在Python中,可以使用多种方法下载图片,包括使用标准库和第三方库。下面是一些常见的方法和步骤:
一、使用requests库下载图片
import requests
def download_image(image_url, save_path):
response = requests.get(image_url)
if response.status_code == 200:
with open(save_path, 'wb') as file:
file.write(response.content)
print(f"Image successfully downloaded: {save_path}")
else:
print(f"Failed to download image. Status code: {response.status_code}")
示例使用
image_url = 'https://example.com/image.jpg'
save_path = 'path/to/save/image.jpg'
download_image(image_url, save_path)
二、使用urllib库下载图片
import urllib.request
def download_image(image_url, save_path):
try:
urllib.request.urlretrieve(image_url, save_path)
print(f"Image successfully downloaded: {save_path}")
except Exception as e:
print(f"Failed to download image. Error: {e}")
示例使用
image_url = 'https://example.com/image.jpg'
save_path = 'path/to/save/image.jpg'
download_image(image_url, save_path)
三、使用BeautifulSoup和requests组合下载网页中的所有图片
import requests
from bs4 import BeautifulSoup
import os
def download_all_images(url, save_folder):
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
img_tags = soup.find_all('img')
if not os.path.exists(save_folder):
os.makedirs(save_folder)
for img in img_tags:
img_url = img.get('src')
if not img_url:
continue
if not img_url.startswith('http'):
img_url = url + img_url
img_name = os.path.join(save_folder, os.path.basename(img_url))
try:
with open(img_name, 'wb') as f:
f.write(requests.get(img_url).content)
print(f"Downloaded: {img_name}")
except Exception as e:
print(f"Could not download {img_url}. Error: {e}")
示例使用
url = 'https://example.com'
save_folder = 'images'
download_all_images(url, save_folder)
四、使用asyncio和aiohttp实现异步下载图片
import aiohttp
import asyncio
import os
async def download_image(session, url, save_path):
async with session.get(url) as response:
if response.status == 200:
with open(save_path, 'wb') as f:
f.write(await response.read())
print(f"Image successfully downloaded: {save_path}")
else:
print(f"Failed to download image. Status code: {response.status}")
async def main(urls, save_folder):
async with aiohttp.ClientSession() as session:
tasks = []
for url in urls:
save_path = os.path.join(save_folder, os.path.basename(url))
tasks.append(download_image(session, url, save_path))
await asyncio.gather(*tasks)
示例使用
image_urls = [
'https://example.com/image1.jpg',
'https://example.com/image2.jpg'
]
save_folder = 'images'
if not os.path.exists(save_folder):
os.makedirs(save_folder)
asyncio.run(main(image_urls, save_folder))
五、使用多线程下载图片
import requests
from concurrent.futures import ThreadPoolExecutor
import os
def download_image(url, save_path):
response = requests.get(url)
if response.status_code == 200:
with open(save_path, 'wb') as file:
file.write(response.content)
print(f"Image successfully downloaded: {save_path}")
else:
print(f"Failed to download image. Status code: {response.status_code}")
def main(urls, save_folder):
if not os.path.exists(save_folder):
os.makedirs(save_folder)
with ThreadPoolExecutor(max_workers=5) as executor:
for url in urls:
save_path = os.path.join(save_folder, os.path.basename(url))
executor.submit(download_image, url, save_path)
示例使用
image_urls = [
'https://example.com/image1.jpg',
'https://example.com/image2.jpg'
]
save_folder = 'images'
main(image_urls, save_folder)
总结
下载图片的Python脚本可以通过多种方式实现,包括使用requests、urllib、BeautifulSoup、aiohttp和多线程等方法。每种方法都有其优点和适用场景,例如requests库简单易用,适合下载单个或少量图片;BeautifulSoup可以方便地解析网页,适合从网页中批量下载图片;aiohttp和多线程则适用于需要高效并发下载大量图片的场景。根据具体需求选择合适的方法,可以有效提升下载效率和代码的可维护性。
相关问答FAQs:
如何使用Python脚本下载特定网址的图片?
要下载特定网址的图片,您可以使用Python中的requests库获取网页内容,并结合BeautifulSoup解析HTML,提取出图片的URL。接着,使用requests库再次下载这些图片。以下是一个简单的示例代码:
import requests
from bs4 import BeautifulSoup
import os
url = 'http://example.com' # 替换为您的目标网址
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
# 创建一个目录来存储下载的图片
os.makedirs('downloaded_images', exist_ok=True)
for img in soup.find_all('img'):
img_url = img.get('src')
if img_url.startswith('http'): # 确保是完整的URL
img_response = requests.get(img_url)
img_name = os.path.join('downloaded_images', img_url.split('/')[-1])
with open(img_name, 'wb') as f:
f.write(img_response.content)
在下载图片时需要注意哪些问题?
在下载图片时,确保遵循网站的使用条款和版权声明。某些网站可能会限制或禁止图片下载。使用爬虫技术时,建议添加适当的延迟,避免对目标网站造成过大负担。此外,检查图片URL是否以HTTP或HTTPS开头,以确保能正确访问。
有哪些Python库可以帮助我下载图片?
除了requests和BeautifulSoup,您还可以使用其他库,如Pillow(PIL)用于处理和保存图像,Scrapy用于更复杂的网站抓取和数据提取,以及wget库提供简化的文件下载功能。这些工具可以根据不同需求,帮助您更高效地下载和处理图片。
如何处理下载过程中可能出现的错误?
在下载图片时,可能会遇到网络问题、权限错误或文件写入错误等。可以通过try-except语句捕获异常,并进行相应的处理。例如,您可以记录错误日志、重试下载或跳过当前文件。确保在下载前检查URL的有效性,可以显著减少错误的发生。
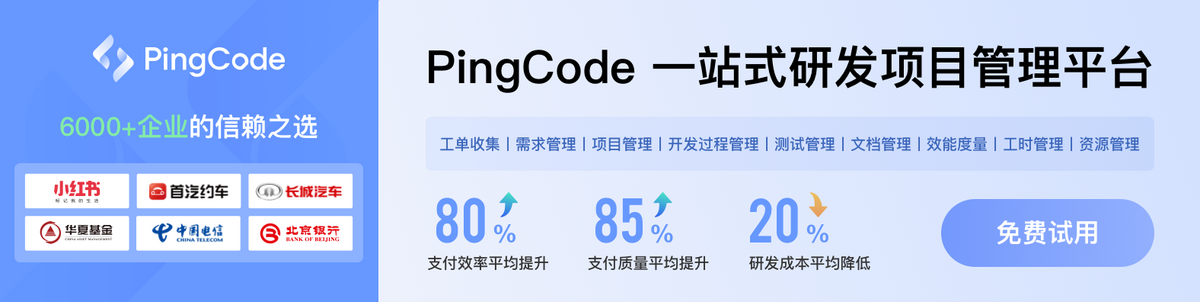