使用Python对比两个列表元素,可以通过多种方法实现,如使用集合(set)、列表推导式、for循环等。 在这篇文章中,我们将详细介绍这些方法,并对其中一种方法进行深入探讨。
一、使用集合对比
集合(set)是一种无序且唯一的元素集合。利用集合的这一特性,我们可以很方便地对比两个列表元素,找出它们的交集、并集和差集。
1.1 交集
交集是指两个列表中同时存在的元素。我们可以使用集合的intersection
方法来获取交集。
list1 = [1, 2, 3, 4, 5]
list2 = [4, 5, 6, 7, 8]
set1 = set(list1)
set2 = set(list2)
intersection = set1.intersection(set2)
print(intersection) # 输出: {4, 5}
1.2 并集
并集是指两个列表中所有不同的元素。我们可以使用集合的union
方法来获取并集。
union = set1.union(set2)
print(union) # 输出: {1, 2, 3, 4, 5, 6, 7, 8}
1.3 差集
差集是指在一个列表中存在但在另一个列表中不存在的元素。我们可以使用集合的difference
方法来获取差集。
difference = set1.difference(set2)
print(difference) # 输出: {1, 2, 3}
二、使用列表推导式对比
列表推导式是一种简洁且高效的方法,通过一行代码即可实现对比操作。
2.1 交集
intersection = [item for item in list1 if item in list2]
print(intersection) # 输出: [4, 5]
2.2 差集
difference = [item for item in list1 if item not in list2]
print(difference) # 输出: [1, 2, 3]
三、使用for循环对比
使用for循环对比列表元素虽然代码较长,但有助于理解对比过程。
3.1 交集
intersection = []
for item in list1:
if item in list2:
intersection.append(item)
print(intersection) # 输出: [4, 5]
3.2 差集
difference = []
for item in list1:
if item not in list2:
difference.append(item)
print(difference) # 输出: [1, 2, 3]
四、使用库函数对比
Python标准库提供了一些函数和模块,可以帮助我们更方便地对比列表元素。
4.1 使用collections.Counter
collections.Counter
是一个字典子类,用于计数对象的频次。我们可以利用它来对比两个列表元素。
from collections import Counter
list1 = [1, 2, 3, 4, 5]
list2 = [4, 5, 6, 7, 8]
counter1 = Counter(list1)
counter2 = Counter(list2)
intersection = list((counter1 & counter2).elements())
print(intersection) # 输出: [4, 5]
4.2 使用numpy库
numpy
库是一个强大的科学计算库,可以高效地对比两个列表元素。
import numpy as np
array1 = np.array(list1)
array2 = np.array(list2)
intersection = np.intersect1d(array1, array2)
print(intersection) # 输出: [4 5]
五、使用递归对比
递归是一种编程技巧,通过函数调用自身来解决问题。虽然递归对比列表元素不常见,但它是一种有趣且有效的方法。
def recursive_intersection(list1, list2, index=0):
if index == len(list1):
return []
elif list1[index] in list2:
return [list1[index]] + recursive_intersection(list1, list2, index + 1)
else:
return recursive_intersection(list1, list2, index + 1)
list1 = [1, 2, 3, 4, 5]
list2 = [4, 5, 6, 7, 8]
intersection = recursive_intersection(list1, list2)
print(intersection) # 输出: [4, 5]
六、对比两个列表的顺序和内容
有时候,我们不仅需要对比两个列表的内容,还需要对比它们的顺序。可以使用zip
函数和==
运算符来实现。
list1 = [1, 2, 3, 4, 5]
list2 = [1, 2, 3, 4, 5]
list3 = [5, 4, 3, 2, 1]
对比内容和顺序
print(list1 == list2) # 输出: True
print(list1 == list3) # 输出: False
对比内容,不考虑顺序
print(sorted(list1) == sorted(list2)) # 输出: True
print(sorted(list1) == sorted(list3)) # 输出: True
七、对比两个嵌套列表
嵌套列表是指列表中的元素仍然是列表。可以使用递归或深度对比来实现。
def deep_compare(list1, list2):
if len(list1) != len(list2):
return False
for item1, item2 in zip(list1, list2):
if isinstance(item1, list) and isinstance(item2, list):
if not deep_compare(item1, item2):
return False
elif item1 != item2:
return False
return True
nested_list1 = [[1, 2], [3, 4], [5, 6]]
nested_list2 = [[1, 2], [3, 4], [5, 6]]
nested_list3 = [[1, 2], [4, 3], [6, 5]]
print(deep_compare(nested_list1, nested_list2)) # 输出: True
print(deep_compare(nested_list1, nested_list3)) # 输出: False
八、性能对比
在选择对比方法时,性能也是一个重要考虑因素。对于大列表,集合和numpy
库通常比列表推导式和for循环更高效。
8.1 性能测试
我们可以使用timeit
模块来测试不同方法的性能。
import timeit
list1 = list(range(1000))
list2 = list(range(500, 1500))
使用集合对比
def set_intersection():
set1 = set(list1)
set2 = set(list2)
return set1.intersection(set2)
使用列表推导式对比
def list_comprehension_intersection():
return [item for item in list1 if item in list2]
使用for循环对比
def for_loop_intersection():
intersection = []
for item in list1:
if item in list2:
intersection.append(item)
return intersection
使用numpy对比
def numpy_intersection():
import numpy as np
array1 = np.array(list1)
array2 = np.array(list2)
return np.intersect1d(array1, array2)
print(timeit.timeit(set_intersection, number=1000)) # 测试集合
print(timeit.timeit(list_comprehension_intersection, number=1000)) # 测试列表推导式
print(timeit.timeit(for_loop_intersection, number=1000)) # 测试for循环
print(timeit.timeit(numpy_intersection, number=1000)) # 测试numpy
九、总结
对比两个列表元素的方法多种多样,包括使用集合、列表推导式、for循环、库函数和递归等。每种方法都有其优缺点,具体选择应根据实际需求和列表大小来决定。对于大规模列表,使用集合和numpy
库通常更高效,而对于小规模列表,列表推导式和for循环则更加直观易懂。
希望这篇文章能帮助您更好地理解和应用Python对比列表元素的方法。如果您有其他问题或见解,欢迎在评论区交流分享。
相关问答FAQs:
如何在Python中比较两个列表的相似性?
在Python中,可以使用集合(set)来比较两个列表的相似性。通过将两个列表转换为集合,可以轻松找出它们的交集、差集和并集。例如,使用set(list1) & set(list2)
可以获得两个列表中共同的元素,而set(list1) - set(list2)
则可以找出只存在于第一个列表中的元素。这种方法不仅简洁明了,还能有效处理重复元素。
在Python中,比较两个列表的元素顺序是否相同该怎么做?
要检查两个列表的元素是否相同且顺序一致,可以直接使用比较运算符==
。例如,list1 == list2
将返回True
,如果两个列表的长度、元素及其顺序都完全相同。如果只关心元素是否相同而不考虑顺序,可以使用集合的比较方法。
有没有方法可以找出两个列表之间的不同元素?
可以使用列表推导式来找出两个列表之间的不同元素。例如,通过[item for item in list1 if item not in list2]
可以得到只存在于第一个列表中的元素,反之亦然。结合这两种方法,可以全面了解两个列表之间的差异。还可以使用collections.Counter
来帮助比较频率,以识别出现次数不同的元素。
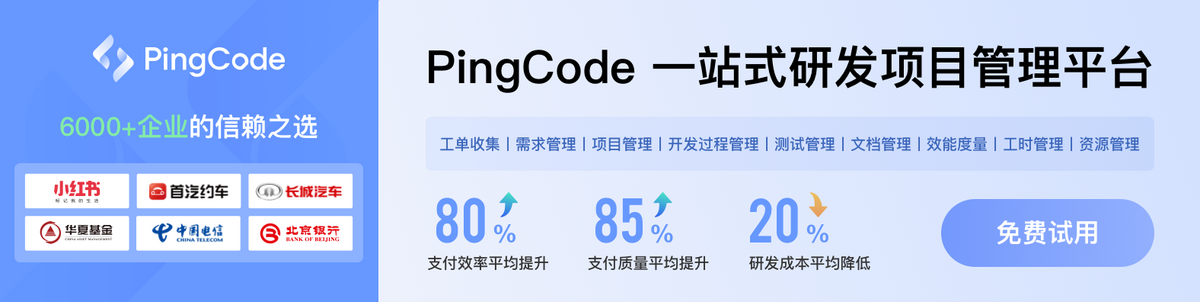