要用Python连接微信发消息,可以通过以下几种方法:使用itchat库、使用微信公众平台API、使用企业微信API。下面我们将详细介绍这几种方法中的一种,即使用itchat库进行操作。
itchat库是一个开源的微信个人号接口,可以方便地通过Python脚本实现微信消息的收发。以下是使用itchat库连接微信并发送消息的详细步骤:
一、安装itchat库
首先,我们需要安装itchat库。你可以使用以下命令通过pip安装:
pip install itchat
二、登录微信
使用itchat库登录微信需要扫描二维码进行验证,登录代码如下:
import itchat
登录微信
itchat.auto_login(hotReload=True)
保持登录状态
itchat.run()
hotReload=True
参数表示在本地保存登录状态,即使程序关闭一段时间也可以重新启动并保持登录状态,而无需再次扫码登录。
三、发送微信消息
登录成功后,我们可以发送微信消息。以下代码展示了如何给指定用户发送消息:
import itchat
登录微信
itchat.auto_login(hotReload=True)
发送消息
def send_message(to_user, message):
# 查找用户
users = itchat.search_friends(name=to_user)
if users:
user = users[0]
# 发送消息
itchat.send(message, toUserName=user['UserName'])
else:
print(f'User {to_user} not found!')
示例:发送消息给某个用户
send_message('FriendName', 'Hello, this is a test message!')
保持登录状态
itchat.run()
在上述代码中,我们定义了一个send_message
函数,该函数接受两个参数:to_user
表示接收消息的用户名称,message
表示要发送的消息内容。通过itchat.search_friends
函数找到用户,并通过itchat.send
函数发送消息。
四、发送群消息
除了发送消息给个人,我们还可以发送消息到微信群。以下代码展示了如何发送群消息:
import itchat
登录微信
itchat.auto_login(hotReload=True)
发送群消息
def send_group_message(group_name, message):
# 查找群聊
group = itchat.search_chatrooms(name=group_name)
if group:
group = group[0]
# 发送消息
itchat.send(message, toUserName=group['UserName'])
else:
print(f'Group {group_name} not found!')
示例:发送消息到某个群聊
send_group_message('GroupName', 'Hello, this is a test message to the group!')
保持登录状态
itchat.run()
在上述代码中,我们定义了一个send_group_message
函数,该函数接受两个参数:group_name
表示接收消息的群聊名称,message
表示要发送的消息内容。通过itchat.search_chatrooms
函数找到群聊,并通过itchat.send
函数发送消息。
五、附加功能
除了基本的发送消息功能,itchat库还提供了许多其他功能,例如接收消息、回复消息、获取好友列表等。以下是一些常用功能的示例代码:
1、接收消息
import itchat
登录微信
itchat.auto_login(hotReload=True)
接收消息的回调函数
@itchat.msg_register(itchat.content.TEXT)
def text_reply(msg):
print(f'Received message: {msg["Text"]}')
保持登录状态
itchat.run()
2、自动回复消息
import itchat
登录微信
itchat.auto_login(hotReload=True)
自动回复消息的回调函数
@itchat.msg_register(itchat.content.TEXT)
def auto_reply(msg):
reply = f'You said: {msg["Text"]}'
return reply
保持登录状态
itchat.run()
3、获取好友列表
import itchat
登录微信
itchat.auto_login(hotReload=True)
获取好友列表
friends = itchat.get_friends()
for friend in friends:
print(friend['NickName'])
保持登录状态
itchat.run()
六、注意事项
- itchat库是基于微信网页版的实现,微信网页版在某些地区或某些情况下可能无法使用。如果遇到无法登录的问题,可以尝试使用其他API或第三方库。
- 微信官方对使用自动化工具发送消息有一定限制,如果频繁发送消息可能会导致账号被封禁。在使用过程中请遵守相关规定,避免滥用。
- 使用itchat库发送消息时,需要确保微信客户端处于登录状态,否则消息发送会失败。
- 为了安全起见,建议在本地开发和测试,不要在公共服务器上运行代码,以免泄露个人微信信息。
通过以上步骤,我们可以使用Python通过itchat库实现连接微信并发送消息的功能。同时,itchat库还提供了丰富的扩展功能,可以根据实际需求进行灵活应用。
相关问答FAQs:
如何使用Python实现与微信的连接和消息发送?
要使用Python连接微信并发送消息,您可以使用一些现有的库,如itchat
。这个库提供了简单的API来发送和接收消息。您可以安装itchat
库,并使用相关函数登录您的微信账号,然后通过指定的用户或群组发送消息。
在Python中发送微信消息需要哪些步骤?
发送微信消息的主要步骤包括:安装itchat
库,使用itchat.auto_login()
登录您的微信账号,获取目标用户的ID(可以使用itchat.get_friends()
获取好友列表),最后使用itchat.send()
函数发送消息。确保在执行过程中保持微信的登录状态。
使用Python发送微信消息有哪些限制?
在使用Python发送微信消息时,需注意一些限制。例如,使用itchat
库发送消息时,可能受到微信平台的反骚扰机制限制,频繁发送同一内容可能会导致账号被封。此外,itchat
目前不支持发送文件或图片,您需要使用其他库来实现更复杂的功能。
如何确保发送的消息能够被接收者及时看到?
为了确保消息被及时看到,可以考虑在发送前确认接收者的在线状态,尽量选择在对方活跃的时间段发送消息。此外,发送的内容应该简明扼要,避免冗长的信息,这样更容易引起接收者的注意。
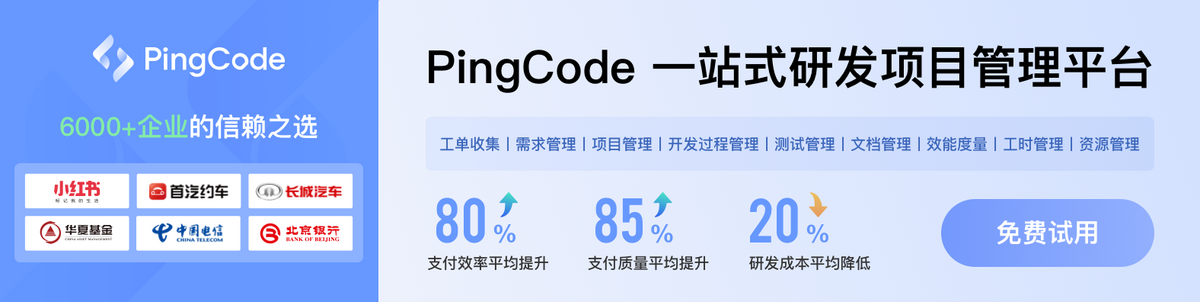