通过Python命令行导入别的类的方法有多种,包括使用import语句、sys.path、PYTHONPATH环境变量等。 其中最常见的方法是使用import语句导入模块或类。接下来,我们将详细讨论这些方法并提供示例。
一、使用import语句
在Python中,最简单的方法是使用import语句直接导入所需的模块或类。假设我们有一个名为my_module.py
的文件,其中包含一个名为MyClass
的类。我们可以在命令行中导入这个类并创建其实例。
# my_module.py
class MyClass:
def __init__(self):
print("MyClass instance created")
在命令行中,可以通过以下方式导入并使用该类:
>>> from my_module import MyClass
>>> instance = MyClass()
MyClass instance created
二、使用sys.path
如果你的模块不在当前目录下,可以使用sys.path
添加模块所在的目录。sys.path
是一个包含目录路径的列表,Python会在这些路径中搜索模块。
import sys
sys.path.append('/path/to/your/module')
from my_module import MyClass
instance = MyClass()
三、使用PYTHONPATH环境变量
另一种方法是设置PYTHONPATH
环境变量,这样Python会在指定的路径中搜索模块。可以在终端中设置此环境变量:
export PYTHONPATH=/path/to/your/module
然后在命令行中导入模块:
from my_module import MyClass
instance = MyClass()
四、相对导入
在大型项目中,模块可能位于包中,可以使用相对导入。假设我们有以下目录结构:
project/
├── package/
│ ├── __init__.py
│ ├── module_a.py
│ └── module_b.py
└── main.py
在module_a.py
中有一个类ClassA
,我们想在module_b.py
中导入并使用它:
# module_a.py
class ClassA:
def __init__(self):
print("ClassA instance created")
# module_b.py
from .module_a import ClassA
class ClassB:
def __init__(self):
instance = ClassA()
print("ClassB instance created")
五、绝对导入
在同一个包中,可以使用绝对导入。这种方法在大型项目中更常见,因为它使代码更加清晰和易于维护。
# module_b.py
from package.module_a import ClassA
class ClassB:
def __init__(self):
instance = ClassA()
print("ClassB instance created")
六、导入多个类
如果一个模块中有多个类,可以一次性导入所有需要的类:
# my_module.py
class MyClass1:
def __init__(self):
print("MyClass1 instance created")
class MyClass2:
def __init__(self):
print("MyClass2 instance created")
>>> from my_module import MyClass1, MyClass2
>>> instance1 = MyClass1()
MyClass1 instance created
>>> instance2 = MyClass2()
MyClass2 instance created
七、导入模块中的所有内容
可以使用import *
导入模块中的所有内容,但这种方法不推荐使用,因为它可能导致命名空间污染。
>>> from my_module import *
>>> instance1 = MyClass1()
MyClass1 instance created
>>> instance2 = MyClass2()
MyClass2 instance created
八、使用命令行参数
在某些情况下,可以通过命令行参数动态导入模块和类。例如,使用argparse
模块解析命令行参数并导入类:
import argparse
import importlib
parser = argparse.ArgumentParser(description="Import and use a class from a module.")
parser.add_argument("module", help="The module to import")
parser.add_argument("class_name", help="The class to import")
args = parser.parse_args()
module = importlib.import_module(args.module)
class_ = getattr(module, args.class_name)
instance = class_()
print(f"{args.class_name} instance created")
运行此脚本时,可以指定模块和类:
python script.py my_module MyClass1
以上是通过Python命令行导入别的类的多种方法。这些方法根据不同的需求和场景提供了灵活的选择,使得代码更加模块化和可维护。通过合理使用这些方法,可以轻松实现代码的复用和组织。
相关问答FAQs:
如何在Python命令行中导入自定义模块?
在Python命令行中,您可以使用import
语句导入自定义模块。首先,确保您的自定义模块文件(例如my_module.py
)位于当前工作目录中或Python的模块搜索路径中。您可以使用import my_module
导入模块,并通过my_module.ClassName
来访问其中的类。
在Python命令行中如何处理导入错误?
如果在导入模块时遇到错误,通常是因为模块未在搜索路径中,或文件名拼写错误。您可以使用sys.path
查看当前的模块搜索路径,确保您的模块文件在这些路径中。此外,检查文件名和类名的拼写是否正确也很重要。
如何在Python命令行中导入特定类而非整个模块?
要在Python命令行中导入特定类,可以使用from ... import ...
语法。例如,如果您只想导入MyClass
类,可以使用from my_module import MyClass
。这样,您可以直接使用MyClass
而无需加上模块名前缀。
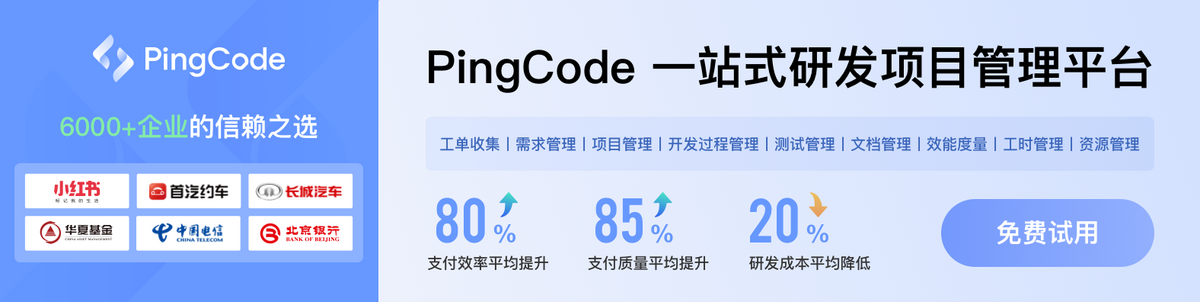