使用Python制作渐变背景色的方法包括:使用图像处理库(如PIL)、使用可视化库(如Matplotlib)和使用GUI库(如Tkinter)。下面将重点介绍使用PIL库来创建渐变背景色的方法。
使用PIL(Python Imaging Library)的Image和ImageDraw模块,您可以轻松创建渐变背景。这个方法的核心是遍历图像的每一个像素,根据其位置计算颜色值并填充。具体操作步骤如下:
- 导入PIL库并创建一个新图像。
- 定义渐变的颜色范围。
- 遍历图像像素并根据位置计算颜色值。
- 保存并显示生成的图像。
一、导入PIL库并创建新图像
首先,您需要安装PIL库(现为Pillow),然后导入相关模块,并创建一个新的空白图像。
from PIL import Image, ImageDraw
图像尺寸
width, height = 800, 600
创建一个新的空白图像
image = Image.new('RGB', (width, height))
二、定义渐变的颜色范围
为了创建渐变效果,我们需要定义起始颜色和结束颜色。假设我们希望从蓝色渐变到绿色。
# 定义渐变的起始颜色和结束颜色
start_color = (0, 0, 255) # 蓝色
end_color = (0, 255, 0) # 绿色
三、遍历图像像素并根据位置计算颜色值
通过遍历图像的每一个像素位置,我们可以根据其位置计算出相应的颜色值。这里假设我们创建的是从上到下的垂直渐变。
# 获取ImageDraw对象
draw = ImageDraw.Draw(image)
for y in range(height):
# 计算当前行的颜色
r = int(start_color[0] + (end_color[0] - start_color[0]) * (y / height))
g = int(start_color[1] + (end_color[1] - start_color[1]) * (y / height))
b = int(start_color[2] + (end_color[2] - start_color[2]) * (y / height))
color = (r, g, b)
# 画一条水平线
draw.line([(0, y), (width, y)], fill=color)
四、保存并显示生成的图像
最后,将生成的图像保存到文件并显示出来。
# 保存图像
image.save('gradient_background.png')
显示图像
image.show()
更多渐变效果
使用上述方法,您还可以创建其他方向和样式的渐变背景。例如:
- 水平渐变:遍历图像的每一列而不是每一行。
- 对角渐变:同时遍历行和列,根据两者的比例计算颜色。
- 多重渐变:通过设置多个颜色节点,实现复杂的渐变效果。
水平渐变示例
for x in range(width):
# 计算当前列的颜色
r = int(start_color[0] + (end_color[0] - start_color[0]) * (x / width))
g = int(start_color[1] + (end_color[1] - start_color[1]) * (x / width))
b = int(start_color[2] + (end_color[2] - start_color[2]) * (x / width))
color = (r, g, b)
# 画一条垂直线
draw.line([(x, 0), (x, height)], fill=color)
多重渐变示例
# 定义多个颜色节点
colors = [(255, 0, 0), (255, 255, 0), (0, 255, 0), (0, 255, 255), (0, 0, 255)]
num_colors = len(colors)
for y in range(height):
# 当前行所处的颜色段
segment = y / (height / (num_colors - 1))
index = int(segment)
if index >= num_colors - 1:
index = num_colors - 2
start_color = colors[index]
end_color = colors[index + 1]
# 计算当前行的颜色
ratio = segment - index
r = int(start_color[0] + (end_color[0] - start_color[0]) * ratio)
g = int(start_color[1] + (end_color[1] - start_color[1]) * ratio)
b = int(start_color[2] + (end_color[2] - start_color[2]) * ratio)
color = (r, g, b)
# 画一条水平线
draw.line([(0, y), (width, y)], fill=color)
使用其他库实现渐变效果
除了PIL,您还可以使用其他库来实现渐变背景效果。例如:
- Matplotlib:用于数据可视化,也可以生成渐变背景。
- Tkinter:用于GUI应用开发,可以创建渐变背景的窗口。
使用Matplotlib生成渐变背景
import matplotlib.pyplot as plt
import numpy as np
图像尺寸
width, height = 800, 600
创建渐变背景数据
gradient = np.linspace(0, 1, height)
gradient = np.vstack((gradient, gradient))
显示渐变背景
fig, ax = plt.subplots(figsize=(width/100, height/100))
ax.imshow(gradient, aspect='auto', cmap=plt.get_cmap('Blues'))
ax.axis('off') # 关闭坐标轴
plt.savefig('gradient_background_matplotlib.png', bbox_inches='tight', pad_inches=0)
plt.show()
使用Tkinter创建渐变背景
import tkinter as tk
def create_gradient(canvas, width, height, start_color, end_color):
for i in range(height):
ratio = i / height
r = int(start_color[0] + (end_color[0] - start_color[0]) * ratio)
g = int(start_color[1] + (end_color[1] - start_color[1]) * ratio)
b = int(start_color[2] + (end_color[2] - start_color[2]) * ratio)
color = f'#{r:02x}{g:02x}{b:02x}'
canvas.create_line(0, i, width, i, fill=color)
创建Tkinter窗口
root = tk.Tk()
width, height = 800, 600
canvas = tk.Canvas(root, width=width, height=height)
canvas.pack()
定义渐变颜色
start_color = (0, 0, 255)
end_color = (0, 255, 0)
创建渐变背景
create_gradient(canvas, width, height, start_color, end_color)
运行Tkinter主循环
root.mainloop()
通过以上方法,您可以在Python中使用不同的库创建各种样式的渐变背景。每种方法都有其独特的优势和适用场景,选择适合您的方法可以更好地实现所需的效果。
相关问答FAQs:
如何在Python中使用渐变背景色的库?
在Python中,使用渐变背景色可以通过多种图形库实现。常用的库包括Pygame、Tkinter和Matplotlib等。Pygame可以用于创建游戏界面,Tkinter适合于桌面应用程序,而Matplotlib则可以用于数据可视化。在这些库中,你可以通过设置颜色渐变的算法,动态生成背景色。
实现渐变背景色的基本步骤是什么?
实现渐变背景色通常需要定义起始和结束颜色,然后计算中间的颜色值。具体步骤包括:
- 确定起始颜色和结束颜色的RGB值。
- 根据需要的渐变步数,计算每一步的颜色变化量。
- 在绘制背景时,根据计算出的颜色值逐步填充背景。
渐变背景色在用户界面设计中的应用有哪些?
渐变背景色在用户界面设计中应用广泛。它可以增强视觉吸引力,提升用户体验,创造深度和层次感。比如,渐变可以用于网页背景、按钮、卡片或其他界面元素,帮助引导用户注意力。同时,合理的渐变色彩组合还能传达品牌形象和情感,增加整体设计的专业感和美观度。
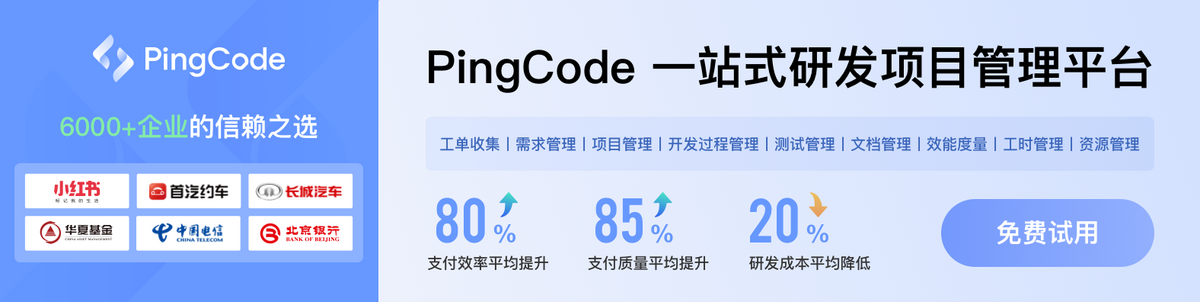