Python使用三角函数的步骤包括:导入math模块、使用内置三角函数方法、应用三角函数在实际问题中。本文将详细介绍如何在Python中使用三角函数,从基础的三角函数到高级应用,帮助您在编程中更好地理解和应用这些数学概念。
Python是一种功能强大的编程语言,它内置了丰富的数学函数库,其中包括各种三角函数,如正弦、余弦和正切函数。这些函数对于解决几何问题、信号处理、物理模拟等领域的问题非常有用。接下来,我们将详细介绍如何在Python中使用这些三角函数。
一、导入math模块
在Python中,三角函数被包含在math模块中。因此,在使用任何三角函数之前,我们需要先导入math模块。以下是导入math模块的基本方法:
import math
导入math模块后,我们就可以使用其中的各种三角函数和其他数学函数了。
二、使用内置三角函数方法
Python的math模块提供了多种三角函数,包括正弦函数(sin)、余弦函数(cos)、正切函数(tan)等。下面是一些常用三角函数的示例:
- 正弦函数(sin)
正弦函数用于计算一个角度的正弦值。输入的角度单位为弧度,返回值范围在[-1, 1]之间。
import math
计算30度的正弦值
angle_degrees = 30
angle_radians = math.radians(angle_degrees)
sin_value = math.sin(angle_radians)
print(f"Sin(30 degrees) = {sin_value}")
- 余弦函数(cos)
余弦函数用于计算一个角度的余弦值。输入的角度单位为弧度,返回值范围在[-1, 1]之间。
import math
计算60度的余弦值
angle_degrees = 60
angle_radians = math.radians(angle_degrees)
cos_value = math.cos(angle_radians)
print(f"Cos(60 degrees) = {cos_value}")
- 正切函数(tan)
正切函数用于计算一个角度的正切值。输入的角度单位为弧度,返回值范围在所有实数之间。
import math
计算45度的正切值
angle_degrees = 45
angle_radians = math.radians(angle_degrees)
tan_value = math.tan(angle_radians)
print(f"Tan(45 degrees) = {tan_value}")
三、角度与弧度转换
在使用三角函数时,经常需要在角度和弧度之间进行转换。Python的math模块提供了两个函数来进行这种转换:radians()
和degrees()
。
- 角度转换为弧度
import math
angle_degrees = 90
angle_radians = math.radians(angle_degrees)
print(f"{angle_degrees} degrees = {angle_radians} radians")
- 弧度转换为角度
import math
angle_radians = math.pi / 2
angle_degrees = math.degrees(angle_radians)
print(f"{angle_radians} radians = {angle_degrees} degrees")
四、反三角函数
反三角函数用于从三角函数值中计算角度。Python的math模块提供了三种反三角函数:反正弦函数(asin)、反余弦函数(acos)和反正切函数(atan)。
- 反正弦函数(asin)
反正弦函数返回一个值的反正弦,结果的范围在[-π/2, π/2]之间。
import math
sin_value = 0.5
angle_radians = math.asin(sin_value)
angle_degrees = math.degrees(angle_radians)
print(f"asin(0.5) = {angle_radians} radians = {angle_degrees} degrees")
- 反余弦函数(acos)
反余弦函数返回一个值的反余弦,结果的范围在[0, π]之间。
import math
cos_value = 0.5
angle_radians = math.acos(cos_value)
angle_degrees = math.degrees(angle_radians)
print(f"acos(0.5) = {angle_radians} radians = {angle_degrees} degrees")
- 反正切函数(atan)
反正切函数返回一个值的反正切,结果的范围在[-π/2, π/2]之间。
import math
tan_value = 1
angle_radians = math.atan(tan_value)
angle_degrees = math.degrees(angle_radians)
print(f"atan(1) = {angle_radians} radians = {angle_degrees} degrees")
五、三角函数的应用
三角函数在许多领域都有广泛的应用,包括物理、工程、计算机图形学等。以下是一些实际应用示例:
- 计算斜边长度
在直角三角形中,已知一个角度和一条直角边,可以使用三角函数计算斜边的长度。
import math
angle_degrees = 30
adjacent_length = 5
angle_radians = math.radians(angle_degrees)
hypotenuse_length = adjacent_length / math.cos(angle_radians)
print(f"斜边长度 = {hypotenuse_length}")
- 计算波形
三角函数广泛应用于波形计算,如正弦波和余弦波。在信号处理和音频处理中,正弦波和余弦波是基本的波形。
import math
import numpy as np
import matplotlib.pyplot as plt
生成正弦波
frequency = 5
sampling_rate = 1000
t = np.linspace(0, 1, sampling_rate)
amplitude = np.sin(2 * math.pi * frequency * t)
plt.plot(t, amplitude)
plt.title("Sine Wave")
plt.xlabel("Time (s)")
plt.ylabel("Amplitude")
plt.show()
- 计算旋转变换
在计算机图形学中,旋转变换是常见的操作。使用三角函数可以计算二维平面上点的旋转。
import math
def rotate_point(x, y, angle_degrees):
angle_radians = math.radians(angle_degrees)
x_new = x * math.cos(angle_radians) - y * math.sin(angle_radians)
y_new = x * math.sin(angle_radians) + y * math.cos(angle_radians)
return x_new, y_new
x, y = 1, 0
angle_degrees = 90
x_new, y_new = rotate_point(x, y, angle_degrees)
print(f"Rotated point: ({x_new}, {y_new})")
六、总结
通过本文的介绍,我们详细了解了如何在Python中使用三角函数,包括导入math模块、使用内置三角函数方法、角度与弧度转换、反三角函数以及三角函数的实际应用。三角函数在编程中的应用非常广泛,掌握这些函数的使用方法将帮助我们解决许多实际问题。希望本文对您理解和应用Python中的三角函数有所帮助。
相关问答FAQs:
如何在Python中导入三角函数库?
在Python中,可以通过导入math
模块来使用三角函数。只需在代码的开头添加import math
,就可以使用如math.sin()
、math.cos()
和math.tan()
等函数来计算三角函数值。此外,如果需要处理角度,可以使用math.radians()
将角度转换为弧度。
Python中的三角函数返回值是什么?
Python中的三角函数返回值通常是浮点数,表示函数的计算结果。比如,math.sin()
返回给定角度的正弦值,范围在-1到1之间。对于math.acos()
等反三角函数,它们的返回值范围也有明确的限制,比如math.acos()
的返回值在0到π之间。
如何处理角度和弧度之间的转换?
在Python中,三角函数的输入通常是以弧度为单位的。如果你有角度值,想要在三角函数中使用,可以使用math.radians()
将角度转换为弧度。例如,radians = math.radians(30)
将30度转换为弧度,随后可以传入math.sin(radians)
进行计算。反之,使用math.degrees()
可以将弧度转换回角度。
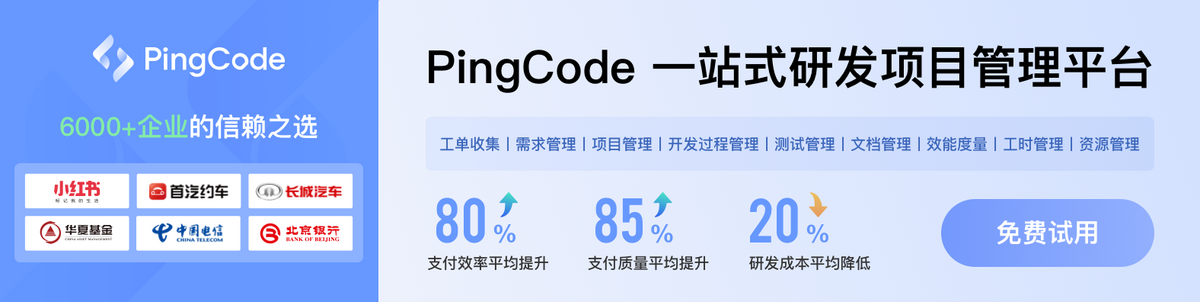