进行复杂替换
当需要进行更复杂的模式匹配和替换时,可以使用Python的re
模块。正则表达式提供了强大的模式匹配功能,可以实现更复杂的转换规则。
import re
def convert_a_to_b(input_string):
return re.sub(r'a', 'b', input_string)
input_string = "apple and banana"
converted_string = convert_a_to_b(input_string)
print(converted_string) # 输出: bpple bnd bnbnb
在这个示例中,re.sub
函数使用正则表达式r'a'
匹配所有的'a',并将其替换为'b'。
四、使用函数映射进行自定义转换
通过定义一个转换函数,可以实现更灵活的转换规则。使用map
函数将转换函数应用于输入字符串的每个字符。
def convert_char(char):
if char == 'a':
return 'b'
return char
input_string = "apple and banana"
converted_string = ''.join(map(convert_char, input_string))
print(converted_string) # 输出: bpple bnd bnbnb
在这个示例中,我们定义了一个转换函数convert_char
,用于将字符'a'转换为字符'b'。通过map
函数,将convert_char
函数应用于输入字符串的每个字符。
五、处理不同类型的数据
在实际应用中,数据类型可能不仅仅是字符串,还可能涉及列表、元组等其他数据结构。为了实现更通用的转换,可以编写递归函数处理不同类型的数据。
def convert_a_to_b(data):
if isinstance(data, str):
return data.replace('a', 'b')
elif isinstance(data, list):
return [convert_a_to_b(item) for item in data]
elif isinstance(data, tuple):
return tuple(convert_a_to_b(item) for item in data)
elif isinstance(data, dict):
return {key: convert_a_to_b(value) for key, value in data.items()}
else:
return data
data = {
"name": "apple",
"fruits": ["banana", "grape", "pear"],
"nested": {
"char": "a"
}
}
converted_data = convert_a_to_b(data)
print(converted_data)
输出:
{'name': 'bpple', 'fruits': ['bbnbnb', 'grbpe', 'pebr'], 'nested': {'char': 'b'}}
在这个示例中,我们定义了一个递归函数convert_a_to_b
,用于处理不同类型的数据结构。该函数可以处理字符串、列表、元组和字典,并递归地将字符'a'转换为字符'b'。
六、结合多种方法实现复杂转换
在实际项目中,可能需要结合多种方法来实现复杂的转换需求。例如,将字典映射和正则表达式结合使用,可以实现复杂的模式匹配和转换。
import re
def convert_a_to_b(input_string, conversion_dict):
def replace(match):
return conversion_dict.get(match.group(0), match.group(0))
pattern = re.compile('|'.join(map(re.escape, conversion_dict.keys())))
return pattern.sub(replace, input_string)
conversion_dict = {
'a': 'b',
'apple': 'fruit',
'banana': 'yellow fruit'
}
input_string = "apple and banana are a common fruit"
converted_string = convert_a_to_b(input_string, conversion_dict)
print(converted_string) # 输出: fruit and yellow fruit are b common fruit
在这个示例中,我们结合字典映射和正则表达式,实现了复杂的模式匹配和转换。通过re.compile
生成匹配模式,并使用sub
方法进行替换操作。
七、性能优化和大数据处理
在处理大数据时,转换操作的性能可能成为瓶颈。可以通过优化代码,提高转换操作的效率。例如,使用生成器和流式处理技术,避免一次性加载大量数据到内存中。
def convert_a_to_b_stream(input_stream, conversion_dict):
pattern = re.compile('|'.join(map(re.escape, conversion_dict.keys())))
def replace(match):
return conversion_dict.get(match.group(0), match.group(0))
for line in input_stream:
yield pattern.sub(replace, line)
conversion_dict = {
'a': 'b',
'apple': 'fruit',
'banana': 'yellow fruit'
}
with open('large_input.txt', 'r') as input_file:
with open('converted_output.txt', 'w') as output_file:
for converted_line in convert_a_to_b_stream(input_file, conversion_dict):
output_file.write(converted_line)
在这个示例中,我们定义了一个流式处理函数convert_a_to_b_stream
,用于逐行读取和转换大文件的数据。通过生成器yield
,避免一次性加载大量数据到内存中,从而提高处理效率。
八、总结
通过以上方法,可以灵活地将字符'a'转换为字符'b',并适应不同类型的数据和复杂的转换需求。无论是简单的字符串替换,还是复杂的模式匹配和大数据处理,都可以找到合适的方法来实现。掌握这些技术,可以帮助开发者在实际项目中高效地处理数据转换任务。
相关问答FAQs:
在Python中,如何将数据类型从a转化为b?
在Python中,数据类型的转换可以使用内置函数。例如,可以使用int()
函数将字符串或浮点数转化为整数,使用str()
函数将整数或浮点数转化为字符串。如果需要将一个列表转换为集合,可以使用set()
函数。具体的转换方法取决于你想要的目标数据类型。
如何处理在转换过程中可能出现的错误?
在进行数据类型转换时,可能会遇到类型不匹配或值错误。例如,尝试将非数字字符串转换为整数时会引发ValueError
。为了避免这些问题,建议使用try...except
语句来捕获异常,并根据需求提供适当的错误处理,确保程序的稳定性。
在Python中,是否可以自定义转换函数?
是的,Python允许用户定义自己的转换函数。可以创建一个函数,根据特定的逻辑将输入的a类型数据转换为b类型数据。例如,你可以编写一个函数,将特定格式的字符串解析为字典。这种自定义函数的灵活性使得处理复杂数据转换变得更加容易和高效。
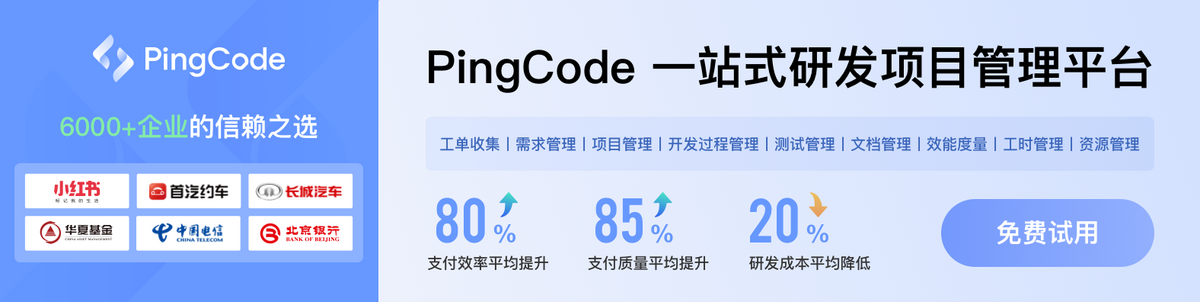