Python调用C模块的方法有多种,主要包括使用ctypes、cffi、SWIG和编写Python扩展模块。对于简单和常见的需求,可以使用ctypes或cffi,而对于需要更高性能或复杂交互的场景,编写Python扩展模块是更好的选择。
为了详细描述其中一种方法,本文将重点介绍如何使用ctypes来调用C模块,并涵盖其他几种方法的基本步骤和使用场景。
一、使用CTYPES调用C模块
-
编写C代码并生成共享库
首先,我们需要编写一个简单的C程序,并将其编译成共享库(.so文件)。
// example.c
#include <stdio.h>
void hello_world() {
printf("Hello, World from C!\n");
}
int add(int a, int b) {
return a + b;
}
编译该C代码生成共享库:
gcc -shared -o example.so -fPIC example.c
-
在Python中使用ctypes加载共享库
接下来,我们在Python代码中使用ctypes加载并调用C函数。
import ctypes
加载共享库
example = ctypes.CDLL('./example.so')
调用C函数
example.hello_world()
调用带参数的C函数
result = example.add(5, 3)
print(f'Result of add function: {result}')
-
处理复杂数据类型
ctypes还支持处理复杂的数据类型,如数组、结构体等。
// example.c
#include <stdio.h>
typedef struct {
int x;
int y;
} Point;
int distance(Point p1, Point p2) {
return (p2.x - p1.x) * (p2.x - p1.x) + (p2.y - p1.y) * (p2.y - p1.y);
}
import ctypes
class Point(ctypes.Structure):
_fields_ = [("x", ctypes.c_int),
("y", ctypes.c_int)]
example = ctypes.CDLL('./example.so')
定义函数参数和返回类型
example.distance.argtypes = [Point, Point]
example.distance.restype = ctypes.c_int
p1 = Point(1, 2)
p2 = Point(4, 6)
dist = example.distance(p1, p2)
print(f'Distance: {dist}')
二、使用CFFI调用C模块
-
编写C代码
与ctypes类似,首先编写C代码并生成共享库。
-
在Python中使用cffi
使用cffi库加载并调用C函数。
from cffi import FFI
ffi = FFI()
定义C函数原型
ffi.cdef("""
void hello_world();
int add(int a, int b);
""")
加载共享库
example = ffi.dlopen('./example.so')
调用C函数
example.hello_world()
result = example.add(5, 3)
print(f'Result of add function: {result}')
三、使用SWIG调用C模块
-
编写C代码
与前面的方法一样,编写C代码并生成共享库。
-
编写SWIG接口文件
创建一个SWIG接口文件(example.i)。
%module example
%{
#include "example.h"
%}
void hello_world();
int add(int a, int b);
-
生成Python包装代码
使用SWIG工具生成Python包装代码。
swig -python -o example_wrap.c example.i
gcc -shared -o _example.so example_wrap.c example.c -I/usr/include/python3.8
-
在Python中调用C函数
使用生成的Python包装模块调用C函数。
import example
example.hello_world()
result = example.add(5, 3)
print(f'Result of add function: {result}')
四、编写Python扩展模块
-
编写C扩展代码
编写C扩展代码,该代码需要包含Python.h头文件。
#include <Python.h>
static PyObject* py_hello_world(PyObject* self, PyObject* args) {
printf("Hello, World from C!\n");
Py_RETURN_NONE;
}
static PyObject* py_add(PyObject* self, PyObject* args) {
int a, b;
if (!PyArg_ParseTuple(args, "ii", &a, &b)) {
return NULL;
}
return PyLong_FromLong(a + b);
}
static PyMethodDef ExampleMethods[] = {
{"hello_world", py_hello_world, METH_NOARGS, "Print hello world"},
{"add", py_add, METH_VARARGS, "Add two numbers"},
{NULL, NULL, 0, NULL}
};
static struct PyModuleDef examplemodule = {
PyModuleDef_HEAD_INIT,
"example",
NULL,
-1,
ExampleMethods
};
PyMODINIT_FUNC PyInit_example(void) {
return PyModule_Create(&examplemodule);
}
-
编写setup.py文件
编写setup.py文件用于编译和安装扩展模块。
from setuptools import setup, Extension
module = Extension('example', sources=['example.c'])
setup(
name='example',
version='1.0',
description='Python Package with C Extension',
ext_modules=[module],
)
-
编译并安装扩展模块
使用setup.py编译并安装扩展模块。
python setup.py build
python setup.py install
-
在Python中调用C函数
使用扩展模块调用C函数。
import example
example.hello_world()
result = example.add(5, 3)
print(f'Result of add function: {result}')
总结,Python调用C模块的方法有多种,选择哪种方法取决于具体需求和复杂度。对于简单的需求,可以使用ctypes或cffi,而对于需要更高性能或复杂交互的场景,编写Python扩展模块是更好的选择。SWIG可以简化C代码的包装过程,但可能需要额外的学习成本。无论选择哪种方法,都需要对C语言和Python的基本知识有一定的掌握。
相关问答FAQs:
如何在Python中加载和使用C模块?
在Python中加载C模块的过程通常涉及使用Python的C API或通过ctypes和cffi库来实现。你需要先编写C代码并编译成共享库文件(如.so或.dll),然后在Python中通过import
语句或这些库来加载它。具体步骤包括:编写C代码、编译代码、确保共享库路径正确、在Python脚本中导入模块并调用相关函数。
调用C模块时需要注意哪些事项?
在调用C模块时,确保C代码中定义的函数与Python的期望参数类型相匹配是非常重要的。此外,你还需要处理内存管理问题,确保在C和Python之间正确分配和释放内存。错误的内存管理可能导致程序崩溃或内存泄漏。
如何使用ctypes库调用C函数?
使用ctypes库调用C函数的步骤包括:首先,使用ctypes加载共享库,然后声明C函数的参数和返回值类型。接下来,可以直接调用这些函数。确保C函数的参数类型与Python中的类型相对应,以避免类型不匹配引发的错误。
在Python中使用C扩展模块的性能优势是什么?
使用C扩展模块可以显著提高性能,尤其是在执行计算密集型任务时。C语言的执行速度远快于Python,因此将性能关键的代码部分用C实现,可以有效提升整体应用程序的性能。同时,C扩展允许开发者利用现有的C库,扩展Python的功能。
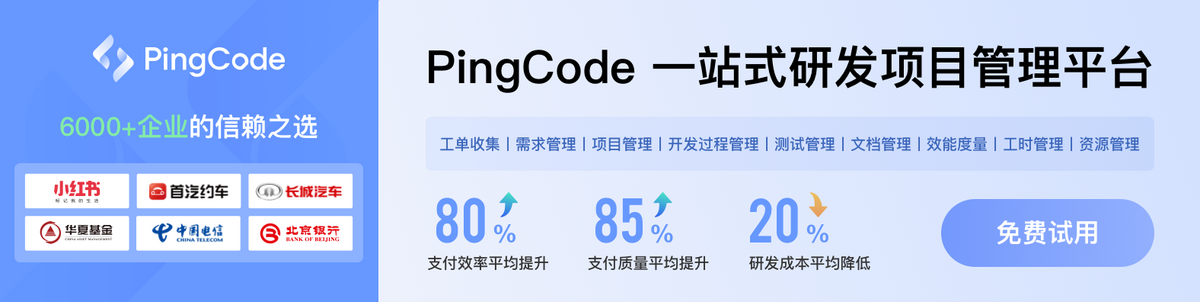