Python的time模块使用方法
Python的time模块提供了多种用于操作时间和日期的函数。它主要用于测量时间的流逝、获取当前时间、格式化时间、进行延时等操作。常用的函数包括time()、sleep()、strftime()、localtime()、gmtime()、mktime()等。下面我们将详细介绍这些函数的使用方法及其应用。
一、获取当前时间
time()
函数
time()
函数返回当前时间的时间戳(即从1970年1月1日00:00:00 UTC开始到现在的秒数)。这是一个浮点数,表示自纪元以来的秒数,适用于高精度时间测量。
import time
current_time = time.time()
print(f"当前时间的时间戳为: {current_time}")
localtime()
和gmtime()
函数
localtime()
函数将时间戳转换为当前本地时间的struct_time对象。gmtime()
函数将时间戳转换为UTC时间的struct_time对象。
import time
current_time = time.time()
转换为本地时间
local_time = time.localtime(current_time)
print(f"本地时间为: {local_time}")
转换为UTC时间
utc_time = time.gmtime(current_time)
print(f"UTC时间为: {utc_time}")
二、格式化时间
strftime()
函数
strftime()
函数根据指定的格式,将struct_time对象转换为字符串。常用的格式符号有:%Y(年份)、%m(月)、%d(日)、%H(小时)、%M(分钟)、%S(秒)等。
import time
current_time = time.localtime()
formatted_time = time.strftime("%Y-%m-%d %H:%M:%S", current_time)
print(f"格式化后的时间为: {formatted_time}")
strptime()
函数
strptime()
函数将字符串解析为struct_time对象。需要提供时间字符串和相应的格式。
import time
time_string = "2023-10-01 12:00:00"
parsed_time = time.strptime(time_string, "%Y-%m-%d %H:%M:%S")
print(f"解析后的时间为: {parsed_time}")
三、时间延时
sleep()
函数
sleep()
函数使程序暂停执行指定的秒数。适用于需要延时操作的场景。
import time
print("延时开始")
time.sleep(5) # 暂停5秒
print("延时结束")
四、测量时间间隔
time()
函数
通过记录两个时间点的时间戳,可以测量代码或函数执行的时间间隔。
import time
start_time = time.time()
要测量的代码
time.sleep(3)
end_time = time.time()
elapsed_time = end_time - start_time
print(f"代码执行时间为: {elapsed_time} 秒")
五、转换时间
mktime()
函数
mktime()
函数将struct_time对象转换为时间戳。
import time
current_time = time.localtime()
time_stamp = time.mktime(current_time)
print(f"时间戳为: {time_stamp}")
asctime()
和ctime()
函数
asctime()
函数将struct_time对象转换为字符串。ctime()
函数将时间戳转换为字符串。
import time
current_time = time.localtime()
formatted_time = time.asctime(current_time)
print(f"asctime格式化后的时间为: {formatted_time}")
time_stamp = time.time()
formatted_time = time.ctime(time_stamp)
print(f"ctime格式化后的时间为: {formatted_time}")
六、实例应用
- 定时任务
通过sleep()
函数可以创建简单的定时任务。例如,每隔10秒打印一次当前时间。
import time
while True:
current_time = time.strftime("%Y-%m-%d %H:%M:%S", time.localtime())
print(f"当前时间为: {current_time}")
time.sleep(10)
- 倒计时
实现一个简单的倒计时功能。
import time
def countdown(seconds):
while seconds:
mins, secs = divmod(seconds, 60)
timeformat = f"{mins:02}:{secs:02}"
print(timeformat, end='\r')
time.sleep(1)
seconds -= 1
print("倒计时结束!")
countdown(10)
- 计算函数执行时间
通过记录函数执行前后的时间戳,可以计算函数的执行时间。
import time
def example_function():
time.sleep(2) # 模拟函数执行时间
start_time = time.time()
example_function()
end_time = time.time()
print(f"函数执行时间为: {end_time - start_time} 秒")
七、时间处理的注意事项
- 时区问题
使用localtime()
和gmtime()
时要注意时区的差异,特别是在需要处理跨时区时间的场景中。
- 时间精度
time()
函数返回的时间戳是浮点数,可以提供亚秒级的精度。如果需要更高精度的时间测量,可以使用time.perf_counter()
或time.process_time()
。
import time
start_time = time.perf_counter()
time.sleep(1)
end_time = time.perf_counter()
print(f"高精度时间测量: {end_time - start_time} 秒")
- 时间格式
在使用strftime()
和strptime()
时要确保格式字符串与时间字符串匹配,否则会引发解析错误。
import time
time_string = "2023-10-01 12:00:00"
try:
parsed_time = time.strptime(time_string, "%Y-%m-%d %H:%M:%S")
print(f"解析成功: {parsed_time}")
except ValueError as e:
print(f"解析失败: {e}")
通过以上介绍,我们可以看到Python的time模块提供了丰富的函数来处理各种时间和日期相关的操作。掌握这些函数的使用方法,可以帮助我们更高效地完成时间处理任务。希望这篇文章对您深入了解和应用Python的time模块有所帮助。
相关问答FAQs:
如何在Python中使用time模块来获取当前时间?
使用time模块,可以通过time.time()函数获取当前的时间戳,单位为秒。时间戳表示从1970年1月1日(UTC时间)到现在的总秒数。此外,time.localtime()和time.strftime()函数可以将时间戳转换为当地时间格式,方便人类阅读。例如,使用time.localtime()
获取当前时间的结构化表示,接着用time.strftime('%Y-%m-%d %H:%M:%S', time.localtime())
将其格式化为字符串。
在Python的time模块中如何实现时间延迟?
time模块提供了time.sleep(seconds)函数,允许程序暂停指定的秒数。这在需要控制程序执行速度或等待特定事件发生时非常实用。例如,可以使用time.sleep(5)
使程序暂停5秒,适用于网络请求的重试机制或定时任务。
time模块中有哪些常用的时间格式化方法?
time模块中常用的时间格式化方法包括time.strftime(format, t)和time.strptime(string, format)。strftime用于将时间元组格式化为指定字符串格式,而strptime用于将字符串解析为时间元组。例如,time.strftime('%Y-%m-%d', time.localtime())
可用于获取当前日期的字符串格式,而time.strptime('2023-10-01', '%Y-%m-%d')
则能将字符串转换为时间元组,方便后续的时间计算和比较。
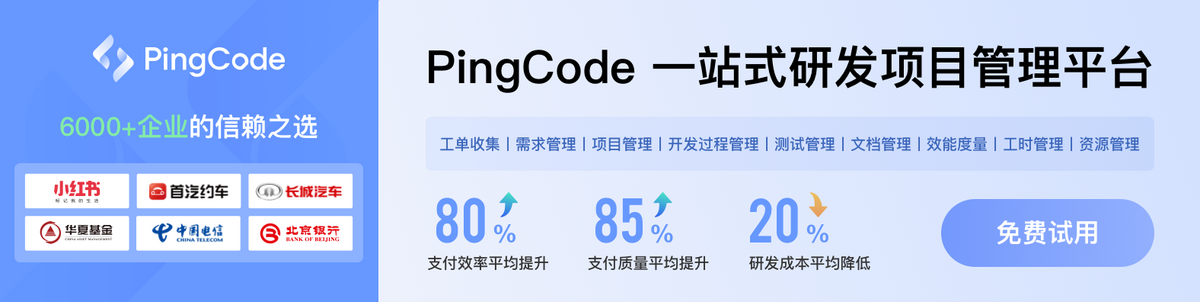