在Python中,删除字符串中的子串有多种方法,例如使用字符串的replace()方法、字符串切片、正则表达式等。replace()方法、字符串切片、正则表达式是三种常见的方式,下面将详细展开介绍这三种方法,并讲解如何使用它们来删去字符串中的子串。
一、使用replace()方法
replace()方法是Python中字符串对象的一个内建方法,它可以用来将字符串中的某些子串替换为另一个子串。如果将目标子串替换为空字符串,就达到了删除该子串的目的。
示例代码:
original_string = "Hello, this is a sample string."
substring_to_remove = "sample"
new_string = original_string.replace(substring_to_remove, "")
print(new_string) # 输出: Hello, this is a string.
详细解析:
replace()方法的第一个参数是需要被替换的子串,第二个参数是用来替换的子串。将第二个参数设置为空字符串,就可以删除目标子串。
二、使用字符串切片
字符串切片是Python中处理字符串的一个强大工具。通过计算子串在字符串中的位置,可以使用切片操作将子串删除。
示例代码:
original_string = "Hello, this is a sample string."
substring_to_remove = "sample"
start_index = original_string.find(substring_to_remove)
if start_index != -1:
end_index = start_index + len(substring_to_remove)
new_string = original_string[:start_index] + original_string[end_index:]
else:
new_string = original_string
print(new_string) # 输出: Hello, this is a string.
详细解析:
- 使用find()方法找到子串的起始位置。
- 计算子串的结束位置。
- 使用字符串切片将子串前后的部分连接起来,达到删除子串的效果。
三、使用正则表达式
正则表达式是处理字符串的强大工具,可以用来匹配、替换和删除字符串中的特定模式。在Python中,可以使用re模块来进行正则表达式操作。
示例代码:
import re
original_string = "Hello, this is a sample string."
substring_to_remove = "sample"
new_string = re.sub(substring_to_remove, "", original_string)
print(new_string) # 输出: Hello, this is a string.
详细解析:
- 导入re模块。
- 使用re.sub()方法将目标子串替换为空字符串,从而删除子串。
四、总结与扩展
总结:
replace()方法是最简单直接的方法,适合处理简单的字符串替换;字符串切片适合在知道子串位置的情况下使用;正则表达式则适合处理复杂的字符串模式匹配和替换。
扩展:
- 多次删除:如果需要删除多个子串,可以在一个循环中多次调用上述方法。
- 忽略大小写:在使用正则表达式时,可以添加re.IGNORECASE标志来忽略大小写。
- 删除多个不同子串:可以将多个子串放在一个列表中,循环遍历列表并依次删除每个子串。
示例代码(扩展):
import re
original_string = "Hello, this is a sample string. Sample strings are common."
substrings_to_remove = ["sample", "Sample"]
使用replace()方法
new_string = original_string
for substring in substrings_to_remove:
new_string = new_string.replace(substring, "")
print(new_string) # 输出: Hello, this is a string. strings are common.
使用正则表达式,忽略大小写
pattern = "|".join(substrings_to_remove) # 构建正则表达式模式
new_string = re.sub(pattern, "", original_string, flags=re.IGNORECASE)
print(new_string) # 输出: Hello, this is a string. strings are common.
通过以上方法,可以灵活地删除字符串中的子串,满足不同场景下的需求。这些方法不仅适用于简单的字符串操作,也可以在复杂的文本处理任务中发挥作用。希望这些方法和示例代码能对你有所帮助。
相关问答FAQs:
如何在Python中安全地删除字符串中的子串?
在Python中,您可以使用str.replace()
方法来删除子串。该方法允许您将目标子串替换为空字符串,从而达到删除的效果。例如,original_string.replace("substring", "")
会删除所有出现的“substring”。这种方法简单易用,但请注意它会删除所有匹配的子串。
是否可以使用正则表达式来删除字符串中的子串?
确实可以,使用Python的re
模块可以通过正则表达式删除字符串中的子串。例如,您可以使用re.sub()
方法,像这样:re.sub("substring", "", original_string)
。这种方法提供了更强大的模式匹配功能,适合更复杂的删除需求。
在删除字符串子串时,是否有性能方面的考虑?
当处理非常大的字符串或大量子串时,性能可能会成为一个问题。str.replace()
方法通常效率较高,但如果需要删除的子串非常多,使用正则表达式可能会导致性能下降。在这种情况下,考虑使用其他方法,如列表推导式或字符串拼接,来实现更高效的操作。
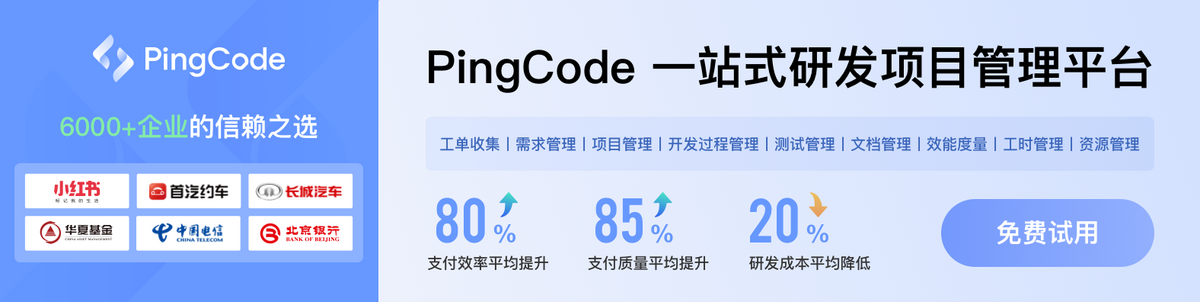