在Python中,全选某一条指令的方式有多种。主要的方法有:使用内置函数、使用外部库、使用正则表达式等。最常用的方法包括:使用字符串操作、使用列表解析、使用正则表达式。接下来,我将详细介绍如何使用这些方法在Python中全选某一条指令,并给出具体的代码示例。
一、字符串操作
使用字符串操作是最简单的方式之一。通过字符串的内置方法,可以轻松地找到并选中特定的指令或字符串。
text = "This is a sample text. Select the word 'sample' in this text."
keyword = "sample"
使用find方法找到指令的起始位置
start_index = text.find(keyword)
if start_index != -1:
end_index = start_index + len(keyword)
selected_text = text[start_index:end_index]
print(f"Selected text: {selected_text}")
else:
print("Keyword not found.")
在这个示例中,我们使用了find
方法来找到关键字的起始位置,并使用切片操作来获取该关键字。
二、列表解析
列表解析是一种简洁的方式,可以用于在数据集中找到并选中特定的指令或元素。
data = ["apple", "banana", "cherry", "date", "elderberry"]
keyword = "cherry"
使用列表解析找到并选中关键字
selected_items = [item for item in data if item == keyword]
print(f"Selected items: {selected_items}")
在这个示例中,我们使用列表解析来遍历数据集,并选中与关键字匹配的元素。
三、正则表达式
正则表达式是一种强大的工具,可以用于匹配复杂的模式和选中特定的指令。
import re
text = "This is a sample text. Select the word 'sample' in this text."
pattern = r'sample'
使用正则表达式找到并选中指令
matches = re.findall(pattern, text)
if matches:
print(f"Selected text: {matches}")
else:
print("Keyword not found.")
在这个示例中,我们使用了re.findall
方法来找到所有匹配模式的指令,并返回一个列表。
四、使用外部库
有许多外部库可以用于处理文本和选中特定的指令。例如,pandas
库可以用于处理数据集,并轻松地找到和选中特定的指令。
import pandas as pd
data = pd.Series(["apple", "banana", "cherry", "date", "elderberry"])
keyword = "cherry"
使用pandas库找到并选中关键字
selected_items = data[data == keyword]
print(f"Selected items: {selected_items.tolist()}")
在这个示例中,我们使用pandas
库来处理数据集,并选中与关键字匹配的元素。
五、使用函数封装
为了提高代码的可重用性,可以将选中指令的逻辑封装到一个函数中。
def select_text(text, keyword):
start_index = text.find(keyword)
if start_index != -1:
end_index = start_index + len(keyword)
return text[start_index:end_index]
return "Keyword not found."
示例使用
text = "This is a sample text. Select the word 'sample' in this text."
keyword = "sample"
selected_text = select_text(text, keyword)
print(f"Selected text: {selected_text}")
在这个示例中,我们定义了一个select_text
函数,用于选中文本中的关键字。
六、结合多种方法
在实际应用中,有时需要结合多种方法来实现更复杂的选中逻辑。例如,可以先使用正则表达式找到匹配的模式,然后使用字符串操作来进一步处理。
import re
def select_text_advanced(text, pattern):
matches = re.finditer(pattern, text)
selected_texts = []
for match in matches:
start_index = match.start()
end_index = match.end()
selected_texts.append(text[start_index:end_index])
return selected_texts
示例使用
text = "This is a sample text. Select the word 'sample' in this text. Another sample here."
pattern = r'sample'
selected_texts = select_text_advanced(text, pattern)
print(f"Selected texts: {selected_texts}")
在这个示例中,我们使用了re.finditer
方法来找到所有匹配模式的指令,并使用字符串操作来获取每个匹配的文本。
七、处理大规模数据
在处理大规模数据时,性能是一个重要的考虑因素。可以使用更高效的数据结构和算法来提高选中指令的性能。例如,使用numpy
库来处理大型数组。
import numpy as np
data = np.array(["apple", "banana", "cherry", "date", "elderberry", "cherry", "cherry"])
keyword = "cherry"
使用numpy库找到并选中关键字
selected_indices = np.where(data == keyword)[0]
selected_items = data[selected_indices]
print(f"Selected items: {selected_items}")
在这个示例中,我们使用numpy
库来处理大型数组,并选中与关键字匹配的元素。
八、处理复杂文本
在处理复杂文本时,可能需要使用自然语言处理(NLP)技术来更准确地选中指令。例如,可以使用spaCy
库来进行词性标注和命名实体识别。
import spacy
加载预训练的spaCy模型
nlp = spacy.load("en_core_web_sm")
text = "Apple is looking at buying U.K. startup for $1 billion. The company was founded by Steve Jobs."
keyword = "Apple"
使用spaCy库进行词性标注和命名实体识别
doc = nlp(text)
selected_entities = [ent.text for ent in doc.ents if ent.text == keyword]
print(f"Selected entities: {selected_entities}")
在这个示例中,我们使用spaCy
库来进行命名实体识别,并选中与关键字匹配的实体。
九、处理多行文本
在处理多行文本时,可以使用多行字符串操作来选中特定的指令。例如,使用splitlines
方法来按行分割文本,并遍历每一行来查找关键字。
text = """This is the first line.
This is the second line with sample.
This is the third line with another sample."""
keyword = "sample"
按行分割文本并查找关键字
lines = text.splitlines()
selected_lines = [line for line in lines if keyword in line]
print(f"Selected lines: {selected_lines}")
在这个示例中,我们使用splitlines
方法来按行分割文本,并选中包含关键字的行。
十、处理结构化数据
在处理结构化数据时,可以使用专门的库来处理和选中特定的指令。例如,使用xml.etree.ElementTree
库来处理XML数据。
import xml.etree.ElementTree as ET
xml_data = """
<root>
<item>apple</item>
<item>banana</item>
<item>cherry</item>
<item>date</item>
<item>elderberry</item>
</root>
"""
keyword = "cherry"
解析XML数据并查找关键字
root = ET.fromstring(xml_data)
selected_items = [item.text for item in root.findall('item') if item.text == keyword]
print(f"Selected items: {selected_items}")
在这个示例中,我们使用xml.etree.ElementTree
库来解析XML数据,并选中与关键字匹配的元素。
总结
在Python中,全选某一条指令的方法有很多,可以根据具体的需求选择合适的方法。常用的方法包括字符串操作、列表解析、正则表达式、使用外部库等。对于复杂的应用场景,可以结合多种方法来实现更高效和准确的选中逻辑。同时,在处理大规模数据和复杂文本时,性能和准确性是需要重点考虑的因素。通过合理地选择和组合这些方法,可以有效地实现Python中的全选指令操作。
相关问答FAQs:
如何在Python中选择特定的指令或代码块?
在Python中,选择特定的指令通常涉及到使用条件语句,例如if
语句。你可以根据条件来执行特定的代码。例如:
if condition:
# 执行特定指令
这种方式允许你根据程序的运行情况,灵活选择执行的指令。
在Python中,有哪些方法可以批量选择和执行多条指令?
你可以使用函数、循环或列表推导式来批量选择和执行多条指令。定义一个函数,将需要执行的指令放入其中,然后调用该函数。例如:
def my_function():
# 多条指令
my_function()
此外,使用for
循环可以对一系列数据进行操作,从而有效地选择和执行多条指令。
怎样在Python中使用条件选择来控制指令的执行?
通过使用if-elif-else
结构,你可以精确控制指令的执行。根据不同的条件,选择执行不同的指令。例如:
if condition1:
# 指令1
elif condition2:
# 指令2
else:
# 默认指令
这种结构使得代码更具可读性和维护性,便于根据不同情况选择执行的指令。
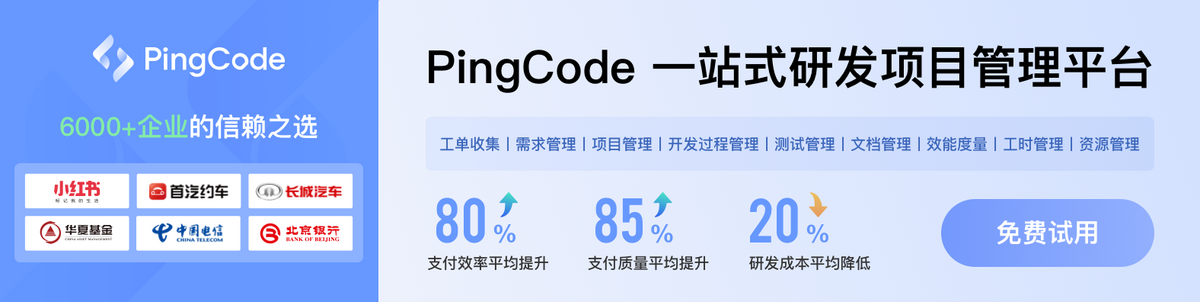