要使用Python通过FTP下载文件夹,可以使用以下方法:使用ftplib库、遍历文件夹、递归下载文件、处理连接问题。我们将详细描述如何通过这几种方法实现这一目的。
一、使用ftplib库
Python的标准库中包含一个名为ftplib的模块,可以用于FTP操作。首先,我们需要连接到FTP服务器并登录。以下是基本的连接和登录代码:
from ftplib import FTP
ftp = FTP('ftp.yourserver.com')
ftp.login(user='yourusername', passwd='yourpassword')
在连接和登录后,我们可以开始处理文件和文件夹的下载。ftplib库提供了一些基本的方法,比如nlst()
列出目录内容,retrbinary()
下载文件等等。下面我们会详细介绍如何使用这些方法。
二、遍历文件夹
我们需要一种方法来遍历FTP服务器上的文件夹,以便找到所有文件和子文件夹。以下是一个基本的示例代码,用于遍历目录内容并下载文件:
import os
from ftplib import FTP
def download_file(ftp, remote_file, local_file):
with open(local_file, 'wb') as f:
ftp.retrbinary(f'RETR {remote_file}', f.write)
def download_directory(ftp, remote_dir, local_dir):
if not os.path.exists(local_dir):
os.makedirs(local_dir)
ftp.cwd(remote_dir)
items = ftp.nlst()
for item in items:
if is_directory(ftp, item):
download_directory(ftp, item, os.path.join(local_dir, item))
else:
download_file(ftp, item, os.path.join(local_dir, item))
ftp.cwd('..')
def is_directory(ftp, name):
current = ftp.pwd()
try:
ftp.cwd(name)
ftp.cwd(current)
return True
except Exception as e:
return False
ftp = FTP('ftp.yourserver.com')
ftp.login(user='yourusername', passwd='yourpassword')
download_directory(ftp, '/remote/directory/path', 'local/directory/path')
ftp.quit()
三、递归下载文件
在上面的代码中,download_directory
函数通过递归调用自身来处理子目录中的内容。这个方法有效地处理了多层嵌套的目录结构。在download_file
函数中,我们使用retrbinary
方法下载文件。
四、处理连接问题
在实际操作中,FTP连接可能会出现超时或其他连接问题。我们可以使用try-except块来处理这些问题,并在必要时重新连接:
import time
from ftplib import FTP, error_perm
def connect_ftp(host, user, passwd):
ftp = FTP(host)
ftp.login(user=user, passwd=passwd)
return ftp
def download_file(ftp, remote_file, local_file):
with open(local_file, 'wb') as f:
ftp.retrbinary(f'RETR {remote_file}', f.write)
def download_directory(ftp, remote_dir, local_dir):
if not os.path.exists(local_dir):
os.makedirs(local_dir)
ftp.cwd(remote_dir)
items = ftp.nlst()
for item in items:
if is_directory(ftp, item):
download_directory(ftp, item, os.path.join(local_dir, item))
else:
download_file(ftp, item, os.path.join(local_dir, item))
ftp.cwd('..')
def is_directory(ftp, name):
current = ftp.pwd()
try:
ftp.cwd(name)
ftp.cwd(current)
return True
except error_perm:
return False
def main():
host = 'ftp.yourserver.com'
user = 'yourusername'
passwd = 'yourpassword'
remote_dir = '/remote/directory/path'
local_dir = 'local/directory/path'
ftp = connect_ftp(host, user, passwd)
try:
download_directory(ftp, remote_dir, local_dir)
except Exception as e:
print(f'Error: {e}')
finally:
ftp.quit()
if __name__ == '__main__':
main()
五、优化代码
为了提高代码的可读性和可维护性,我们可以将一些重复的代码进行封装。比如,可以将FTP连接部分单独封装成一个函数,便于在需要时重新连接:
def connect_ftp(host, user, passwd):
ftp = FTP(host)
ftp.login(user=user, passwd=passwd)
return ftp
def download_file(ftp, remote_file, local_file):
with open(local_file, 'wb') as f:
ftp.retrbinary(f'RETR {remote_file}', f.write)
def download_directory(ftp, remote_dir, local_dir):
if not os.path.exists(local_dir):
os.makedirs(local_dir)
ftp.cwd(remote_dir)
items = ftp.nlst()
for item in items:
if is_directory(ftp, item):
download_directory(ftp, item, os.path.join(local_dir, item))
else:
download_file(ftp, item, os.path.join(local_dir, item))
ftp.cwd('..')
def is_directory(ftp, name):
current = ftp.pwd()
try:
ftp.cwd(name)
ftp.cwd(current)
return True
except error_perm:
return False
def main():
host = 'ftp.yourserver.com'
user = 'yourusername'
passwd = 'yourpassword'
remote_dir = '/remote/directory/path'
local_dir = 'local/directory/path'
ftp = connect_ftp(host, user, passwd)
try:
download_directory(ftp, remote_dir, local_dir)
except Exception as e:
print(f'Error: {e}')
finally:
ftp.quit()
if __name__ == '__main__':
main()
六、使用第三方库
除了ftplib库外,Python还提供了一些第三方库,如paramiko(用于SFTP)和pyftpdlib(用于FTP服务器)。这些库可以提供更多的功能和更高的灵活性。
例如,使用paramiko可以实现SFTP下载:
import paramiko
import os
def download_file(sftp, remote_file, local_file):
sftp.get(remote_file, local_file)
def download_directory(sftp, remote_dir, local_dir):
if not os.path.exists(local_dir):
os.makedirs(local_dir)
for item in sftp.listdir_attr(remote_dir):
remote_path = os.path.join(remote_dir, item.filename)
local_path = os.path.join(local_dir, item.filename)
if item.st_mode & 0o040000: # 判断是否为目录
download_directory(sftp, remote_path, local_path)
else:
download_file(sftp, remote_path, local_path)
def main():
host = 'sftp.yourserver.com'
port = 22
user = 'yourusername'
passwd = 'yourpassword'
remote_dir = '/remote/directory/path'
local_dir = 'local/directory/path'
transport = paramiko.Transport((host, port))
transport.connect(username=user, password=passwd)
sftp = paramiko.SFTPClient.from_transport(transport)
try:
download_directory(sftp, remote_dir, local_dir)
except Exception as e:
print(f'Error: {e}')
finally:
sftp.close()
transport.close()
if __name__ == '__main__':
main()
七、总结
通过本文,我们介绍了如何使用Python通过FTP下载文件夹的方法。我们详细描述了使用ftplib库、遍历文件夹、递归下载文件、处理连接问题等内容。我们还提到了一些优化代码的方法和使用第三方库的替代方案。希望这些内容能帮助你更好地理解和实现Python的FTP下载操作。
相关问答FAQs:
如何使用Python实现FTP文件夹的下载?
在Python中,可以使用ftplib
库来连接FTP服务器,并通过递归的方法来下载整个文件夹。首先需要连接到FTP服务器,然后列出目录内容,逐一下载文件,并对每个子文件夹执行相同的操作。这样可以确保整个文件夹及其子文件夹中的所有文件都被下载。
需要安装额外的库吗?
在标准库中,ftplib
已经包含在内,因此不需要额外安装任何库。不过,如果您希望处理更复杂的FTP操作,例如支持多线程下载,您可能会考虑使用asyncio
或第三方库如pyftpdlib
等。
如何处理FTP连接的异常情况?
在连接FTP服务器时,网络问题或服务器响应时间过长可能会导致异常。可以使用try-except
块来捕获这些异常,并相应地处理,例如重试连接或记录错误信息。此外,确保在下载过程中验证文件的完整性,以避免因网络问题导致的文件损坏。
下载完成后如何验证文件?
下载文件夹后,可以通过比较文件大小和校验和(例如MD5或SHA256)来验证下载的文件是否完整。使用Python的hashlib
库计算文件的哈希值,并与服务器上文件的哈希值进行比较,可以确保文件下载无误。这种方式能够有效避免数据损坏或丢失的问题。
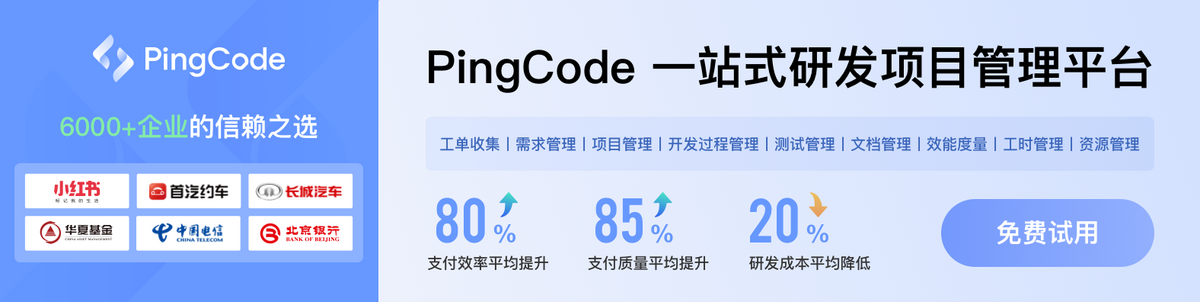