在Python中,可以通过以下几种方法在函数内调用另一个函数:直接调用、嵌套函数、通过参数传递函数、使用闭包和装饰器等。直接调用、嵌套函数、通过参数传递函数是最常见的方法。直接调用是指在一个函数内部直接调用另一个函数。这种方法非常直观和简单,适用于大多数情况。下面将详细介绍这些方法并提供示例代码。
一、直接调用
直接调用是指在一个函数内部直接调用另一个函数。这种方法非常直观和简单,适用于大多数情况。
def outer_function():
print("This is the outer function")
def inner_function():
print("This is the inner function")
inner_function()
outer_function()
在上述代码中,inner_function
被直接在 outer_function
内部调用。
二、嵌套函数
嵌套函数是指在一个函数内部定义另一个函数。嵌套函数可以访问其外部函数的变量和参数。
def outer_function(x):
def inner_function(y):
return x + y
result = inner_function(5)
print(result)
outer_function(10)
在这个例子中,inner_function
是 outer_function
的嵌套函数,它可以访问 outer_function
的参数 x
。
三、通过参数传递函数
可以将一个函数作为参数传递给另一个函数,并在被调用的函数中执行它。
def add(x, y):
return x + y
def operate(func, x, y):
return func(x, y)
result = operate(add, 10, 5)
print(result)
在这个示例中,add
函数被作为参数传递给 operate
函数,并在 operate
函数中被调用。
四、使用闭包
闭包是指一个函数对象,即使在其词法作用域外被调用时,仍然能够访问其词法作用域内的变量。
def outer_function(x):
def inner_function(y):
return x + y
return inner_function
closure = outer_function(10)
result = closure(5)
print(result)
在这个示例中,outer_function
返回 inner_function
,并且 inner_function
保留了对 x
的访问权。
五、使用装饰器
装饰器是一个函数,它接收另一个函数作为参数,并返回一个新的函数。装饰器常用于在不修改原函数代码的情况下,增加额外的功能。
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
在这个例子中,my_decorator
是一个装饰器,它在 say_hello
函数的前后添加了额外的打印语句。
六、递归函数
递归函数是指一个函数在其内部调用自己。这种方法通常用于解决分治问题,如计算阶乘、斐波那契数列等。
def factorial(n):
if n == 1:
return 1
else:
return n * factorial(n-1)
result = factorial(5)
print(result)
在这个例子中,factorial
函数通过递归的方式计算阶乘。
七、使用类和方法
在面向对象编程中,可以在一个方法中调用同一个类中的另一个方法。
class MyClass:
def method1(self):
print("This is method1")
def method2(self):
print("This is method2")
self.method1()
obj = MyClass()
obj.method2()
在这个示例中,method2
调用了 method1
。
八、匿名函数(Lambda)
匿名函数是一种没有名字的函数,通常用于短小的函数。可以在函数内部定义并调用匿名函数。
def outer_function(x):
inner_function = lambda y: x + y
result = inner_function(5)
print(result)
outer_function(10)
在这个例子中,inner_function
是一个匿名函数,通过 lambda
关键字定义,并在 outer_function
内部调用。
九、生成器函数
生成器函数是使用 yield
关键字的函数,它返回一个生成器对象,可以在函数内部调用生成器函数来生成一系列值。
def generator_function():
for i in range(5):
yield i
def caller_function():
for value in generator_function():
print(value)
caller_function()
在这个示例中,generator_function
是一个生成器函数,通过 yield
关键字生成一系列值,并在 caller_function
中调用。
十、动态函数调用
在某些情况下,可能需要根据运行时条件动态调用不同的函数。可以使用内置函数 getattr
动态调用函数。
class MyClass:
def method1(self):
print("This is method1")
def method2(self):
print("This is method2")
def call_method(self, method_name):
method = getattr(self, method_name)
method()
obj = MyClass()
obj.call_method('method1')
obj.call_method('method2')
在这个示例中,call_method
方法根据传入的 method_name
动态调用相应的方法。
通过以上方法,可以在Python中灵活地在函数内调用另一个函数。不同的方法适用于不同的场景,选择合适的方法可以提高代码的可读性和可维护性。
相关问答FAQs:
在Python中,如何在一个函数内调用另一个函数?
在Python中,可以直接在一个函数内部调用另一个函数,只需使用被调用函数的名称并传递必要的参数。例如,如果你有两个函数func_a
和func_b
,可以在func_a
中调用func_b
。确保在调用之前定义了这两个函数,以避免出现未定义的错误。
调用函数时需要注意什么?
调用函数时要确保参数类型和数量正确。如果被调用函数期望接收参数,而调用时没有提供或者提供了错误类型的参数,会引发错误。此外,也需要注意局部和全局变量的作用域,确保在函数内部访问到所需的数据。
如何处理函数调用中的返回值?
在函数调用后,可以通过返回值来获取被调用函数的输出。如果被调用函数有返回值,可以将其赋值给一个变量,方便后续操作。在编写函数时,合理设计返回值,可以提高代码的可读性和可维护性。
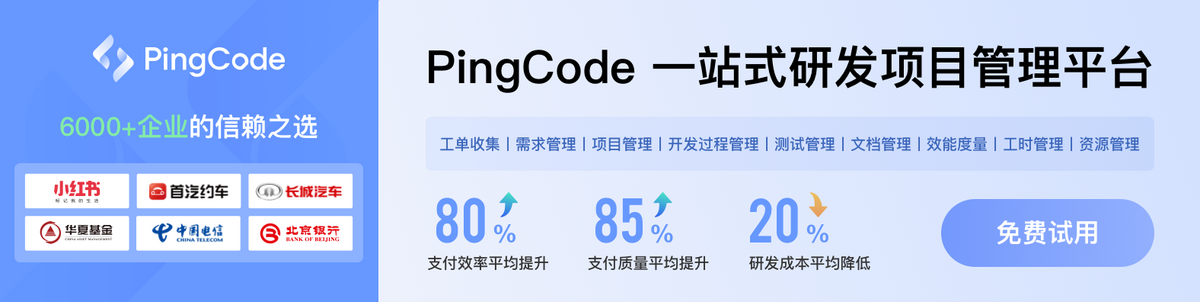