在Python中,输出字符串的方法有很多,主要包括使用print()函数、格式化字符串输出、使用f-string等。print()函数最为常用,可以直接输出字符串。
在Python中,输出字符串是一个基础但非常重要的操作,尤其是在编写程序时,需要经常与用户进行交互或者调试代码。常用的方式是通过print()
函数输出字符串。下面将详细介绍几种输出字符串的方法及其应用。
一、PRINT()函数
print()
函数是Python中最常用的输出方式。它可以将任何类型的数据输出到控制台,包括字符串、数字、列表等。使用print()
函数输出字符串非常简单,只需要将字符串传递给函数即可。
print("Hello, World!")
这段代码会输出字符串 "Hello, World!" 到控制台。
多个参数输出
print()
函数可以接受多个参数,并将它们依次输出,参数之间会用空格分隔。
print("Hello", "World", "from", "Python")
输出结果为:Hello World from Python
使用sep参数
print()
函数提供了sep
参数,可以自定义参数之间的分隔符。
print("Hello", "World", "from", "Python", sep="-")
输出结果为:Hello-World-from-Python
使用end参数
print()
函数的end
参数用于指定输出结束后的字符,默认为换行符\n
。
print("Hello, World!", end=" ")
print("Welcome to Python.")
输出结果为:Hello, World! Welcome to Python.
二、使用格式化字符串输出
在Python中,可以使用%
操作符、str.format()
方法和f-string
来格式化输出字符串。
使用%操作符
%
操作符可以用来格式化字符串,通过将变量插入到字符串中。
name = "Alice"
age = 30
print("Name: %s, Age: %d" % (name, age))
输出结果为:Name: Alice, Age: 30
使用str.format()方法
str.format()
方法是Python 3中推荐的字符串格式化方法,它功能更加强大和灵活。
name = "Alice"
age = 30
print("Name: {}, Age: {}".format(name, age))
输出结果为:Name: Alice, Age: 30
使用f-string
f-string是Python 3.6引入的一种新的字符串格式化方法,使用起来更加简洁和高效。
name = "Alice"
age = 30
print(f"Name: {name}, Age: {age}")
输出结果为:Name: Alice, Age: 30
三、字符串拼接输出
除了使用print()
函数和格式化字符串外,还可以通过字符串拼接的方式输出字符串。
使用+操作符
使用+
操作符可以将多个字符串拼接成一个新的字符串。
str1 = "Hello"
str2 = "World"
result = str1 + " " + str2
print(result)
输出结果为:Hello World
使用join()方法
join()
方法可以将一个可迭代对象(如列表、元组)中的所有元素拼接成一个字符串。
words = ["Hello", "World", "from", "Python"]
result = " ".join(words)
print(result)
输出结果为:Hello World from Python
四、输出字符串的特殊字符
在输出字符串时,有时需要包含一些特殊字符,如换行符、制表符等。可以使用转义字符来实现。
换行符
换行符\n
用于在输出字符串时换行。
print("Hello\nWorld")
输出结果为:
Hello
World
制表符
制表符\t
用于在输出字符串时添加水平制表符。
print("Hello\tWorld")
输出结果为:Hello World
反斜杠
反斜杠\
用于在输出字符串时包含一个反斜杠。
print("This is a backslash: \\")
输出结果为:This is a backslash:
五、输出包含引号的字符串
有时需要输出包含引号的字符串,可以使用转义字符或不同类型的引号来实现。
使用转义字符
print("He said, \"Hello, World!\"")
输出结果为:He said, "Hello, World!"
使用不同类型的引号
可以使用单引号或双引号来包含字符串,从而避免转义字符。
print('He said, "Hello, World!"')
print("It's a beautiful day!")
输出结果为:
He said, "Hello, World!"
It's a beautiful day!
六、输出多行字符串
Python支持使用三重引号('''
或 """
)来输出多行字符串。
print("""This is a
multi-line
string.""")
输出结果为:
This is a
multi-line
string.
七、使用变量和表达式
在输出字符串时,可以包含变量和表达式,使用不同的方法来实现。
使用print()函数
name = "Alice"
age = 30
print("Name:", name, "Age:", age)
输出结果为:Name: Alice Age: 30
使用格式化字符串
name = "Alice"
age = 30
print(f"Name: {name}, Age: {age}")
输出结果为:Name: Alice, Age: 30
使用表达式
x = 10
y = 20
print(f"The sum of {x} and {y} is {x + y}")
输出结果为:The sum of 10 and 20 is 30
八、输出字符串到文件
除了输出字符串到控制台,还可以将字符串输出到文件中。
使用write()方法
可以使用文件对象的write()
方法将字符串写入文件。
with open("output.txt", "w") as file:
file.write("Hello, World!")
使用print()函数
print()
函数也可以将输出重定向到文件。
with open("output.txt", "w") as file:
print("Hello, World!", file=file)
总结
通过以上介绍,我们详细讨论了Python中输出字符串的各种方法,包括print()
函数、格式化字符串输出、字符串拼接、特殊字符、多行字符串、变量和表达式、以及输出到文件等。掌握这些方法可以帮助我们在编写Python程序时,更加灵活和高效地输出字符串。希望这些内容对你有所帮助。
相关问答FAQs:
如何在Python中打印字符串?
在Python中,可以使用print()
函数来输出字符串。只需将字符串作为参数传递给该函数,例如:print("Hello, World!")
,这将会在控制台上显示“Hello, World!”。
Python中是否支持多种字符串格式化输出?
是的,Python支持多种字符串格式化方法,包括f-string、format()
方法和百分号格式化。f-string是Python 3.6及以上版本的特性,通过在字符串前加f
,并用花括号包裹变量,可以轻松实现格式化。例如:name = "Alice"; print(f"Hello, {name}!")
会输出“Hello, Alice!”。
如何在Python中输出包含变量的字符串?
输出包含变量的字符串可以通过字符串拼接、f-string或者format()
方法实现。例如,使用f-string的方式:age = 30; print(f"I am {age} years old.")
将输出“I am 30 years old.”。这种方式不仅简洁,而且易于阅读。
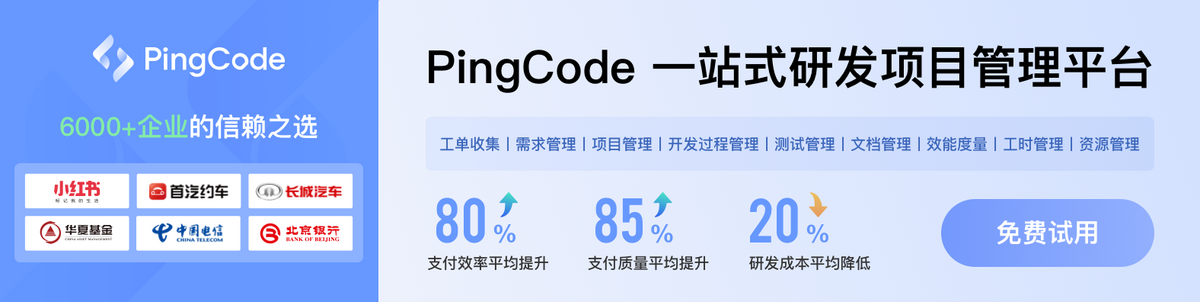