在Python中,可以使用文件流在函数中进行文件操作。要在函数中使用文件流,可以通过以下几个步骤来实现:打开文件、读取或写入文件、关闭文件。其中,常用的方法包括使用open()
函数打开文件、使用read()
或write()
函数进行文件操作、使用close()
函数关闭文件。以下是一个详细的示例,展示了如何在函数里使用文件流:
首先,我们需要了解文件操作的基本步骤:
- 打开文件:使用
open()
函数打开文件,并指定文件的模式(读取、写入、追加等)。 - 读取或写入文件:使用文件对象的
read()
、write()
等方法进行文件操作。 - 关闭文件:使用文件对象的
close()
方法关闭文件,确保资源被释放。
在详细描述文件流操作之前,让我们先简单介绍一下文件模式:
'r'
:读取模式(默认模式)。如果文件不存在,会引发FileNotFoundError
。'w'
:写入模式。如果文件已存在,会覆盖文件。如果文件不存在,会创建新文件。'a'
:追加模式。如果文件已存在,会在文件末尾追加内容。如果文件不存在,会创建新文件。'b'
:二进制模式。可以与其他模式组合使用,如'rb'
、'wb'
、'ab'
。'+'
:读写模式。可以与其他模式组合使用,如'r+'
、'w+'
、'a+'
。
一、打开文件
在Python中,使用open()
函数可以打开文件。它返回一个文件对象,提供了一些方法和属性供我们操作文件。open()
函数的基本语法如下:
file_object = open(file_path, mode)
file_path
:文件路径(可以是相对路径或绝对路径)。mode
:文件模式,指定如何打开文件(例如读取、写入等)。
二、读取文件
在函数中读取文件的示例:
def read_file(file_path):
try:
with open(file_path, 'r') as file:
content = file.read()
return content
except FileNotFoundError:
return "File not found."
示例调用
file_content = read_file('example.txt')
print(file_content)
在这个示例中,函数read_file
接受文件路径作为参数,使用open()
函数以读取模式打开文件,并使用with
语句确保文件在操作结束后自动关闭。文件内容通过read()
方法读取并返回。
三、写入文件
在函数中写入文件的示例:
def write_file(file_path, content):
with open(file_path, 'w') as file:
file.write(content)
示例调用
write_file('example.txt', 'Hello, world!')
在这个示例中,函数write_file
接受文件路径和要写入的内容作为参数,使用open()
函数以写入模式打开文件,并使用write()
方法将内容写入文件。
四、追加内容到文件
在函数中追加内容到文件的示例:
def append_to_file(file_path, content):
with open(file_path, 'a') as file:
file.write(content)
示例调用
append_to_file('example.txt', '\nAppended text.')
在这个示例中,函数append_to_file
接受文件路径和要追加的内容作为参数,使用open()
函数以追加模式打开文件,并使用write()
方法将内容追加到文件末尾。
五、使用二进制模式操作文件
在函数中使用二进制模式读取文件的示例:
def read_binary_file(file_path):
with open(file_path, 'rb') as file:
content = file.read()
return content
示例调用
binary_content = read_binary_file('example.bin')
print(binary_content)
在这个示例中,函数read_binary_file
接受文件路径作为参数,使用open()
函数以二进制读取模式打开文件,并使用read()
方法读取文件内容。
六、文件流操作的错误处理
在文件操作过程中,可能会遇到一些常见的错误,如文件不存在、权限不足等。为了提高代码的鲁棒性,可以使用try-except
语句进行错误处理。以下是一个示例:
def safe_read_file(file_path):
try:
with open(file_path, 'r') as file:
content = file.read()
return content
except FileNotFoundError:
return "File not found."
except PermissionError:
return "Permission denied."
except Exception as e:
return f"An unexpected error occurred: {e}"
示例调用
file_content = safe_read_file('example.txt')
print(file_content)
在这个示例中,函数safe_read_file
使用try-except
语句捕获并处理常见的文件操作错误,如文件不存在和权限不足。
七、使用上下文管理器
在Python中,使用上下文管理器(with
语句)进行文件操作是一个好习惯。上下文管理器可以确保文件在操作结束后自动关闭,即使在操作过程中发生异常。以下是一个示例:
def read_file_with_context_manager(file_path):
with open(file_path, 'r') as file:
content = file.read()
return content
示例调用
file_content = read_file_with_context_manager('example.txt')
print(file_content)
在这个示例中,函数read_file_with_context_manager
使用with
语句打开文件,并在操作结束后自动关闭文件。
八、示例:在函数中使用文件流处理数据
以下是一个更复杂的示例,展示如何在函数中使用文件流读取数据、处理数据并将结果写入新文件:
def process_file(input_file_path, output_file_path):
try:
with open(input_file_path, 'r') as infile:
content = infile.readlines()
processed_content = [line.strip().upper() for line in content]
with open(output_file_path, 'w') as outfile:
for line in processed_content:
outfile.write(line + '\n')
return "File processed successfully."
except FileNotFoundError:
return "Input file not found."
except PermissionError:
return "Permission denied."
except Exception as e:
return f"An unexpected error occurred: {e}"
示例调用
result = process_file('input.txt', 'output.txt')
print(result)
在这个示例中,函数process_file
接受输入文件路径和输出文件路径作为参数,使用文件流读取输入文件的内容,对每行内容进行处理(去除空白字符并转换为大写),然后将处理后的内容写入输出文件。
通过以上示例,我们可以看出,在Python中使用文件流在函数中进行文件操作是非常方便和灵活的。无论是读取文件、写入文件还是追加内容,都可以通过open()
函数和文件对象的方法轻松实现。同时,使用上下文管理器和错误处理机制可以提高代码的鲁棒性,确保文件操作的安全性和可靠性。
相关问答FAQs:
如何在Python函数中打开和读取文件流?
在Python中,可以使用内置的open()
函数在函数内部打开文件。通过指定文件路径和模式(如读取、写入等),可以获得一个文件对象。使用该文件对象的read()
、readline()
或readlines()
等方法,可以读取文件内容。例如:
def read_file(file_path):
with open(file_path, 'r') as file:
content = file.read()
return content
这种方式确保文件在使用后自动关闭,避免资源泄露。
在函数中写入文件流的最佳实践是什么?
在函数中写入文件流时,建议使用with
语句来管理文件操作,它可以确保文件在完成写入后自动关闭。写入模式可以是'w'
(写入)或'a'
(追加)。例如:
def write_to_file(file_path, data):
with open(file_path, 'a') as file:
file.write(data + '\n')
这种方法简洁明了,并能有效防止因忘记关闭文件而造成的潜在问题。
如何在Python函数中处理文件流异常?
在操作文件流时,可能会遇到各种异常,例如文件不存在或权限问题。使用try...except
语句可以捕获这些异常并进行适当处理。例如:
def safe_read_file(file_path):
try:
with open(file_path, 'r') as file:
return file.read()
except FileNotFoundError:
return "文件未找到,请检查路径。"
except IOError:
return "读取文件时发生错误。"
这种方式可以提高程序的健壮性,确保用户能够获得清晰的错误提示。
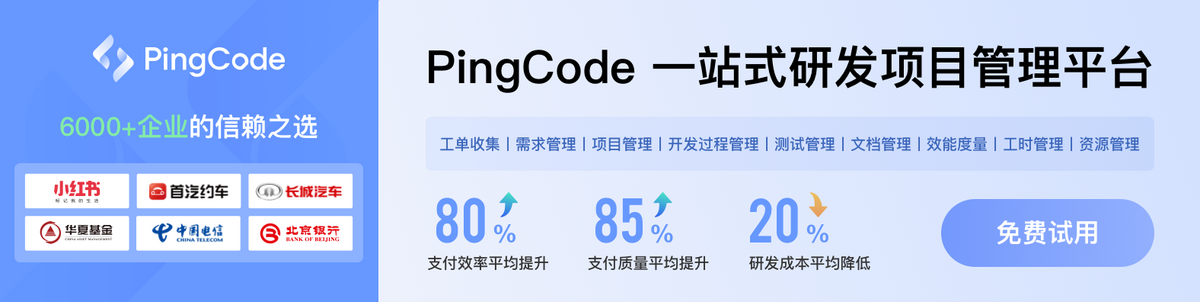