查看.txt文件编码的方法有多种,常见的有:使用chardet库、使用open函数的errors参数、使用codecs库。在实际操作中,使用chardet库是最方便的,因为它能够自动检测文件的编码类型。接下来,我们将详细介绍这三种方法。
一、使用chardet库
chardet库是一个用于检测文件编码的第三方库,能够自动检测文件的编码类型。
安装chardet库
首先,你需要安装chardet库。可以使用pip进行安装:
pip install chardet
使用chardet检测文件编码
安装完成后,可以使用以下代码来检测文件的编码:
import chardet
def detect_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
result = chardet.detect(raw_data)
encoding = result['encoding']
print(f"Detected encoding: {encoding}")
return encoding
示例
file_path = 'example.txt'
encoding = detect_encoding(file_path)
解释:
- 打开文件并读取内容为二进制数据。
- 使用chardet.detect()函数检测编码类型。
- 打印并返回检测到的编码类型。
二、使用open函数的errors参数
Python的内置open函数提供了一个errors参数,可以帮助我们识别文件编码错误。
示例代码
def read_file_with_errors(file_path):
try:
with open(file_path, 'r', errors='strict') as file:
content = file.read()
print("File read successfully with default encoding.")
except UnicodeDecodeError:
print("UnicodeDecodeError detected. File encoding may not be UTF-8 or default encoding.")
# 进一步处理,如尝试其他编码
示例
file_path = 'example.txt'
read_file_with_errors(file_path)
解释:
- 使用open函数尝试读取文件内容。
- 如果遇到UnicodeDecodeError错误,说明文件不是默认编码(通常是UTF-8)。
- 可以进一步处理错误,如尝试其他编码。
三、使用codecs库
codecs库也是Python内置库,可以用于处理不同编码的文件。
示例代码
import codecs
def read_file_with_codecs(file_path):
try:
with codecs.open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
print("File read successfully with UTF-8 encoding.")
except UnicodeDecodeError:
print("UnicodeDecodeError detected. Trying another encoding...")
# 尝试其他编码,如ISO-8859-1
with codecs.open(file_path, 'r', encoding='iso-8859-1') as file:
content = file.read()
print("File read successfully with ISO-8859-1 encoding.")
示例
file_path = 'example.txt'
read_file_with_codecs(file_path)
解释:
- 使用codecs.open函数尝试读取文件内容,指定编码为UTF-8。
- 如果遇到UnicodeDecodeError错误,说明文件不是UTF-8编码。
- 尝试使用其他编码(如ISO-8859-1)读取文件。
结论
通过上述方法,可以有效地检测和读取不同编码的.txt文件。使用chardet库是最简便的方法,因为它能够自动检测文件的编码类型。此外,使用open函数和codecs库也能帮助我们处理编码问题。在实际应用中,可以根据具体需求选择合适的方法。
相关问答FAQs:
如何在Python中识别文本文件的编码格式?
在Python中,您可以使用chardet
库来自动检测文本文件的编码格式。首先,确保安装了该库,可以通过pip install chardet
进行安装。接下来,您可以使用以下代码读取文件并检测其编码:
import chardet
with open('yourfile.txt', 'rb') as f:
raw_data = f.read()
result = chardet.detect(raw_data)
encoding = result['encoding']
print(f"文件编码是: {encoding}")
使用Python查看文件编码的其他方法有哪些?
除了使用chardet
库,您还可以利用codecs
模块来尝试打开文件,捕获可能的编码错误,进而推断文件编码。以下是一个示例代码:
import codecs
file_path = 'yourfile.txt'
try:
with codecs.open(file_path, 'r', 'utf-8') as f:
f.read()
encoding = 'utf-8'
except UnicodeDecodeError:
encoding = '其他编码'
print(f"推测的文件编码是: {encoding}")
如果文件编码不正确,如何处理?
如果在读取文件时遇到编码错误,可以使用errors
参数来指定如何处理编码问题。比如,您可以选择忽略错误或使用替代字符:
with open('yourfile.txt', 'r', encoding='utf-8', errors='ignore') as f:
content = f.read()
这将允许您读取文件而不会因编码错误而中断。确保根据实际需求选择合适的错误处理方式。
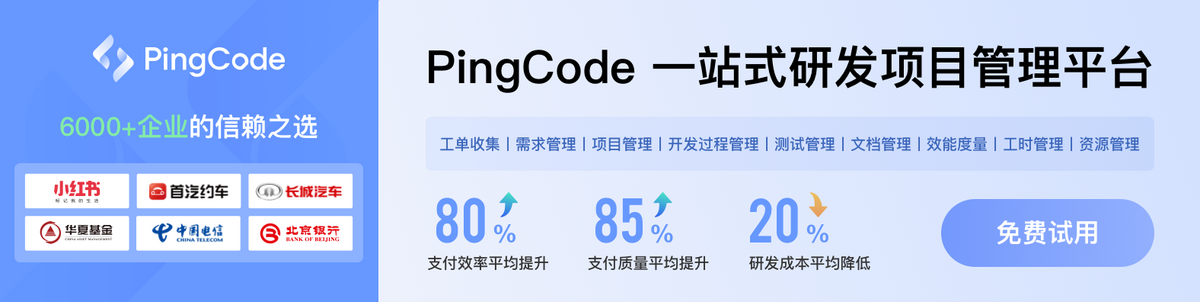