在Python中,可以使用多种方法来判断某个值是否存在。常用的方法包括使用“in”关键字、try-except结构、以及通过函数和方法进行判断。其中,使用“in”关键字是最常见的方式,因为它简洁且高效。通过“in”关键字,可以判断某个值是否存在于列表、字典、集合、元组等数据结构中。
例如,使用“in”关键字判断某个值是否存在于列表中,可以这样写:
my_list = [1, 2, 3, 4, 5]
if 3 in my_list:
print("3 exists in the list")
else:
print("3 does not exist in the list")
这种方法简单明了,对于大多数情况来说非常实用。
一、使用“in”关键字
使用“in”关键字是判断值是否存在于某个数据结构中的最常见方法。这种方法适用于列表、字典、集合、元组等。
1. 列表(List)
在列表中检查某个值是否存在,可以直接使用“in”关键字:
my_list = [10, 20, 30, 40, 50]
if 20 in my_list:
print("20 exists in the list")
else:
print("20 does not exist in the list")
这种方法的时间复杂度是O(n),因为在最坏情况下需要遍历整个列表来查找值。
2. 字典(Dictionary)
在字典中,通常检查某个键是否存在,而不是值。使用“in”关键字可以轻松实现这一点:
my_dict = {'a': 1, 'b': 2, 'c': 3}
if 'b' in my_dict:
print("'b' key exists in the dictionary")
else:
print("'b' key does not exist in the dictionary")
字典的键查找时间复杂度是O(1),因为字典是基于哈希表实现的。
3. 集合(Set)
集合也是基于哈希表实现的,因此查找时间复杂度同样是O(1)。可以使用“in”关键字来检查值是否在集合中:
my_set = {1, 2, 3, 4, 5}
if 4 in my_set:
print("4 exists in the set")
else:
print("4 does not exist in the set")
4. 元组(Tuple)
元组是不可变的列表,判断值是否存在的方式与列表相同:
my_tuple = (1, 2, 3, 4, 5)
if 5 in my_tuple:
print("5 exists in the tuple")
else:
print("5 does not exist in the tuple")
二、使用try-except结构
对于某些特定情况,如访问字典中某个键的值,可以使用try-except结构来判断键是否存在。这种方法在处理字典和列表时非常有用,尤其是在你需要处理键或索引不存在的情况时。
1. 字典(Dictionary)
使用try-except结构来判断字典中是否存在某个键:
my_dict = {'x': 10, 'y': 20, 'z': 30}
try:
value = my_dict['y']
print(f"The value for 'y' is {value}")
except KeyError:
print("'y' key does not exist in the dictionary")
2. 列表(List)
在列表中使用try-except结构来判断索引是否存在:
my_list = [100, 200, 300, 400, 500]
try:
value = my_list[2]
print(f"The value at index 2 is {value}")
except IndexError:
print("Index 2 does not exist in the list")
三、使用函数和方法
除了上述方法,还可以使用一些Python内置函数和方法来判断值是否存在。
1. get()方法(字典)
字典的get()方法可以用来判断键是否存在,同时可以提供默认值:
my_dict = {'a': 1, 'b': 2, 'c': 3}
value = my_dict.get('d', 'Key not found')
print(value) # 输出:Key not found
2. count()方法(列表和元组)
count()方法可以用来判断某个值在列表或元组中出现的次数,从而间接判断值是否存在:
my_list = [1, 2, 3, 2, 4, 2]
if my_list.count(2) > 0:
print("2 exists in the list")
else:
print("2 does not exist in the list")
四、使用any()函数
any()函数可以结合生成器表达式或列表推导式,来判断复杂的存在性条件:
my_list_of_dicts = [{'id': 1, 'name': 'Alice'}, {'id': 2, 'name': 'Bob'}, {'id': 3, 'name': 'Charlie'}]
if any(d['id'] == 2 for d in my_list_of_dicts):
print("A dictionary with id 2 exists in the list")
else:
print("No dictionary with id 2 exists in the list")
五、使用自定义函数
在某些情况下,可能需要定义自己的函数来判断值是否存在。这通常用于更复杂的数据结构或特定的应用场景。
def value_exists(data, value):
if isinstance(data, dict):
return value in data
elif isinstance(data, (list, tuple, set)):
return value in data
else:
raise TypeError("Unsupported data type")
示例
my_data = [1, 2, 3, 4, 5]
if value_exists(my_data, 3):
print("3 exists in the data")
else:
print("3 does not exist in the data")
六、使用正则表达式
在字符串中查找子串时,可以使用正则表达式来判断特定模式是否存在:
import re
text = "Hello, welcome to the world of Python."
pattern = "Python"
if re.search(pattern, text):
print("Pattern exists in the text")
else:
print("Pattern does not exist in the text")
七、使用numpy库
对于处理大型数组,numpy库提供了一些高效的方法来判断值是否存在:
import numpy as np
array = np.array([1, 2, 3, 4, 5])
value_to_check = 3
if value_to_check in array:
print(f"{value_to_check} exists in the array")
else:
print(f"{value_to_check} does not exist in the array")
八、使用pandas库
在数据分析中,pandas库广泛用于处理数据框和系列。可以使用pandas提供的方法来判断值是否存在:
1. 数据框(DataFrame)
import pandas as pd
df = pd.DataFrame({'A': [1, 2, 3], 'B': [4, 5, 6]})
if (df == 5).any().any():
print("5 exists in the DataFrame")
else:
print("5 does not exist in the DataFrame")
2. 系列(Series)
import pandas as pd
series = pd.Series([1, 2, 3, 4, 5])
if series.isin([3]).any():
print("3 exists in the Series")
else:
print("3 does not exist in the Series")
九、使用内置的下一代生成器表达式
在处理大量数据或需要懒加载时,可以使用生成器表达式与内置的next函数结合来判断值是否存在:
my_list = [1, 2, 3, 4, 5]
gen = (x for x in my_list if x == 3)
if next(gen, None) is not None:
print("3 exists in the list")
else:
print("3 does not exist in the list")
十、使用递归(适用于嵌套数据结构)
对于嵌套的数据结构,可以使用递归函数来判断值是否存在:
def recursive_search(data, value):
if isinstance(data, dict):
return value in data or any(recursive_search(v, value) for v in data.values())
elif isinstance(data, (list, tuple, set)):
return any(recursive_search(item, value) for item in data)
else:
return data == value
nested_data = {'a': [1, {'b': 2, 'c': [3, 4]}]}
if recursive_search(nested_data, 3):
print("3 exists in the nested data structure")
else:
print("3 does not exist in the nested data structure")
以上是多种在Python中判断某个值是否存在的方法。选择哪种方法取决于具体的应用场景和数据结构。希望这些方法能帮助你更高效地进行数据处理和判断。
相关问答FAQs:
如何在Python中检查一个值是否存在于列表或字典中?
在Python中,可以使用in
关键字来判断一个值是否存在于列表或字典中。例如,使用value in list
可以检查一个值是否在列表中,而key in dict
可以用来检查一个键是否在字典中。这种方法简洁且高效,适用于各种数据类型的集合。
在Python中,如何处理不存在的值以避免错误?
为了避免在查找不存在的值时引发错误,可以使用try-except
结构来捕获异常。对于字典,使用dict.get(key, default)
方法可以安全地获取值,如果键不存在,则返回一个默认值。这样可以确保代码的健壮性,避免因访问不存在的元素而导致的崩溃。
使用Python判断值是否存在时,性能会受到影响吗?
在处理大型数据集时,检查值的存在性可能会影响性能。列表的查找操作是O(n)时间复杂度,而集合和字典的查找操作是O(1)。因此,如果你需要频繁检查值的存在性,使用集合或字典通常会更高效。可以根据具体场景选择合适的数据结构以优化性能。
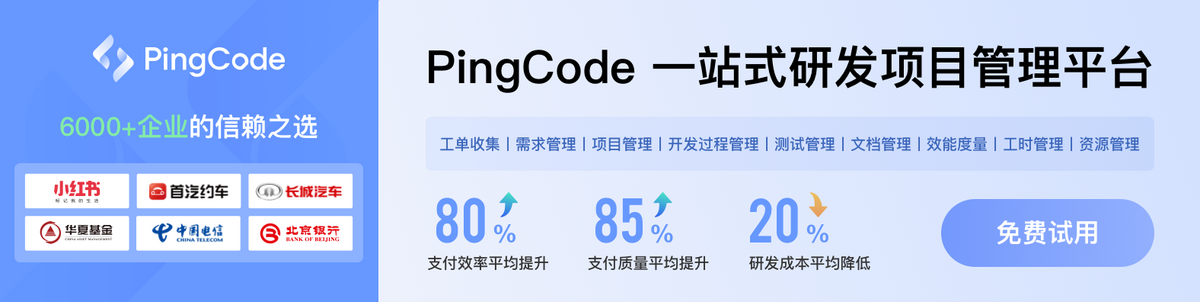