使用Python显示当前时间的方法有多种,主要包括使用datetime模块、time模块和pytz模块。这些模块提供了丰富的功能,可以根据需求灵活选择和组合使用。datetime模块功能强大、易于使用、支持时区操作。下面将详细介绍使用datetime模块的基本方法,并进一步探讨如何根据需求进行扩展和优化。
一、使用datetime模块
1、获取当前时间
Python的datetime模块提供了datetime类,该类有一个now()方法,可以获取当前的日期和时间。我们可以通过datetime.datetime.now()来显示当前时间。
import datetime
current_time = datetime.datetime.now()
print("当前时间:", current_time)
2、格式化显示时间
可以通过strftime()方法将时间格式化为特定的字符串形式。strftime()方法接受一个格式化字符串作为参数,用于指定时间的显示格式。常见的格式化字符包括:
- %Y: 四位数的年份
- %m: 两位数的月份
- %d: 两位数的日期
- %H: 两位数的小时(24小时制)
- %M: 两位数的分钟
- %S: 两位数的秒
formatted_time = current_time.strftime("%Y-%m-%d %H:%M:%S")
print("格式化后的时间:", formatted_time)
3、获取特定的时间元素
datetime对象提供了许多属性,可以获取特定的时间元素,如年、月、日、时、分、秒等。
year = current_time.year
month = current_time.month
day = current_time.day
hour = current_time.hour
minute = current_time.minute
second = current_time.second
print(f"年: {year}, 月: {month}, 日: {day}, 时: {hour}, 分: {minute}, 秒: {second}")
二、使用time模块
time模块提供了许多与时间相关的函数,也可以用于显示当前时间。尽管time模块的功能不如datetime模块全面,但在某些场景中仍然非常有用。
1、获取当前时间
time模块提供了time()函数,可以获取当前时间的时间戳(自1970年1月1日以来的秒数)。
import time
timestamp = time.time()
print("当前时间戳:", timestamp)
2、格式化显示时间
time模块的localtime()函数将时间戳转换为struct_time对象,而strftime()函数可以将struct_time对象格式化为字符串。
local_time = time.localtime(timestamp)
formatted_time = time.strftime("%Y-%m-%d %H:%M:%S", local_time)
print("格式化后的时间:", formatted_time)
三、使用pytz模块
pytz模块提供了对时区的支持,可以用于显示带有时区的当前时间。使用pytz模块可以轻松实现跨时区的时间转换和显示。
1、安装pytz模块
首先需要安装pytz模块,可以使用pip命令进行安装:
pip install pytz
2、获取当前时间并显示时区
可以使用pytz模块获取带有时区信息的当前时间,并根据需要进行格式化显示。
from datetime import datetime
import pytz
timezone = pytz.timezone('Asia/Shanghai')
current_time = datetime.now(timezone)
formatted_time = current_time.strftime("%Y-%m-%d %H:%M:%S %Z%z")
print("当前时间(带时区):", formatted_time)
3、时区转换
pytz模块还可以方便地进行时区转换。例如,将当前时间转换为美国东部时间(EST)。
eastern_timezone = pytz.timezone('US/Eastern')
eastern_time = current_time.astimezone(eastern_timezone)
formatted_eastern_time = eastern_time.strftime("%Y-%m-%d %H:%M:%S %Z%z")
print("美国东部时间:", formatted_eastern_time)
四、结合使用
在实际应用中,可以结合使用上述方法,根据具体需求灵活选择和组合。例如,可以使用datetime模块获取当前时间,使用time模块格式化显示时间,使用pytz模块处理时区问题。
import datetime
import time
import pytz
获取当前时间
current_time = datetime.datetime.now()
格式化显示时间
formatted_time = current_time.strftime("%Y-%m-%d %H:%M:%S")
print("格式化后的时间:", formatted_time)
获取带有时区的当前时间
timezone = pytz.timezone('Asia/Shanghai')
current_time_with_timezone = datetime.datetime.now(timezone)
formatted_time_with_timezone = current_time_with_timezone.strftime("%Y-%m-%d %H:%M:%S %Z%z")
print("当前时间(带时区):", formatted_time_with_timezone)
时区转换
eastern_timezone = pytz.timezone('US/Eastern')
eastern_time = current_time_with_timezone.astimezone(eastern_timezone)
formatted_eastern_time = eastern_time.strftime("%Y-%m-%d %H:%M:%S %Z%z")
print("美国东部时间:", formatted_eastern_time)
五、实践应用
在实际项目中,显示当前时间的需求非常普遍。以下是一些常见的应用场景:
1、日志记录
在记录日志时,通常需要记录日志发生的时间。可以使用datetime模块获取当前时间,并格式化为合适的字符串形式,用于记录日志。
import logging
import datetime
logging.basicConfig(level=logging.INFO)
def log_message(message):
current_time = datetime.datetime.now().strftime("%Y-%m-%d %H:%M:%S")
logging.info(f"{current_time} - {message}")
log_message("This is a log message.")
2、调度任务
在调度任务时,可能需要根据当前时间来决定任务的执行时间。可以使用datetime模块获取当前时间,并进行相应的计算。
import datetime
import time
def schedule_task(task, delay_seconds):
current_time = datetime.datetime.now()
scheduled_time = current_time + datetime.timedelta(seconds=delay_seconds)
print(f"Task scheduled to run at: {scheduled_time.strftime('%Y-%m-%d %H:%M:%S')}")
time.sleep(delay_seconds)
task()
def example_task():
print("Task executed.")
schedule_task(example_task, 5)
3、计时器
在开发计时器功能时,需要获取当前时间并计算时间差。可以使用datetime模块获取开始时间和结束时间,并计算时间差。
import datetime
def timer_example():
start_time = datetime.datetime.now()
print(f"Start time: {start_time.strftime('%Y-%m-%d %H:%M:%S')}")
# 模拟一些耗时操作
time.sleep(3)
end_time = datetime.datetime.now()
print(f"End time: {end_time.strftime('%Y-%m-%d %H:%M:%S')}")
time_diff = end_time - start_time
print(f"Time taken: {time_diff}")
timer_example()
通过上述方法,可以轻松地在Python程序中显示当前时间,并根据实际需求进行格式化、时区处理和应用。无论是记录日志、调度任务还是实现计时器功能,都可以灵活地使用datetime、time和pytz模块来满足需求。希望本文对您理解和使用Python显示当前时间有所帮助。
相关问答FAQs:
如何在Python中获取当前时间的不同格式?
在Python中,可以使用datetime
模块来获取当前时间并以多种格式显示。常见的格式包括“YYYY-MM-DD HH:MM:SS”、“DD/MM/YYYY”、“Month Day, Year”等。例如,可以通过datetime.now()
获取当前时间,然后使用strftime()
方法来格式化时间字符串。
显示当前时间时,如何处理时区问题?
处理时区时,建议使用pytz
库来确保时间的准确性。可以通过pytz.timezone()
指定时区,并结合datetime.now()
来获取特定时区的当前时间。这对于需要在全球范围内共享时间的应用程序尤为重要。
在Python中如何定期更新并显示当前时间?
可以使用while
循环和time.sleep()
函数来定期更新显示的时间。例如,每秒钟更新一次当前时间,可以使用print()
函数输出当前时间,并在循环中使用sleep(1)
来控制更新时间间隔。这种方法可以用来创建简单的时钟程序。
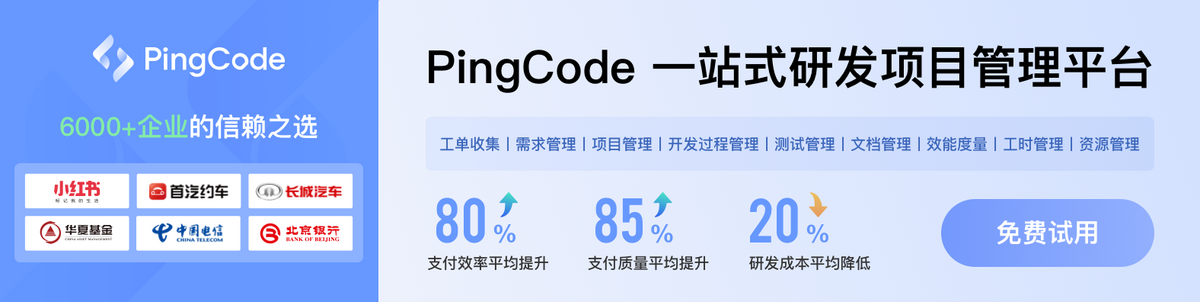