要将字符串复制到Python中的变量,可以使用赋值运算符(=)来实现。 例如,如果你有一个字符串并且想将其复制到一个变量中,可以像下面这样做:
original_string = "Hello, World!"
copied_string = original_string
在这个例子中,original_string
的值被复制到 copied_string
中。现在 copied_string
中包含了与 original_string
相同的字符串内容。你可以通过修改其中的一个变量来验证它们是否独立。
一、字符串赋值的基本操作
在Python中,字符串是一种不可变的数据类型,这意味着字符串一旦创建,就无法被修改。正因为如此,字符串赋值在实际使用中非常简单,只需要使用赋值运算符即可。以下是一些基本操作示例:
original_string = "Python is awesome!"
new_string = original_string
print(new_string) # 输出: Python is awesome!
在这个例子中,new_string
被赋值为 original_string
的值。由于字符串是不可变的,因此 new_string
和 original_string
都指向相同的字符串对象。
二、字符串的深拷贝与浅拷贝
在Python中,字符串的赋值操作实际上是浅拷贝,因为字符串是不可变的,所以浅拷贝和深拷贝没有什么区别。然而,为了理解深浅拷贝的概念,我们可以通过列表来解释。
import copy
original_list = ["a", "b", "c"]
shallow_copy = original_list
deep_copy = copy.deepcopy(original_list)
original_list[0] = "z"
print(original_list) # 输出: ['z', 'b', 'c']
print(shallow_copy) # 输出: ['z', 'b', 'c']
print(deep_copy) # 输出: ['a', 'b', 'c']
在这个例子中,shallow_copy
和 original_list
指向同一个对象,因此修改 original_list
也会影响 shallow_copy
。但 deep_copy
是 original_list
的深拷贝,修改 original_list
不会影响 deep_copy
。
三、字符串操作的实际应用
在实际应用中,我们经常需要对字符串进行各种操作,如拼接、分割、替换等。以下是一些常见的字符串操作示例:
1. 字符串拼接
字符串拼接可以使用 +
运算符或 join
方法:
str1 = "Hello"
str2 = "World"
combined_str = str1 + ", " + str2 + "!"
print(combined_str) # 输出: Hello, World!
或者使用 join
方法:
words = ["Hello", "World"]
combined_str = ", ".join(words) + "!"
print(combined_str) # 输出: Hello, World!
2. 字符串分割
字符串分割可以使用 split
方法:
sentence = "Python is awesome"
words = sentence.split()
print(words) # 输出: ['Python', 'is', 'awesome']
你还可以指定分隔符:
sentence = "Python,is,awesome"
words = sentence.split(",")
print(words) # 输出: ['Python', 'is', 'awesome']
3. 字符串替换
字符串替换可以使用 replace
方法:
sentence = "Python is awesome"
new_sentence = sentence.replace("awesome", "fantastic")
print(new_sentence) # 输出: Python is fantastic
四、字符串格式化
字符串格式化是将变量的值插入到字符串中的一种方法。Python 提供了多种字符串格式化的方法:
1. 使用 %
运算符
name = "John"
age = 25
formatted_str = "Name: %s, Age: %d" % (name, age)
print(formatted_str) # 输出: Name: John, Age: 25
2. 使用 format
方法
formatted_str = "Name: {}, Age: {}".format(name, age)
print(formatted_str) # 输出: Name: John, Age: 25
3. 使用 f-string (Python 3.6+)
formatted_str = f"Name: {name}, Age: {age}"
print(formatted_str) # 输出: Name: John, Age: 25
五、字符串的编码与解码
在处理字符串时,有时需要进行编码和解码操作,特别是在处理文件输入输出或网络通信时。Python 提供了方便的编码和解码方法:
1. 编码
将字符串编码为字节序列:
original_string = "Hello, World!"
encoded_string = original_string.encode("utf-8")
print(encoded_string) # 输出: b'Hello, World!'
2. 解码
将字节序列解码为字符串:
decoded_string = encoded_string.decode("utf-8")
print(decoded_string) # 输出: Hello, World!
六、字符串的常用方法
Python 提供了许多内置的字符串方法,以下是一些常用的方法:
1. upper
和 lower
将字符串转换为全大写或全小写:
original_string = "Hello, World!"
upper_string = original_string.upper()
lower_string = original_string.lower()
print(upper_string) # 输出: HELLO, WORLD!
print(lower_string) # 输出: hello, world!
2. strip
移除字符串两端的空白字符:
original_string = " Hello, World! "
stripped_string = original_string.strip()
print(stripped_string) # 输出: Hello, World!
3. startswith
和 endswith
检查字符串是否以特定子字符串开头或结尾:
original_string = "Hello, World!"
starts_with_hello = original_string.startswith("Hello")
ends_with_world = original_string.endswith("World!")
print(starts_with_hello) # 输出: True
print(ends_with_world) # 输出: True
七、字符串的正则表达式
正则表达式是一种强大的字符串匹配工具,Python 提供了 re
模块来支持正则表达式操作:
1. 匹配
使用 match
方法匹配字符串:
import re
pattern = r"Hello"
string = "Hello, World!"
match = re.match(pattern, string)
if match:
print("Match found!") # 输出: Match found!
else:
print("No match found.")
2. 搜索
使用 search
方法搜索字符串中的模式:
pattern = r"World"
search = re.search(pattern, string)
if search:
print("Search found!") # 输出: Search found!
else:
print("No search found.")
3. 替换
使用 sub
方法替换字符串中的模式:
pattern = r"World"
replacement = "Universe"
new_string = re.sub(pattern, replacement, string)
print(new_string) # 输出: Hello, Universe!
八、字符串的多行操作
在Python中,可以使用三重引号('''
或 """
)来创建多行字符串:
multi_line_string = """This is a
multi-line
string."""
print(multi_line_string)
这种方法在处理长字符串或包含换行符的字符串时非常有用。
九、字符串的分割与连接
字符串的分割与连接是处理文本数据时常用的操作:
1. 分割
使用 split
方法将字符串分割为列表:
sentence = "Python is awesome"
words = sentence.split()
print(words) # 输出: ['Python', 'is', 'awesome']
2. 连接
使用 join
方法将列表连接为字符串:
words = ["Python", "is", "awesome"]
sentence = " ".join(words)
print(sentence) # 输出: Python is awesome
十、字符串的查找与计数
字符串的查找与计数操作在文本分析中非常有用:
1. 查找
使用 find
方法查找子字符串的位置:
sentence = "Python is awesome"
position = sentence.find("awesome")
print(position) # 输出: 10
2. 计数
使用 count
方法统计子字符串出现的次数:
sentence = "Python is awesome. Python is great."
count = sentence.count("Python")
print(count) # 输出: 2
十一、字符串的验证
字符串的验证操作用于检查字符串是否满足某些条件:
1. 是否全为字母
使用 isalpha
方法检查字符串是否全为字母:
string = "Python"
is_alpha = string.isalpha()
print(is_alpha) # 输出: True
2. 是否全为数字
使用 isdigit
方法检查字符串是否全为数字:
string = "12345"
is_digit = string.isdigit()
print(is_digit) # 输出: True
十二、字符串的大小写转换
字符串的大小写转换在文本处理和格式化时非常常见:
1. 大写转小写
使用 lower
方法将字符串转换为小写:
string = "HELLO, WORLD!"
lower_string = string.lower()
print(lower_string) # 输出: hello, world!
2. 小写转大写
使用 upper
方法将字符串转换为大写:
string = "hello, world!"
upper_string = string.upper()
print(upper_string) # 输出: HELLO, WORLD!
十三、字符串的字符替换
字符串的字符替换用于修改字符串中的特定字符:
1. 使用 replace
方法
string = "Hello, World!"
new_string = string.replace("World", "Python")
print(new_string) # 输出: Hello, Python!
2. 使用正则表达式进行复杂替换
import re
string = "Hello, World!"
pattern = r"World"
replacement = "Python"
new_string = re.sub(pattern, replacement, string)
print(new_string) # 输出: Hello, Python!
十四、字符串的切片操作
字符串的切片操作用于提取字符串的子字符串:
1. 基本切片
string = "Hello, World!"
substring = string[7:12]
print(substring) # 输出: World
2. 步长切片
string = "Hello, World!"
substring = string[0:12:2]
print(substring) # 输出: Hlo ol
十五、字符串的反转
字符串的反转操作在某些算法中非常有用:
1. 使用切片操作反转字符串
string = "Hello, World!"
reversed_string = string[::-1]
print(reversed_string) # 输出: !dlroW ,olleH
十六、字符串的比较
字符串的比较用于检查两个字符串是否相等或比较它们的字典序:
1. 使用 ==
运算符比较字符串
string1 = "Python"
string2 = "Python"
is_equal = string1 == string2
print(is_equal) # 输出: True
2. 使用 >
和 <
运算符比较字符串的字典序
string1 = "Python"
string2 = "Java"
is_greater = string1 > string2
print(is_greater) # 输出: True
通过以上详细的内容,我们可以看到Python提供了丰富的字符串操作方法,使得字符串处理变得非常方便和高效。掌握这些方法可以极大地提高编码效率和代码的可读性。
相关问答FAQs:
如何在Python中创建字符串的副本?
在Python中,可以通过简单的赋值语句将字符串复制到另一个变量中。例如,使用new_string = original_string
语句会将original_string
的内容复制到new_string
中。需要注意的是,字符串在Python中是不可变的,因此对new_string
的任何更改不会影响original_string
。
是否可以使用切片或其他方法来复制字符串?
当然可以!切片是一个常用的方法,您可以使用new_string = original_string[:]
来创建字符串的副本。此外,还可以使用str()
函数,例如new_string = str(original_string)
,这两种方法都能有效地复制字符串。
在复制字符串时是否会影响内存使用?
在Python中,字符串是不可变的,因此复制字符串会在内存中创建一个新的字符串对象。虽然Python会在某些情况下优化内存使用(例如,两个变量可能指向同一个字符串对象),一般来说,创建副本会消耗额外的内存。为了更好地管理内存,尽量避免不必要的字符串复制。
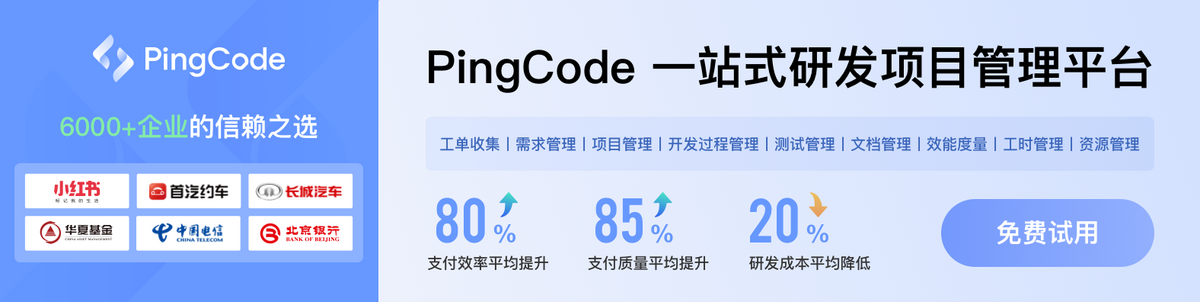