C语言转换为Python的核心步骤包括:理解C代码、分析C代码中的数据结构和算法、找出Python等效的语法和库函数、逐步重写代码。以下将详细描述将C语言转换为Python的每个步骤。
一、理解C代码
在将C语言代码转换为Python之前,首先需要彻底理解C代码。分析C代码的功能、数据结构、算法以及每个函数和变量的作用。通过阅读代码注释和运行代码,逐步掌握其工作原理。
二、分析C代码中的数据结构和算法
C语言和Python在数据结构和算法实现上存在一些差异。C语言中常用的数组、指针、结构体等数据结构,在Python中有不同的实现方式。了解这些差异,找到合适的Python等效实现是转换代码的关键。
数据结构
- 数组和列表
在C语言中,数组是固定大小的,而在Python中,列表是动态的,可以随时添加和删除元素。因此,C语言中的数组通常可以直接转换为Python中的列表。
// C语言数组
int arr[5] = {1, 2, 3, 4, 5};
# Python列表
arr = [1, 2, 3, 4, 5]
- 指针和引用
C语言中的指针用于直接操作内存地址,而Python中没有指针概念。Python中的变量引用内存地址,通过引用传递对象。因此,将C语言中的指针操作转换为Python时,需要理解指针的用途,并使用Python的引用特性来实现。
// C语言指针
int a = 10;
int *p = &a;
# Python引用
a = 10
p = a
- 结构体和类
C语言中的结构体在Python中可以用类来表示。结构体成员变量在Python类中作为实例变量,通过初始化方法(init)来定义。
// C语言结构体
struct Point {
int x;
int y;
};
struct Point p = {10, 20};
# Python类
class Point:
def __init__(self, x, y):
self.x = x
self.y = y
p = Point(10, 20)
算法
- 循环和条件语句
C语言中的for、while循环和if条件语句在Python中有类似的语法,只需稍作调整即可。
// C语言循环和条件语句
for (int i = 0; i < 5; i++) {
if (arr[i] % 2 == 0) {
printf("%d is even\n", arr[i]);
}
}
# Python循环和条件语句
for i in range(5):
if arr[i] % 2 == 0:
print(f"{arr[i]} is even")
- 函数和模块
C语言中的函数可以直接转换为Python中的函数。在C语言中,函数可以在多个文件中定义和调用,而在Python中,函数可以在模块中定义,并通过导入模块来调用。
// C语言函数
int add(int a, int b) {
return a + b;
}
# Python函数
def add(a, b):
return a + b
三、找出Python等效的语法和库函数
在将C代码转换为Python时,需要找出Python中等效的语法和库函数。例如,C语言中的标准库函数可以在Python中找到类似的实现。
输入输出
C语言中的输入输出函数(如printf、scanf等)在Python中有对应的实现(如print、input等)。
// C语言输入输出
#include <stdio.h>
int main() {
int a;
printf("Enter a number: ");
scanf("%d", &a);
printf("You entered: %d\n", a);
return 0;
}
# Python输入输出
a = int(input("Enter a number: "))
print(f"You entered: {a}")
文件操作
C语言中的文件操作函数(如fopen、fclose、fread、fwrite等)在Python中有对应的实现(如open、close、read、write等)。
// C语言文件操作
#include <stdio.h>
int main() {
FILE *fp;
char buffer[100];
fp = fopen("file.txt", "r");
if (fp == NULL) {
printf("Error opening file\n");
return 1;
}
while (fgets(buffer, sizeof(buffer), fp) != NULL) {
printf("%s", buffer);
}
fclose(fp);
return 0;
}
# Python文件操作
with open("file.txt", "r") as fp:
for line in fp:
print(line, end="")
动态内存分配
C语言中的动态内存分配函数(如malloc、calloc、free等)在Python中不需要显式使用。Python的内存管理是自动的,通过引用计数和垃圾回收机制来管理内存。
// C语言动态内存分配
#include <stdio.h>
#include <stdlib.h>
int main() {
int *arr;
int n = 5;
arr = (int *)malloc(n * sizeof(int));
if (arr == NULL) {
printf("Memory allocation failed\n");
return 1;
}
for (int i = 0; i < n; i++) {
arr[i] = i + 1;
}
for (int i = 0; i < n; i++) {
printf("%d ", arr[i]);
}
free(arr);
return 0;
}
# Python动态内存分配
n = 5
arr = [i + 1 for i in range(n)]
print(arr)
错误处理
C语言中的错误处理通常使用返回值和全局变量(如errno)来实现,而在Python中,错误处理通常使用异常机制(try-except语句)。
// C语言错误处理
#include <stdio.h>
#include <errno.h>
#include <string.h>
int main() {
FILE *fp;
fp = fopen("nonexistent.txt", "r");
if (fp == NULL) {
printf("Error opening file: %s\n", strerror(errno));
return 1;
}
fclose(fp);
return 0;
}
# Python错误处理
try:
with open("nonexistent.txt", "r") as fp:
pass
except FileNotFoundError as e:
print(f"Error opening file: {e}")
四、逐步重写代码
理解C代码,分析数据结构和算法,找到Python等效的语法和库函数后,可以开始逐步重写代码。建议逐行转换,并在每个步骤后进行测试,确保功能正确。
示例:将完整的C程序转换为Python
以下是一个完整的C程序示例,以及其对应的Python转换:
C程序
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node *next;
};
void append(struct Node head_ref, int new_data) {
struct Node *new_node = (struct Node *)malloc(sizeof(struct Node));
struct Node *last = *head_ref;
new_node->data = new_data;
new_node->next = NULL;
if (*head_ref == NULL) {
*head_ref = new_node;
return;
}
while (last->next != NULL) {
last = last->next;
}
last->next = new_node;
}
void printList(struct Node *node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
}
int main() {
struct Node *head = NULL;
append(&head, 1);
append(&head, 2);
append(&head, 3);
printf("Created linked list is: ");
printList(head);
return 0;
}
Python程序
class Node:
def __init__(self, data):
self.data = data
self.next = None
def append(head, new_data):
new_node = Node(new_data)
if head is None:
return new_node
last = head
while last.next:
last = last.next
last.next = new_node
return head
def print_list(node):
while node:
print(node.data, end=" ")
node = node.next
if __name__ == "__main__":
head = None
head = append(head, 1)
head = append(head, 2)
head = append(head, 3)
print("Created linked list is: ", end="")
print_list(head)
通过上述步骤,可以将C语言代码逐步转换为Python代码。需要注意的是,根据具体情况,可能需要对代码进行调试和优化。Python和C语言在语法和特性上存在一些差异,因此在转换过程中可能需要进行一些调整,以确保功能正确和性能优化。
相关问答FAQs:
如何将C语言的代码结构适应Python的编程风格?
在将C语言代码转换为Python时,重要的是理解两者的编程风格差异。C语言是一种静态类型语言,通常需要显式声明变量类型,而Python是动态类型语言,变量类型可以在运行时决定。为了适应Python的风格,建议使用简洁的命名,并利用Python的内置数据结构,如列表和字典,来替代C语言中的数组和结构体。此外,Python的代码通常不需要分号结束行,且缩进用于表示代码块,这意味着需要调整代码的格式。
在转换过程中,如何处理C语言中的指针和内存管理?
C语言中的指针和手动内存管理在Python中并不直接对应。Python使用垃圾回收机制来自动管理内存,避免了手动分配和释放内存的复杂性。因此,在转换时,应关注数据的引用而不是内存地址。例如,C语言中通过指针传递的数组可以直接转换为Python的列表,这样可以简化代码并提高可读性。在处理复杂数据结构时,可以考虑使用类来封装数据和方法。
有哪些工具或库可以帮助将C语言代码转换为Python?
虽然手动转换C语言到Python是一个常见的做法,但也有一些工具可以帮助简化这一过程。例如,Cython是一个可以将C代码与Python结合的工具,允许将C代码编译为Python扩展模块。此外,SWIG(Simplified Wrapper and Interface Generator)可以生成接口代码,以便将C或C++代码集成到Python中。使用这些工具可以减少手动转换的工作量,同时保持代码的性能和效率。
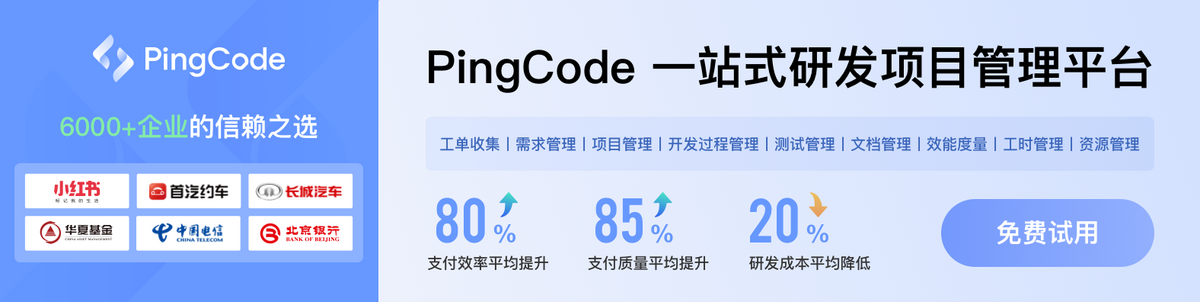