Python后端接受小程序传递文件的方法主要包括以下几个步骤:定义文件上传接口、解析和保存上传的文件、处理文件数据、返回处理结果。
在这篇文章中,我们将详细介绍如何实现这些步骤,并提供一些代码示例和实践建议。
一、定义文件上传接口
首先,我们需要定义一个接口来接收文件上传请求。可以使用Flask、Django或FastAPI等框架来实现这个接口。在这里,我们以Flask为例,展示如何定义一个文件上传接口。
使用Flask定义文件上传接口
Flask是一个轻量级的Python Web框架,非常适合快速开发和原型设计。我们可以使用Flask来定义一个简单的文件上传接口。
from flask import Flask, request, jsonify
import os
app = Flask(__name__)
UPLOAD_FOLDER = 'uploads'
os.makedirs(UPLOAD_FOLDER, exist_ok=True)
app.config['UPLOAD_FOLDER'] = UPLOAD_FOLDER
@app.route('/upload', methods=['POST'])
def upload_file():
if 'file' not in request.files:
return jsonify({"error": "No file part"}), 400
file = request.files['file']
if file.filename == '':
return jsonify({"error": "No selected file"}), 400
if file:
file_path = os.path.join(app.config['UPLOAD_FOLDER'], file.filename)
file.save(file_path)
return jsonify({"message": "File uploaded successfully", "file_path": file_path}), 200
if __name__ == '__main__':
app.run(debug=True)
在上面的代码中,我们定义了一个名为upload_file
的路由,它接收POST请求并处理文件上传。文件会被保存到指定的UPLOAD_FOLDER
目录中。
二、解析和保存上传的文件
在文件上传接口中,我们需要解析上传的文件并将其保存到服务器上。上面的代码示例已经展示了如何解析和保存文件。接下来,我们将进一步讨论如何处理不同类型的文件和一些常见的错误处理方法。
处理不同类型的文件
在实际应用中,我们可能需要处理不同类型的文件,例如图像、文档或音频文件。我们可以根据文件的MIME类型或文件扩展名来确定文件类型,并进行相应的处理。
def allowed_file(filename):
ALLOWED_EXTENSIONS = {'png', 'jpg', 'jpeg', 'gif', 'pdf', 'doc', 'docx'}
return '.' in filename and filename.rsplit('.', 1)[1].lower() in ALLOWED_EXTENSIONS
@app.route('/upload', methods=['POST'])
def upload_file():
if 'file' not in request.files:
return jsonify({"error": "No file part"}), 400
file = request.files['file']
if file.filename == '':
return jsonify({"error": "No selected file"}), 400
if file and allowed_file(file.filename):
file_path = os.path.join(app.config['UPLOAD_FOLDER'], file.filename)
file.save(file_path)
return jsonify({"message": "File uploaded successfully", "file_path": file_path}), 200
else:
return jsonify({"error": "File type not allowed"}), 400
在上面的代码中,我们定义了一个allowed_file
函数来检查文件扩展名是否在允许的范围内。如果文件类型不符合要求,我们将返回一个错误响应。
处理文件上传错误
在文件上传过程中,可能会遇到各种错误情况,例如文件过大、上传超时等。我们需要处理这些错误并返回适当的响应。
@app.route('/upload', methods=['POST'])
def upload_file():
try:
if 'file' not in request.files:
return jsonify({"error": "No file part"}), 400
file = request.files['file']
if file.filename == '':
return jsonify({"error": "No selected file"}), 400
if file and allowed_file(file.filename):
file_path = os.path.join(app.config['UPLOAD_FOLDER'], file.filename)
file.save(file_path)
return jsonify({"message": "File uploaded successfully", "file_path": file_path}), 200
else:
return jsonify({"error": "File type not allowed"}), 400
except Exception as e:
return jsonify({"error": str(e)}), 500
在上面的代码中,我们使用try-except
块来捕获异常,并返回一个包含错误信息的JSON响应。
三、处理文件数据
上传文件后,我们可能需要对文件数据进行处理,例如图像处理、文本解析或数据存储。根据具体需求,我们可以使用不同的库和工具来处理文件数据。
图像处理
如果上传的文件是图像,我们可以使用Pillow库来进行图像处理。例如,可以对图像进行缩放、裁剪或格式转换。
from PIL import Image
def process_image(file_path):
with Image.open(file_path) as img:
# Resize image
img = img.resize((800, 600))
# Save processed image
processed_file_path = file_path.replace('.', '_processed.')
img.save(processed_file_path)
return processed_file_path
@app.route('/upload', methods=['POST'])
def upload_file():
try:
if 'file' not in request.files:
return jsonify({"error": "No file part"}), 400
file = request.files['file']
if file.filename == '':
return jsonify({"error": "No selected file"}), 400
if file and allowed_file(file.filename):
file_path = os.path.join(app.config['UPLOAD_FOLDER'], file.filename)
file.save(file_path)
processed_file_path = process_image(file_path)
return jsonify({"message": "File uploaded and processed successfully", "file_path": processed_file_path}), 200
else:
return jsonify({"error": "File type not allowed"}), 400
except Exception as e:
return jsonify({"error": str(e)}), 500
在上面的代码中,我们定义了一个process_image
函数,用于对上传的图像进行处理。处理后的图像将被保存到一个新的文件中,并返回处理后的文件路径。
文本解析
如果上传的文件是文本或文档,我们可以使用不同的库来解析文件内容。例如,可以使用PyPDF2
库来解析PDF文件,或使用docx
库来解析Word文档。
import PyPDF2
def parse_pdf(file_path):
with open(file_path, 'rb') as file:
reader = PyPDF2.PdfFileReader(file)
text = ""
for page_num in range(reader.numPages):
page = reader.getPage(page_num)
text += page.extract_text()
return text
@app.route('/upload', methods=['POST'])
def upload_file():
try:
if 'file' not in request.files:
return jsonify({"error": "No file part"}), 400
file = request.files['file']
if file.filename == '':
return jsonify({"error": "No selected file"}), 400
if file and allowed_file(file.filename):
file_path = os.path.join(app.config['UPLOAD_FOLDER'], file.filename)
file.save(file_path)
if file.filename.endswith('.pdf'):
parsed_text = parse_pdf(file_path)
return jsonify({"message": "File uploaded and parsed successfully", "parsed_text": parsed_text}), 200
else:
return jsonify({"message": "File uploaded successfully", "file_path": file_path}), 200
else:
return jsonify({"error": "File type not allowed"}), 400
except Exception as e:
return jsonify({"error": str(e)}), 500
在上面的代码中,我们定义了一个parse_pdf
函数,用于解析上传的PDF文件,并将解析后的文本返回给客户端。
四、返回处理结果
在文件上传和处理完成后,我们需要返回处理结果给客户端。可以返回文件路径、处理后的数据或其他相关信息。返回结果时,建议使用JSON格式,以便客户端能够轻松解析。
返回文件路径
在简单的文件上传场景中,我们可以直接返回文件路径。
@app.route('/upload', methods=['POST'])
def upload_file():
try:
if 'file' not in request.files:
return jsonify({"error": "No file part"}), 400
file = request.files['file']
if file.filename == '':
return jsonify({"error": "No selected file"}), 400
if file and allowed_file(file.filename):
file_path = os.path.join(app.config['UPLOAD_FOLDER'], file.filename)
file.save(file_path)
return jsonify({"message": "File uploaded successfully", "file_path": file_path}), 200
else:
return jsonify({"error": "File type not allowed"}), 400
except Exception as e:
return jsonify({"error": str(e)}), 500
返回处理后的数据
在文件处理场景中,我们可以返回处理后的数据,例如图像处理后的新文件路径,或文本解析后的内容。
@app.route('/upload', methods=['POST'])
def upload_file():
try:
if 'file' not in request.files:
return jsonify({"error": "No file part"}), 400
file = request.files['file']
if file.filename == '':
return jsonify({"error": "No selected file"}), 400
if file and allowed_file(file.filename):
file_path = os.path.join(app.config['UPLOAD_FOLDER'], file.filename)
file.save(file_path)
if file.filename.endswith('.pdf'):
parsed_text = parse_pdf(file_path)
return jsonify({"message": "File uploaded and parsed successfully", "parsed_text": parsed_text}), 200
else:
processed_file_path = process_image(file_path)
return jsonify({"message": "File uploaded and processed successfully", "file_path": processed_file_path}), 200
else:
return jsonify({"error": "File type not allowed"}), 400
except Exception as e:
return jsonify({"error": str(e)}), 500
在上面的代码中,我们根据文件类型返回不同的处理结果。如果是PDF文件,返回解析后的文本;如果是图像文件,返回处理后的新文件路径。
总结
通过本文的介绍,我们了解了如何使用Python后端接受小程序传递文件,并对文件进行处理。主要步骤包括定义文件上传接口、解析和保存上传的文件、处理文件数据以及返回处理结果。我们还讨论了如何处理不同类型的文件和常见的错误情况。
希望这些内容能够帮助您更好地理解和实现Python后端文件上传功能。如果您有任何问题或建议,欢迎在评论区留言讨论。
相关问答FAQs:
如何在Python后端处理小程序上传的文件?
在Python后端处理小程序上传的文件通常涉及使用框架如Flask或Django。首先,需要设置一个路由来接收文件上传请求。通过request.files
,你可以获取上传的文件,然后可以选择将其保存到服务器的特定目录,或进行其他处理如数据分析或格式转换。
小程序上传文件时有哪些常见的格式要求?
小程序通常支持多种文件格式的上传,包括图片(如JPEG、PNG)、文档(如PDF、Word)等。在进行文件上传时,应确保在后端对接收到的文件格式进行检查,以确保符合预期的类型和大小限制。这不仅保护了服务器的安全性,还能提升用户体验。
如何提高小程序文件上传的安全性?
为了提高文件上传的安全性,可以采取几项措施。首先,限制可上传的文件类型,确保只允许特定格式的文件。同时,检查文件的大小,避免上传过大的文件导致服务器负担过重。此外,使用安全的存储方式,比如对上传的文件进行重命名,以避免文件覆盖或路径穿越攻击。最后,确保后端代码经过严格测试,以防止潜在的安全漏洞。
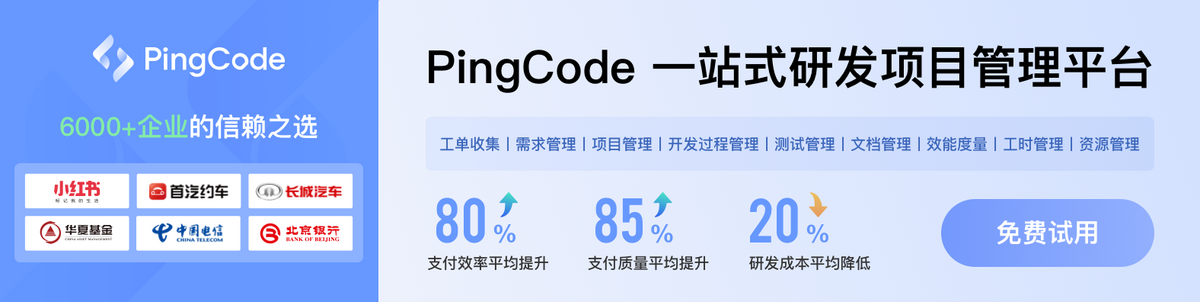