在Python中调用C语言代码的方法有多种:使用ctypes、使用cffi、编写Python扩展模块、使用SWIG。其中,使用ctypes和cffi是最常用的方法。ctypes模块是Python标准库的一部分,允许调用动态链接库(DLL)或共享对象(SO)中的函数,cffi(C Foreign Function Interface)是一个用于调用C代码的Python库,提供了更高级的接口。下面将详细介绍如何使用这两种方法调用C语言代码。
一、使用ctypes模块
1、准备C语言代码
首先,需要编写一个简单的C语言代码,并将其编译为动态库。假设我们有一个简单的C语言代码文件example.c
,内容如下:
// example.c
#include <stdio.h>
void hello() {
printf("Hello from C!\n");
}
int add(int a, int b) {
return a + b;
}
编译上述代码生成动态库(在Linux上生成.so
文件,在Windows上生成.dll
文件,在macOS上生成.dylib
文件):
gcc -shared -o example.so -fPIC example.c
2、使用ctypes加载和调用C函数
在Python中使用ctypes
模块加载动态库并调用C函数:
import ctypes
加载动态库
example = ctypes.CDLL('./example.so')
调用hello函数
example.hello()
调用add函数
result = example.add(2, 3)
print("Result of add:", result)
3、处理复杂的数据类型
如果C函数使用了复杂的数据类型(如结构体、指针),我们需要在Python中定义相应的数据类型。例如:
// complex_example.c
#include <stdio.h>
typedef struct {
int x;
int y;
} Point;
void print_point(Point p) {
printf("Point: (%d, %d)\n", p.x, p.y);
}
Point create_point(int x, int y) {
Point p = {x, y};
return p;
}
编译上述代码生成动态库:
gcc -shared -o complex_example.so -fPIC complex_example.c
在Python中使用ctypes
加载并调用这些函数:
import ctypes
定义结构体
class Point(ctypes.Structure):
_fields_ = [("x", ctypes.c_int),
("y", ctypes.c_int)]
加载动态库
example = ctypes.CDLL('./complex_example.so')
定义返回类型和参数类型
example.create_point.restype = Point
example.create_point.argtypes = [ctypes.c_int, ctypes.c_int]
example.print_point.argtypes = [Point]
调用函数
p = example.create_point(10, 20)
example.print_point(p)
二、使用cffi模块
1、准备C语言代码
与ctypes
方法相同,首先准备C语言代码并生成动态库。
2、使用cffi加载和调用C函数
在Python中使用cffi
模块加载动态库并调用C函数:
from cffi import FFI
ffi = FFI()
定义C函数接口
ffi.cdef("""
void hello();
int add(int a, int b);
""")
加载动态库
example = ffi.dlopen('./example.so')
调用函数
example.hello()
result = example.add(2, 3)
print("Result of add:", result)
3、处理复杂的数据类型
处理复杂的数据类型时,使用cffi
定义相应的数据类型。例如:
from cffi import FFI
ffi = FFI()
定义C函数接口和结构体
ffi.cdef("""
typedef struct {
int x;
int y;
} Point;
void print_point(Point p);
Point create_point(int x, int y);
""")
加载动态库
example = ffi.dlopen('./complex_example.so')
调用函数
p = example.create_point(10, 20)
example.print_point(p)
三、编写Python扩展模块
编写Python扩展模块是另一种调用C代码的方法。可以使用Cython或直接编写C代码来创建扩展模块。
1、使用Cython
Cython是一种编程语言,旨在使编写C扩展模块更加容易。首先安装Cython:
pip install cython
编写一个Cython文件example.pyx
:
# example.pyx
cdef extern from "example.h":
void hello()
int add(int a, int b)
def py_hello():
hello()
def py_add(int a, int b):
return add(a, b)
编写一个setup.py
文件来编译Cython代码:
from setuptools import setup
from Cython.Build import cythonize
setup(
ext_modules = cythonize("example.pyx")
)
运行setup.py
来编译Cython代码:
python setup.py build_ext --inplace
在Python中使用编译后的模块:
import example
example.py_hello()
result = example.py_add(2, 3)
print("Result of add:", result)
2、直接编写C扩展模块
直接编写C扩展模块需要编写一些C代码和Python接口。假设有一个C文件example.c
:
#include <Python.h>
static PyObject* py_hello(PyObject* self, PyObject* args) {
printf("Hello from C!\n");
Py_RETURN_NONE;
}
static PyObject* py_add(PyObject* self, PyObject* args) {
int a, b;
if (!PyArg_ParseTuple(args, "ii", &a, &b)) {
return NULL;
}
return Py_BuildValue("i", a + b);
}
static PyMethodDef ExampleMethods[] = {
{"hello", py_hello, METH_NOARGS, "Print hello from C."},
{"add", py_add, METH_VARARGS, "Add two integers."},
{NULL, NULL, 0, NULL}
};
static struct PyModuleDef examplemodule = {
PyModuleDef_HEAD_INIT,
"example",
NULL,
-1,
ExampleMethods
};
PyMODINIT_FUNC PyInit_example(void) {
return PyModule_Create(&examplemodule);
}
编写一个setup.py
文件来编译C代码:
from setuptools import setup, Extension
example_module = Extension('example',
sources=['example.c'])
setup(
ext_modules=[example_module]
)
运行setup.py
来编译C代码:
python setup.py build_ext --inplace
在Python中使用编译后的模块:
import example
example.hello()
result = example.add(2, 3)
print("Result of add:", result)
四、使用SWIG
SWIG(Simplified Wrapper and Interface Generator)是一种工具,可以自动生成调用C/C++代码的接口。使用SWIG可以简化生成Python扩展模块的过程。
1、准备C语言代码
假设有一个简单的C语言代码文件example.c
:
// example.c
#include <stdio.h>
void hello() {
printf("Hello from C!\n");
}
int add(int a, int b) {
return a + b;
}
编写一个接口文件example.i
:
/* example.i */
%module example
%{
#include "example.h"
%}
extern void hello();
extern int add(int a, int b);
2、使用SWIG生成包装代码
运行SWIG生成包装代码:
swig -python -o example_wrap.c example.i
编写一个setup.py
文件来编译生成的包装代码:
from setuptools import setup, Extension
example_module = Extension('example',
sources=['example_wrap.c', 'example.c'])
setup(
ext_modules=[example_module]
)
运行setup.py
来编译生成的包装代码:
python setup.py build_ext --inplace
在Python中使用编译后的模块:
import example
example.hello()
result = example.add(2, 3)
print("Result of add:", result)
通过上述步骤,可以在Python中成功调用C语言代码。无论是使用ctypes
、cffi
、编写Python扩展模块,还是使用SWIG,都能够实现这一目标。根据具体需求和个人偏好选择合适的方法。
相关问答FAQs:
如何在Python中有效地调用C语言代码以提高性能?
在Python中调用C语言代码可以通过多种方式实现,最常见的方法包括使用Cython、ctypes或者Python的扩展模块。Cython允许你将Python代码编译成C代码,从而提高执行速度。ctypes是一个内置库,可以直接加载C语言共享库,并调用其函数。对于需要复杂交互的情况,可以编写Python扩展模块,使用Python的API接口。
在Python中调用C语言代码时需要注意哪些事项?
在调用C语言代码时,确保数据类型的兼容性非常重要。例如,Python中的整数和C语言中的整型并不完全相同。此外,内存管理也需谨慎,C语言需要手动管理内存,而Python则有垃圾回收机制。因此,确保在C代码中正确分配和释放内存,以避免内存泄漏或崩溃。
有没有简单的示例代码可以展示如何在Python中调用C语言代码?
当然可以。假设你有一个简单的C函数,计算两个整数的和,可以将其编译为共享库。以下是一个简化的示例:
// mymodule.c
int add(int a, int b) {
return a + b;
}
编译为共享库后,使用ctypes调用:
import ctypes
# 加载共享库
mylib = ctypes.CDLL('./mymodule.so')
# 调用C函数
result = mylib.add(3, 5)
print(result) # 输出:8
这个示例展示了如何通过ctypes轻松调用C语言中的函数。
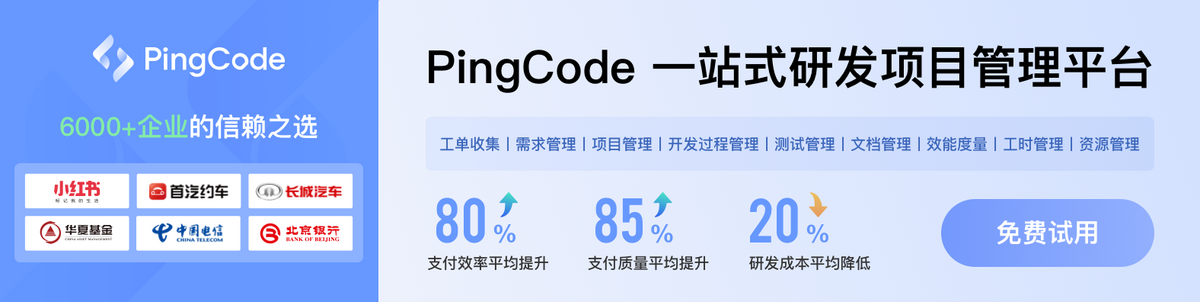