使用Python统计文件中的单词个数,可以通过读取文件内容、分割单词、统计单词数量等步骤来实现。关键步骤包括:读取文件内容、使用正则表达式分割单词、统计单词数量。
详细描述:读取文件内容是第一步,通过Python内置的open
函数,可以方便地读取文件中的所有内容。接下来,利用正则表达式可以精准地将文本内容分割成单词,这样可以避免标点符号的干扰。最后,通过统计分割后的单词列表的长度,就可以得出文件中的单词数量。
一、读取文件内容
要统计一个文件中的单词数量,首先需要读取文件的内容。Python提供了多种读取文件的方式,其中最常用的是使用open
函数。我们可以选择以文本模式(默认模式)打开文件,并使用read
方法读取文件的全部内容。
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
return content
在上面的代码中,使用open
函数打开文件并读取其内容。with
语句可以确保文件在使用完毕后自动关闭,encoding='utf-8'
确保正确处理文件中的非ASCII字符。
二、分割单词
读取文件内容后,需要将内容分割成一个个单词。Python的re
模块提供了强大的正则表达式功能,可以用来匹配单词。以下示例代码展示了如何使用正则表达式分割单词。
import re
def split_into_words(text):
words = re.findall(r'\b\w+\b', text.lower())
return words
在上面的代码中,re.findall
函数用于查找所有匹配的单词。正则表达式r'\b\w+\b'
匹配一个或多个字母、数字或下划线组成的单词。通过调用text.lower()
,可以将文本内容转换为小写,这样可以使单词统计不区分大小写。
三、统计单词数量
分割单词后,只需统计单词列表的长度即可得到文件中的单词数量。
def count_words(words):
return len(words)
在上面的代码中,len
函数返回单词列表的长度,即文件中的单词数量。
四、完整示例
以下是一个完整的示例,展示了如何结合上述步骤来统计文件中的单词数量。
import re
def read_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
content = file.read()
return content
def split_into_words(text):
words = re.findall(r'\b\w+\b', text.lower())
return words
def count_words(words):
return len(words)
def main(file_path):
content = read_file(file_path)
words = split_into_words(content)
word_count = count_words(words)
print(f'The file contains {word_count} words.')
if __name__ == '__main__':
file_path = 'your_file.txt'
main(file_path)
在这个示例中,main
函数将各个步骤串联起来,最终输出文件中的单词数量。请将file_path
替换为实际文件的路径。
五、处理大文件
对于非常大的文件,直接读取全部内容可能会导致内存不足。为了处理这种情况,可以逐行读取文件内容,并逐行统计单词数量。以下示例展示了如何逐行统计单词数量。
import re
def count_words_in_file(file_path):
word_count = 0
with open(file_path, 'r', encoding='utf-8') as file:
for line in file:
words = re.findall(r'\b\w+\b', line.lower())
word_count += len(words)
return word_count
def main(file_path):
word_count = count_words_in_file(file_path)
print(f'The file contains {word_count} words.')
if __name__ == '__main__':
file_path = 'your_file.txt'
main(file_path)
在这个示例中,count_words_in_file
函数逐行读取文件,并逐行统计单词数量。这样可以有效避免因文件过大而导致的内存不足问题。
六、处理不同编码格式的文件
有时文件可能使用不同的编码格式。为了确保能够正确读取文件内容,可以使用chardet
库自动检测文件编码,并使用检测到的编码来读取文件。
import re
import chardet
def read_file_with_encoding(file_path):
with open(file_path, 'rb') as file:
raw_data = file.read()
encoding = chardet.detect(raw_data)['encoding']
content = raw_data.decode(encoding)
return content
def split_into_words(text):
words = re.findall(r'\b\w+\b', text.lower())
return words
def count_words(words):
return len(words)
def main(file_path):
content = read_file_with_encoding(file_path)
words = split_into_words(content)
word_count = count_words(words)
print(f'The file contains {word_count} words.')
if __name__ == '__main__':
file_path = 'your_file.txt'
main(file_path)
在这个示例中,chardet
库用于自动检测文件编码,并使用检测到的编码解码文件内容。这样可以确保文件内容能够正确读取。
七、忽略特定字符和符号
在某些情况下,可能需要忽略特定的字符和符号。例如,我们可能希望忽略标点符号和数字,只统计字母组成的单词。可以通过修改正则表达式来实现这一点。
import re
def split_into_words(text):
words = re.findall(r'\b[a-zA-Z]+\b', text.lower())
return words
def count_words(words):
return len(words)
def main(file_path):
content = read_file(file_path)
words = split_into_words(content)
word_count = count_words(words)
print(f'The file contains {word_count} words.')
if __name__ == '__main__':
file_path = 'your_file.txt'
main(file_path)
在上面的代码中,正则表达式r'\b[a-zA-Z]+\b'
只匹配由字母组成的单词,从而忽略其他字符和符号。
八、统计不同单词的数量
除了统计文件中的总单词数量,有时还需要统计文件中不同单词的数量。可以使用Python的collections.Counter
类来实现这一点。
import re
from collections import Counter
def split_into_words(text):
words = re.findall(r'\b\w+\b', text.lower())
return words
def count_unique_words(words):
word_counter = Counter(words)
return word_counter
def main(file_path):
content = read_file(file_path)
words = split_into_words(content)
word_counter = count_unique_words(words)
print(f'The file contains {len(word_counter)} unique words.')
print('Most common words:', word_counter.most_common(10))
if __name__ == '__main__':
file_path = 'your_file.txt'
main(file_path)
在这个示例中,Counter
类用于统计每个单词的出现次数。most_common
方法返回出现次数最多的前10个单词及其出现次数。
九、处理多种语言的文件
如果文件中包含多种语言的文本,可能需要使用更复杂的分词方法。Python的jieba
库可以用于中文分词,nltk
库可以用于多种语言的分词。
import re
import jieba
from nltk.tokenize import word_tokenize
def split_into_words(text, language='english'):
if language == 'chinese':
words = jieba.lcut(text)
else:
words = word_tokenize(text)
return words
def count_words(words):
return len(words)
def main(file_path, language='english'):
content = read_file(file_path)
words = split_into_words(content, language)
word_count = count_words(words)
print(f'The file contains {word_count} words.')
if __name__ == '__main__':
file_path = 'your_file.txt'
main(file_path, language='chinese')
在这个示例中,根据指定的语言选择不同的分词方法。jieba.lcut
用于中文分词,word_tokenize
用于其他语言的分词。
十、总结
通过本文的介绍,我们学习了如何使用Python统计文件中的单词数量。我们介绍了读取文件内容、分割单词、统计单词数量的基本方法,并讨论了处理大文件、不同编码格式的文件、忽略特定字符和符号、统计不同单词的数量、以及处理多种语言的文件等高级话题。通过掌握这些方法和技巧,可以更灵活地统计和分析文件中的单词数量。
相关问答FAQs:
如何在Python中读取文件内容以统计单词个数?
要统计文件中的单词个数,首先需要使用Python打开并读取文件。可以使用内置的open()
函数读取文件内容,然后通过split()
方法将内容分割成单词。接着,使用len()
函数计算单词的数量。以下是一个示例代码:
with open('filename.txt', 'r') as file:
content = file.read()
words = content.split()
word_count = len(words)
print(f'文件中的单词总数为: {word_count}')
是否可以统计特定单词在文件中出现的次数?
在Python中,统计特定单词的出现次数是非常简单的。读取文件内容后,可以使用count()
方法来计算特定单词的出现频率。例如:
target_word = 'example'
with open('filename.txt', 'r') as file:
content = file.read()
occurrences = content.lower().count(target_word.lower())
print(f'单词"{target_word}"在文件中出现的次数为: {occurrences}')
这样可以确保统计时不区分大小写。
如何处理文件中的标点符号以提高统计的准确性?
在统计单词个数时,标点符号可能会导致结果不准确。可以使用re
模块中的正则表达式来清理文本。例如,可以通过替换或删除标点符号来获得更准确的单词计数:
import re
with open('filename.txt', 'r') as file:
content = file.read()
# 使用正则表达式替换标点符号
cleaned_content = re.sub(r'[^\w\s]', '', content)
words = cleaned_content.split()
word_count = len(words)
print(f'清理后文件中的单词总数为: {word_count}')
这种方法有助于确保统计结果的准确性。
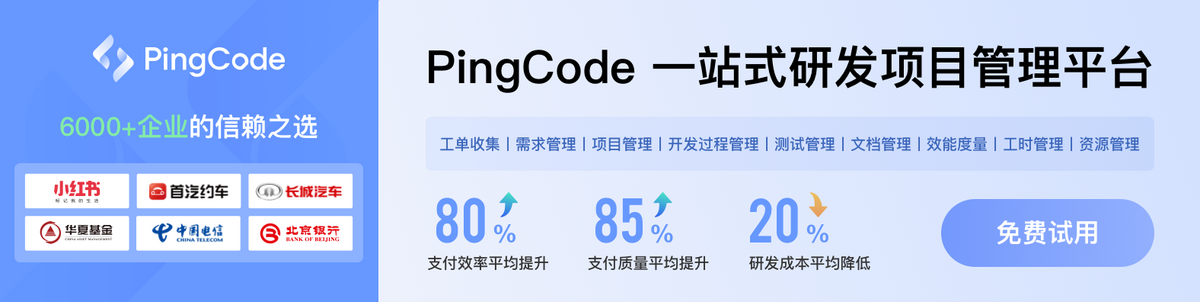