使用Python中的字典加密文字,核心步骤包括:创建加密字典、加密过程、解密过程。其中,创建加密字典是最关键的一步,因为它决定了加密和解密的映射关系。
在Python中,字典(dictionary)是一个非常强大的数据结构,能够以键值对的形式存储数据。我们可以利用字典来创建一个加密映射,从而实现文字的加密和解密。为了更好地理解这个过程,我们可以先创建一个简单的加密字典,然后使用这个字典将文字加密,并最终通过解密字典恢复原文。
一、创建加密字典
首先,我们需要创建一个加密字典。这个字典将每个字母映射到另一个字母或符号。例如,我们可以创建一个简单的加密字典,将每个字母替换为其后的字母:
import string
def create_encryption_dict():
letters = string.ascii_lowercase
encryption_dict = {letters[i]: letters[(i + 1) % len(letters)] for i in range(len(letters))}
return encryption_dict
encryption_dict = create_encryption_dict()
print(encryption_dict)
在这个例子中,我们使用Python的内置模块string
来获取所有小写字母,然后创建一个字典,将每个字母映射到其后的字母。这样,'a'会映射到'b','b'会映射到'c',以此类推,最后一个字母'z'映射回'a'。
二、加密过程
有了加密字典后,我们可以使用它来加密文字。我们遍历原文字中的每个字符,并使用加密字典进行替换:
def encrypt_text(text, encryption_dict):
encrypted_text = ''.join(encryption_dict.get(char, char) for char in text)
return encrypted_text
original_text = "hello"
encrypted_text = encrypt_text(original_text, encryption_dict)
print(f"Original: {original_text}")
print(f"Encrypted: {encrypted_text}")
在这个例子中,我们定义了一个encrypt_text
函数,用于加密文字。我们遍历原文字中的每个字符,并使用加密字典进行替换。如果字符在字典中找不到对应的加密字符,我们保留原字符不变。
三、解密过程
为了能够恢复加密前的原文,我们还需要创建一个解密字典。解密字典是加密字典的反向映射:
def create_decryption_dict(encryption_dict):
decryption_dict = {v: k for k, v in encryption_dict.items()}
return decryption_dict
decryption_dict = create_decryption_dict(encryption_dict)
print(decryption_dict)
有了解密字典后,我们可以使用它来解密文字:
def decrypt_text(text, decryption_dict):
decrypted_text = ''.join(decryption_dict.get(char, char) for char in text)
return decrypted_text
decrypted_text = decrypt_text(encrypted_text, decryption_dict)
print(f"Encrypted: {encrypted_text}")
print(f"Decrypted: {decrypted_text}")
在这个例子中,我们定义了一个decrypt_text
函数,用于解密文字。我们遍历加密文字中的每个字符,并使用解密字典进行替换。如果字符在字典中找不到对应的解密字符,我们保留原字符不变。
四、拓展加密字典
我们可以进一步扩展加密字典,使其能够处理大小写字母、数字和其他符号。以下是一个更复杂的加密字典示例:
def create_complex_encryption_dict():
characters = string.ascii_letters + string.digits + string.punctuation
shuffled_characters = ''.join(random.sample(characters, len(characters)))
encryption_dict = {characters[i]: shuffled_characters[i] for i in range(len(characters))}
return encryption_dict
complex_encryption_dict = create_complex_encryption_dict()
print(complex_encryption_dict)
在这个例子中,我们使用random.sample
函数随机打乱所有字符,然后创建一个更复杂的加密字典。
五、使用复杂加密字典加密和解密
有了复杂的加密字典后,我们可以使用与之前相同的方法对文字进行加密和解密:
complex_encrypted_text = encrypt_text(original_text, complex_encryption_dict)
print(f"Original: {original_text}")
print(f"Complex Encrypted: {complex_encrypted_text}")
complex_decryption_dict = create_decryption_dict(complex_encryption_dict)
complex_decrypted_text = decrypt_text(complex_encrypted_text, complex_decryption_dict)
print(f"Complex Encrypted: {complex_encrypted_text}")
print(f"Complex Decrypted: {complex_decrypted_text}")
六、处理空格和特殊字符
在实际应用中,我们还需要处理空格和其他特殊字符。我们可以将这些字符保留在原文中,不进行加密:
def encrypt_text_with_spaces(text, encryption_dict):
encrypted_text = ''.join(encryption_dict.get(char, char) for char in text if char in encryption_dict or char.isspace())
return encrypted_text
def decrypt_text_with_spaces(text, decryption_dict):
decrypted_text = ''.join(decryption_dict.get(char, char) for char in text if char in decryption_dict or char.isspace())
return decrypted_text
original_text_with_spaces = "hello world!"
encrypted_text_with_spaces = encrypt_text_with_spaces(original_text_with_spaces, complex_encryption_dict)
print(f"Original: {original_text_with_spaces}")
print(f"Encrypted with spaces: {encrypted_text_with_spaces}")
decrypted_text_with_spaces = decrypt_text_with_spaces(encrypted_text_with_spaces, complex_decryption_dict)
print(f"Encrypted with spaces: {encrypted_text_with_spaces}")
print(f"Decrypted with spaces: {decrypted_text_with_spaces}")
在这个例子中,我们在加密和解密过程中检查字符是否为空格,以确保空格保留在原文中。
七、总结
通过以上步骤,我们可以使用Python中的字典实现文字的加密和解密。创建加密字典是最关键的一步,它决定了加密和解密的映射关系。我们可以根据需要创建简单或复杂的加密字典,并根据具体情况处理空格和特殊字符。希望本文能够帮助您更好地理解和应用Python中的字典加密文字。
相关问答FAQs:
如何使用Python字典来加密文本?
在Python中,可以通过创建一个映射字典,将每个字母或字符替换为对应的加密字符。这种方法简单且易于实现。可以使用str.translate()
方法结合str.maketrans()
来实现字典加密,首先定义一个字典,然后生成一个转换表,最后对文本进行加密。
字典加密的效果如何?
字典加密的效果依赖于字典的设计,若字典包含所有可能的字符映射,且映射关系不明显,则加密效果较好。用户可以自定义字典的内容,如使用随机字符、符号或数字来替代原字符,这样可以增加破解的难度。
如何解密使用字典加密的文本?
解密过程与加密相似,只需创建一个反向字典,将加密字符映射回原字符。可以通过将原字典的键和值互换,构建反向字典,然后使用相同的str.translate()
方法对加密文本进行解密。这种方法确保用户可以轻松恢复原文。
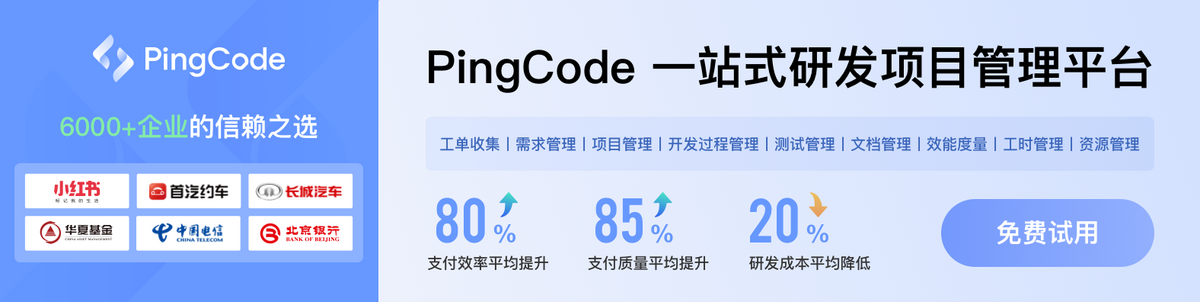