在Python中,可以通过多种方式将两个元组引入函数中:解包、合并元组、使用默认参数等。 其中,最常用和简便的方法是使用解包操作。解包操作可以在函数定义时通过*args
参数来实现,它能够接收任意数量的参数,并在函数内部将这些参数作为元组处理。接下来,我们将详细介绍这些方法并通过示例来演示它们的使用。
一、使用解包操作
解包操作是引入多个元组到函数中的最常用方法。我们可以使用*args
来接收任意数量的参数,然后在函数内部将这些参数作为一个元组处理。
示例:
def process_tuples(*args):
for tuple_ in args:
print(tuple_)
tuple1 = (1, 2, 3)
tuple2 = (4, 5, 6)
process_tuples(tuple1, tuple2)
在上述示例中,函数process_tuples
使用了*args
来接收任意数量的参数,并在内部将这些参数作为元组进行处理。
二、合并元组
另一种方法是将两个元组在调用函数之前进行合并,然后将合并后的元组作为参数传递给函数。
示例:
def process_combined_tuple(combined_tuple):
for item in combined_tuple:
print(item)
tuple1 = (1, 2, 3)
tuple2 = (4, 5, 6)
combined_tuple = tuple1 + tuple2
process_combined_tuple(combined_tuple)
在上述示例中,我们首先将tuple1
和tuple2
合并为combined_tuple
,然后将合并后的元组传递给函数process_combined_tuple
。
三、使用默认参数
我们还可以使用默认参数来引入两个元组。这样可以在函数定义时指定默认的元组值,并在调用函数时传递不同的元组。
示例:
def process_with_defaults(tuple1=(1, 2, 3), tuple2=(4, 5, 6)):
print("Tuple 1:", tuple1)
print("Tuple 2:", tuple2)
tuple_a = (7, 8, 9)
tuple_b = (10, 11, 12)
process_with_defaults(tuple_a, tuple_b)
在上述示例中,函数process_with_defaults
定义了两个默认的元组参数tuple1
和tuple2
。在调用函数时,我们可以传递不同的元组来覆盖默认值。
四、使用关键字参数
关键字参数也可以用于引入多个元组到函数中。通过使用关键字参数,我们可以在调用函数时显式地指定每个元组的参数名称。
示例:
def process_with_kwargs(kwargs):
for key, value in kwargs.items():
print(f"{key}: {value}")
tuple1 = (1, 2, 3)
tuple2 = (4, 5, 6)
process_with_kwargs(tuple1=tuple1, tuple2=tuple2)
在上述示例中,函数process_with_kwargs
使用了kwargs
来接收关键字参数,并在函数内部处理这些参数。
五、使用列表或字典
最后,我们还可以通过将元组放入列表或字典中,然后将列表或字典作为参数传递给函数。这种方法可以在函数内部更灵活地处理多个元组。
示例:
def process_with_list(tuples_list):
for tuple_ in tuples_list:
print(tuple_)
tuple1 = (1, 2, 3)
tuple2 = (4, 5, 6)
tuples_list = [tuple1, tuple2]
process_with_list(tuples_list)
在上述示例中,我们将tuple1
和tuple2
放入列表tuples_list
中,然后将列表传递给函数process_with_list
。
def process_with_dict(tuples_dict):
for key, value in tuples_dict.items():
print(f"{key}: {value}")
tuple1 = (1, 2, 3)
tuple2 = (4, 5, 6)
tuples_dict = {'tuple1': tuple1, 'tuple2': tuple2}
process_with_dict(tuples_dict)
在上述示例中,我们将tuple1
和tuple2
放入字典tuples_dict
中,然后将字典传递给函数process_with_dict
。
结论
在Python中,引入两个元组到函数中有多种方法,包括解包操作、合并元组、使用默认参数、关键字参数以及使用列表或字典。每种方法都有其独特的优势和适用场景。在实际开发中,可以根据具体需求选择最合适的方法来实现功能。通过合理地使用这些方法,可以使代码更加简洁、灵活和易于维护。
相关问答FAQs:
如何在Python函数中传递多个元组?
在Python中,可以通过在函数定义时将元组作为参数传递来引入多个元组。例如,可以定义一个函数,接收两个元组作为参数,然后在函数内部对它们进行操作。示例如下:
def process_tuples(tuple1, tuple2):
# 示例操作:合并两个元组
combined = tuple1 + tuple2
return combined
result = process_tuples((1, 2, 3), (4, 5, 6))
print(result) # 输出: (1, 2, 3, 4, 5, 6)
在函数中如何解包多个元组?
解包元组可以简化对元组内部元素的访问。在函数内部,可以通过参数解包的方式直接获取元组的各个元素。例如:
def unpack_tuples(tuple1, tuple2):
a, b, c = tuple1
x, y, z = tuple2
return a + x, b + y, c + z
result = unpack_tuples((1, 2, 3), (4, 5, 6))
print(result) # 输出: (5, 7, 9)
如何在函数中处理任意数量的元组?
如果希望在函数中接受任意数量的元组,可以使用可变参数。在函数定义中使用星号(*)来接收多个元组。示例如下:
def handle_multiple_tuples(*tuples):
for index, t in enumerate(tuples):
print(f"Tuple {index + 1}: {t}")
handle_multiple_tuples((1, 2), (3, 4), (5, 6))
以上例子展示了如何灵活地处理多个元组,使得函数的使用更加多样化。
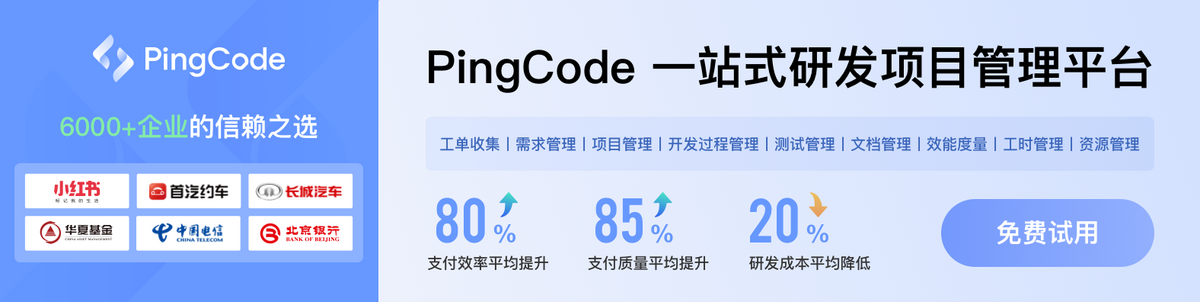