使用Python筛选字符串的方法包括使用字符串方法、列表解析、正则表达式等。 其中最常用的方式是利用字符串方法进行筛选。通过结合使用字符串的各种内置方法,您可以轻松实现对字符串的筛选。例如,通过字符串的find
方法可以查找子字符串的位置,通过startswith
和endswith
方法可以判断字符串是否以指定的前缀或后缀开头或结尾。接下来,我将详细介绍这些方法,并列举其他常用的筛选字符串的技术。
一、字符串方法筛选
Python 提供了许多内置方法,可以直接对字符串进行操作和筛选。这些方法简单易用,性能也较为优秀。
1、查找子字符串
使用find
方法可以查找字符串中子字符串的位置,如果子字符串存在于字符串中,则返回其第一次出现的索引;否则返回-1。
text = "Hello, welcome to the world of Python"
if text.find("welcome") != -1:
print("The substring 'welcome' is found in the text.")
else:
print("The substring 'welcome' is not found in the text.")
2、判断字符串开头和结尾
使用startswith
和endswith
方法,可以分别判断字符串是否以指定的前缀或后缀开头或结尾。
text = "example.py"
if text.startswith("ex"):
print("The text starts with 'ex'.")
if text.endswith(".py"):
print("The text ends with '.py'.")
3、检查字符是否在字符串中
使用in
关键字可以检查一个字符或子字符串是否在另一个字符串中。
text = "This is a sample text"
if "sample" in text:
print("The word 'sample' is in the text.")
else:
print("The word 'sample' is not in the text.")
二、列表解析
列表解析是一种简洁而高效的方式,用于从一个字符串列表中筛选出符合条件的字符串。列表解析可以使代码更加简洁明了。
1、筛选包含某个子字符串的字符串
texts = ["apple", "banana", "cherry", "date", "elderberry"]
filtered_texts = [text for text in texts if "a" in text]
print(filtered_texts) # Output: ['apple', 'banana', 'date']
2、筛选以特定前缀开头的字符串
texts = ["apple", "banana", "cherry", "date", "elderberry"]
filtered_texts = [text for text in texts if text.startswith("b")]
print(filtered_texts) # Output: ['banana']
3、筛选以特定后缀结尾的字符串
texts = ["file1.txt", "file2.py", "document.pdf", "image.png"]
filtered_texts = [text for text in texts if text.endswith(".py")]
print(filtered_texts) # Output: ['file2.py']
三、正则表达式
正则表达式是一个强大的字符串匹配工具,可以用于复杂的字符串筛选需求。Python 的re
模块提供了对正则表达式的支持。
1、匹配包含某个模式的字符串
import re
texts = ["apple", "banana", "cherry", "date", "elderberry"]
pattern = re.compile(r'a.*a')
filtered_texts = [text for text in texts if pattern.search(text)]
print(filtered_texts) # Output: ['banana']
2、匹配以特定模式开头的字符串
import re
texts = ["apple", "banana", "cherry", "date", "elderberry"]
pattern = re.compile(r'^a')
filtered_texts = [text for text in texts if pattern.match(text)]
print(filtered_texts) # Output: ['apple']
3、匹配以特定模式结尾的字符串
import re
texts = ["file1.txt", "file2.py", "document.pdf", "image.png"]
pattern = re.compile(r'\.py$')
filtered_texts = [text for text in texts if pattern.search(text)]
print(filtered_texts) # Output: ['file2.py']
四、结合使用多种方法
在实际应用中,常常需要结合使用多种筛选方法来满足复杂的需求。例如,我们可以先使用列表解析进行初步筛选,然后再使用正则表达式进行进一步的精细筛选。
示例:筛选既包含特定子字符串又以特定后缀结尾的字符串
import re
texts = ["apple.py", "banana.py", "cherry.txt", "date.py", "elderberry.py"]
pattern = re.compile(r'a.*\.py$')
filtered_texts = [text for text in texts if pattern.search(text)]
print(filtered_texts) # Output: ['banana.py']
通过结合不同的方法,我们可以实现更加灵活和精细的字符串筛选。
五、实践案例
1、筛选日志文件中的特定信息
在处理日志文件时,常常需要筛选出特定的日志条目。例如,筛选出所有包含错误信息的日志条目。
import re
log_lines = [
"[INFO] Server started",
"[ERROR] Failed to connect to database",
"[INFO] User login successful",
"[ERROR] Timeout while waiting for response",
]
error_pattern = re.compile(r'\[ERROR\]')
error_logs = [line for line in log_lines if error_pattern.search(line)]
print(error_logs) # Output: ['[ERROR] Failed to connect to database', '[ERROR] Timeout while waiting for response']
2、从文本中提取特定格式的数据
例如,从一段文本中提取所有的电子邮件地址。
import re
text = "Please contact us at support@example.com or sales@example.org for further information."
email_pattern = re.compile(r'[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+')
emails = email_pattern.findall(text)
print(emails) # Output: ['support@example.com', 'sales@example.org']
3、筛选包含特定关键词的新闻标题
在处理新闻数据时,常常需要筛选出包含特定关键词的新闻标题。
news_titles = [
"New Python release announced",
"Breaking news: Major earthquake in city",
"Python programming tips and tricks",
"Sports update: Local team wins championship",
]
keyword = "Python"
filtered_titles = [title for title in news_titles if keyword in title]
print(filtered_titles) # Output: ['New Python release announced', 'Python programming tips and tricks']
通过以上介绍,您可以看到,Python 提供了丰富的工具和方法来实现字符串的筛选。无论是简单的字符串方法、列表解析,还是强大的正则表达式,都可以帮助您高效地完成字符串筛选任务。掌握这些技巧,不仅能提高您的编程效率,还能让您在处理字符串数据时更加得心应手。
相关问答FAQs:
如何使用Python筛选特定条件的字符串?
在Python中,可以使用列表推导式结合条件语句来筛选字符串。例如,如果你想筛选出包含特定字母或长度的字符串,可以如下操作:
strings = ["apple", "banana", "cherry", "date"]
filtered = [s for s in strings if 'a' in s and len(s) > 5]
print(filtered) # 输出: ['banana']
这种方式高效且易于理解,适合处理较小的数据集。
是否可以使用正则表达式来筛选字符串?
正则表达式在字符串筛选中非常有用,尤其是当你需要根据复杂模式匹配时。Python的re
模块提供了丰富的功能来实现这一点。例如,以下代码展示了如何筛选出包含数字的字符串:
import re
strings = ["apple123", "banana", "cherry456", "date"]
filtered = [s for s in strings if re.search(r'\d', s)]
print(filtered) # 输出: ['apple123', 'cherry456']
这种方法灵活性高,适合处理复杂的匹配需求。
Python中还有哪些内置函数可以帮助筛选字符串?
Python提供了多种内置函数,可以简化字符串的筛选过程。filter()
函数结合str.contains()
方法可以用来筛选符合条件的字符串。例如,以下代码展示了如何筛选出以特定字母开头的字符串:
strings = ["apple", "banana", "cherry", "date"]
filtered = list(filter(lambda s: s.startswith('b'), strings))
print(filtered) # 输出: ['banana']
这种方法简洁明了,适合快速筛选。
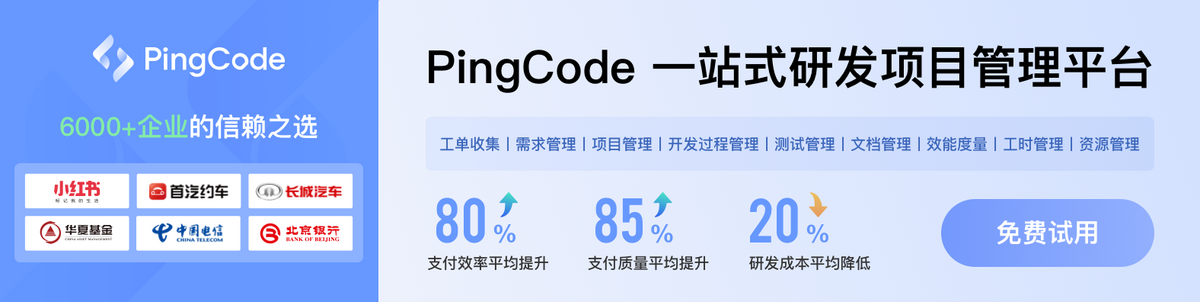