Python写入保存文件夹的方法包含使用适当的文件路径、创建文件夹、写入文件、保存文件等步骤。首先确保路径存在、使用 os 和 open 函数进行操作、处理异常等。 其中一个核心点是确保目标文件夹存在,若不存在则创建。下面将详细描述这些步骤。
一、确保路径存在
在编写Python代码时,首先要确保目标文件夹路径存在。如果路径不存在,需要创建路径。可以使用 os
模块中的 os.path.exists()
和 os.makedirs()
方法来检查和创建路径。
import os
def ensure_dir(directory):
if not os.path.exists(directory):
os.makedirs(directory)
二、写入文件
使用 open()
方法打开文件,然后使用 write()
方法将内容写入文件。要确保文件写入时的路径是正确的。
def write_to_file(file_path, content):
with open(file_path, 'w') as file:
file.write(content)
三、完整示例
结合以上两个步骤,可以写一个完整的示例,确保路径存在,然后将内容写入文件。
import os
def ensure_dir(directory):
if not os.path.exists(directory):
os.makedirs(directory)
def write_to_file(file_path, content):
ensure_dir(os.path.dirname(file_path))
with open(file_path, 'w') as file:
file.write(content)
directory_path = 'example_dir/sub_dir'
file_name = 'example.txt'
file_path = os.path.join(directory_path, file_name)
content = 'Hello, World!'
write_to_file(file_path, content)
四、处理异常
在实际应用中,处理异常是很重要的。使用 try-except
块来捕获和处理可能的异常。
import os
def ensure_dir(directory):
try:
if not os.path.exists(directory):
os.makedirs(directory)
except OSError as e:
print(f"Error creating directory {directory}: {e}")
def write_to_file(file_path, content):
try:
ensure_dir(os.path.dirname(file_path))
with open(file_path, 'w') as file:
file.write(content)
except IOError as e:
print(f"Error writing to file {file_path}: {e}")
directory_path = 'example_dir/sub_dir'
file_name = 'example.txt'
file_path = os.path.join(directory_path, file_name)
content = 'Hello, World!'
write_to_file(file_path, content)
五、其他实用技巧
1、追加写入
使用 open()
方法的第二个参数 'a'
可以实现追加写入。
def append_to_file(file_path, content):
ensure_dir(os.path.dirname(file_path))
with open(file_path, 'a') as file:
file.write(content)
2、处理二进制文件
对于二进制文件,例如图片,可以使用 'wb'
模式。
def write_binary_file(file_path, data):
ensure_dir(os.path.dirname(file_path))
with open(file_path, 'wb') as file:
file.write(data)
3、读取文件内容
使用 open()
和 read()
方法读取文件内容。
def read_file(file_path):
with open(file_path, 'r') as file:
return file.read()
六、实际应用案例
1、日志记录
在开发过程中,记录日志是非常重要的。可以将日志写入文件,以便后续查看和分析。
import datetime
def log_message(file_path, message):
timestamp = datetime.datetime.now().strftime('%Y-%m-%d %H:%M:%S')
log_entry = f"{timestamp} - {message}\n"
append_to_file(file_path, log_entry)
log_file = 'logs/app.log'
log_message(log_file, 'Application started')
log_message(log_file, 'An error occurred')
2、配置文件
配置文件通常用于存储应用程序的配置参数。可以将配置参数写入文件,并在应用程序启动时读取配置文件。
import json
def write_config(file_path, config):
ensure_dir(os.path.dirname(file_path))
with open(file_path, 'w') as file:
json.dump(config, file, indent=4)
def read_config(file_path):
with open(file_path, 'r') as file:
return json.load(file)
config = {
'host': 'localhost',
'port': 8080,
'debug': True
}
config_file = 'config/app_config.json'
write_config(config_file, config)
loaded_config = read_config(config_file)
print(loaded_config)
七、文件操作的安全性
在处理文件操作时,安全性是一个重要的考虑因素。确保文件路径的安全性和文件内容的完整性是关键。
1、路径验证
验证文件路径,以防止路径遍历攻击。可以使用 os.path.abspath()
方法获取绝对路径,并检查路径是否在预期的目录中。
def validate_path(file_path, base_dir):
absolute_path = os.path.abspath(file_path)
if not absolute_path.startswith(os.path.abspath(base_dir)):
raise ValueError(f"Invalid file path: {file_path}")
return absolute_path
base_dir = 'example_dir'
file_path = validate_path('example_dir/sub_dir/example.txt', base_dir)
2、文件内容验证
验证文件内容的完整性,例如计算文件的哈希值,以确保文件未被篡改。
import hashlib
def compute_file_hash(file_path):
hash_algo = hashlib.sha256()
with open(file_path, 'rb') as file:
while chunk := file.read(8192):
hash_algo.update(chunk)
return hash_algo.hexdigest()
file_hash = compute_file_hash(file_path)
print(f"File hash: {file_hash}")
八、总结
本文详细介绍了Python写入保存文件夹的方法,涵盖了确保路径存在、写入文件、处理异常、追加写入、处理二进制文件、读取文件内容等多方面内容。通过多个实际应用案例,如日志记录、配置文件操作等,展示了文件操作在实际开发中的应用。同时,强调了文件操作的安全性,提供了路径验证和文件内容验证的方法。
通过本文的学习,相信读者能够掌握Python写入保存文件夹的基本方法和技巧,并能够在实际开发中灵活应用这些知识,提高代码的健壮性和安全性。
相关问答FAQs:
如何在Python中创建一个新文件夹?
在Python中,可以使用os
模块中的mkdir()
函数来创建新文件夹。只需导入os
模块,然后调用os.mkdir('文件夹路径')
即可。例如,如果要在当前目录下创建一个名为my_folder
的文件夹,可以这样写:
import os
os.mkdir('my_folder')
确保提供的路径是有效的,并且没有同名文件夹存在。
Python如何将文件写入特定的文件夹?
要将文件写入特定的文件夹,您需要先确保该文件夹存在,然后使用open()
函数指定文件的完整路径。例如,如果要在my_folder
中创建一个名为example.txt
的文件,可以使用如下代码:
with open('my_folder/example.txt', 'w') as file:
file.write('Hello, World!')
这段代码将在my_folder
中创建example.txt
文件,并写入Hello, World!
这段文本。
如何检查一个文件夹是否存在,避免重复创建?
在创建文件夹之前,您可以使用os.path.exists()
来检查文件夹是否已经存在。如果不存在,才进行创建。示例代码如下:
import os
folder_name = 'my_folder'
if not os.path.exists(folder_name):
os.mkdir(folder_name)
通过这种方式,可以有效避免因重复创建而导致的错误。
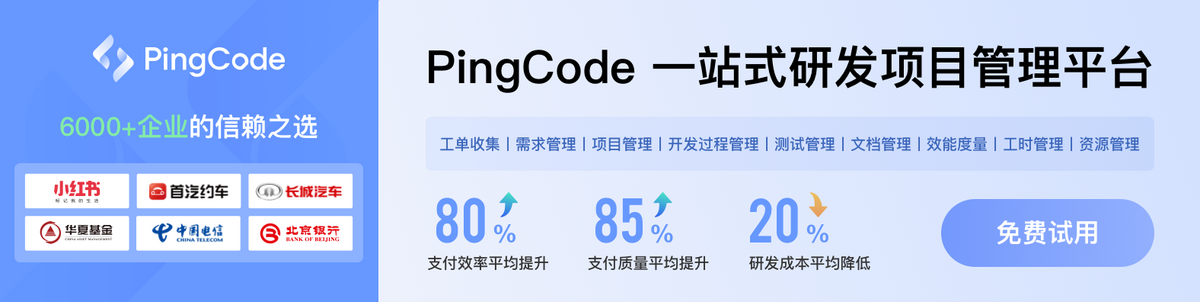