Python中执行Shell命令行的方法有多种,包括使用os.system()、subprocess模块、os.popen()等。推荐使用subprocess模块,因为它提供了更强大的功能和更好的错误处理机制。
使用subprocess模块
subprocess
模块是Python中最强大和推荐的执行Shell命令的方法。它提供了更多的功能和更好的错误处理机制。
基本用法
最基本的用法是使用subprocess.run()
函数:
import subprocess
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.stdout)
在上述代码中,subprocess.run()
函数执行了ls -l
命令,并捕获了其输出。capture_output=True
参数指示函数捕获标准输出和标准错误,text=True
参数指示函数返回字符串而不是字节。
捕获输出和错误
你可以使用subprocess.run()
函数的stderr
参数来捕获错误输出:
result = subprocess.run(['ls', '-l', '/nonexistent'], capture_output=True, text=True)
print("Standard Output:", result.stdout)
print("Standard Error:", result.stderr)
在这个例子中,尝试列出一个不存在的目录会产生错误信息,该信息会被捕获并打印出来。
处理返回码
subprocess.run()
函数返回一个CompletedProcess
对象,其中包含命令的返回码。你可以通过检查返回码来确定命令是否成功执行:
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
if result.returncode == 0:
print("Command succeeded")
else:
print("Command failed with return code", result.returncode)
使用os.system()
os.system()
是另一种执行Shell命令的方法,但它功能较弱且不推荐使用。它不能捕获输出,也没有错误处理机制:
import os
os.system('ls -l')
尽管os.system()
简单易用,但由于其局限性和安全问题(例如潜在的命令注入攻击),一般不推荐使用。
使用os.popen()
os.popen()
也是一种执行Shell命令的方法,它可以捕获命令的输出:
import os
stream = os.popen('ls -l')
output = stream.read()
print(output)
尽管os.popen()
可以捕获输出,但它已被subprocess
模块取代,后者提供了更强大的功能和更好的错误处理机制。
使用subprocess.Popen()
如果需要更复杂的交互,比如向子进程发送输入或实时读取输出,可以使用subprocess.Popen()
:
import subprocess
process = subprocess.Popen(['ls', '-l'], stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
stdout, stderr = process.communicate()
print("Standard Output:", stdout)
print("Standard Error:", stderr)
在这个例子中,subprocess.Popen()
创建了一个子进程,并通过communicate()
方法与其进行交互。
实时输出
有时你可能希望实时查看命令的输出,可以使用以下方法:
import subprocess
process = subprocess.Popen(['ping', 'google.com'], stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
while True:
output = process.stdout.readline()
if output == '' and process.poll() is not None:
break
if output:
print(output.strip())
这个例子展示了如何实时读取子进程的输出,如同在终端中运行命令一样。
安全性考虑
在执行Shell命令时,安全性是一个重要的考虑因素。特别是当你需要执行由用户输入的命令时,必须防止命令注入攻击。使用subprocess.run()
和传递参数列表,而不是拼接字符串,可以有效防止这种攻击:
import subprocess
user_input = 'file.txt'
result = subprocess.run(['ls', '-l', user_input], capture_output=True, text=True)
print(result.stdout)
在这个例子中,user_input
被安全地传递给subprocess.run()
,而不是直接拼接到命令字符串中。
总结
Python提供了多种方法来执行Shell命令,包括os.system()
、os.popen()
和subprocess
模块。推荐使用subprocess
模块,因为它功能强大,提供了更好的错误处理机制和安全性。无论是简单的命令执行还是复杂的子进程交互,subprocess
模块都能满足需求。通过合理使用这些工具,你可以在Python中高效、安全地执行Shell命令。
相关问答FAQs:
在Python中如何执行多个Shell命令?
可以使用subprocess
模块来执行多个Shell命令。通过subprocess.run()
或subprocess.Popen()
方法,您可以在Python脚本中串联多个命令。例如,您可以使用“&&”将命令连接在一起,这样在第一个命令成功执行后,第二个命令才会执行。确保将shell=True
参数传递给函数,以便在Shell环境中运行命令。
执行Shell命令后如何捕获输出?
使用subprocess.run()
时,可以通过设置capture_output=True
参数来捕获命令的标准输出和错误输出。这将返回一个CompletedProcess
对象,其中包含stdout
和stderr
属性,您可以在Python中进一步处理这些输出数据。
在执行Shell命令时如何处理错误?
在使用subprocess
模块执行Shell命令时,可以使用try
和except
块来捕获可能发生的异常。此外,您也可以检查returncode
属性,了解命令是否成功执行。若返回码不为0,则表示命令执行失败,您可以根据需要进行错误处理或输出详细错误信息。
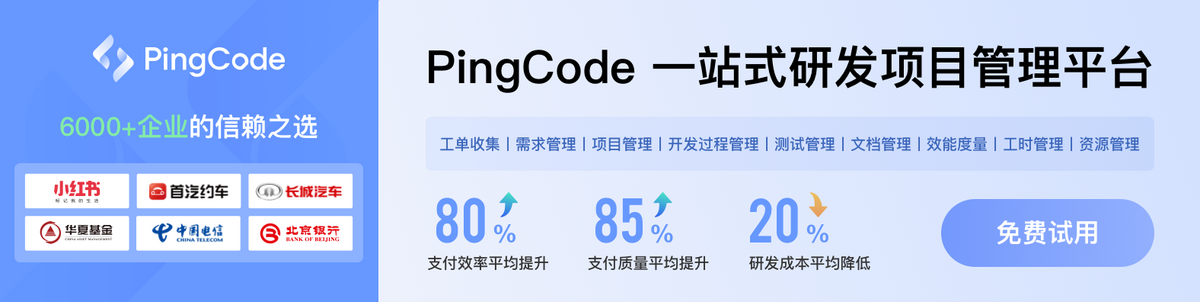