如何用Python制作一个题库
使用Python制作一个题库有以下几个步骤:理解题库结构、设计数据存储方式、编写代码实现题库功能、增加用户交互功能。其中,设计数据存储方式是关键部分,下面将详细解释。
一、理解题库结构
在开始编写代码之前,首先要明确题库的结构。题库通常包括以下几个部分:
- 题目ID
- 题目内容
- 选项(如果是选择题)
- 正确答案
- 题目类型(选择题、填空题、简答题等)
- 题目难度
二、设计数据存储方式
题库的数据可以存储在多种格式的文件中,例如CSV文件、JSON文件或者数据库中。这里我们选择使用JSON文件来存储题库数据,因为JSON格式易于人类阅读和编写,并且Python提供了方便的模块来处理JSON数据。
以下是一个示例题库的JSON格式:
[
{
"id": 1,
"content": "What is the capital of France?",
"options": ["Paris", "London", "Berlin", "Madrid"],
"answer": "Paris",
"type": "multiple_choice",
"difficulty": "easy"
},
{
"id": 2,
"content": "Solve the equation: 2x + 3 = 7.",
"answer": "2",
"type": "short_answer",
"difficulty": "medium"
}
]
三、编写代码实现题库功能
接下来,用Python编写代码来实现题库的基本功能,包括读取题库、添加题目、删除题目和修改题目。
1. 读取题库
首先,编写一个函数从JSON文件中读取题库数据:
import json
def load_question_bank(file_path):
with open(file_path, 'r') as file:
question_bank = json.load(file)
return question_bank
2. 添加题目
编写一个函数向题库中添加新题目:
def add_question(question_bank, question):
question_bank.append(question)
return question_bank
3. 删除题目
编写一个函数从题库中删除题目:
def delete_question(question_bank, question_id):
question_bank = [q for q in question_bank if q['id'] != question_id]
return question_bank
4. 修改题目
编写一个函数修改题目:
def update_question(question_bank, question_id, updated_question):
for i, question in enumerate(question_bank):
if question['id'] == question_id:
question_bank[i] = updated_question
break
return question_bank
四、增加用户交互功能
为了让题库更加实用,可以添加一些用户交互功能,例如通过命令行输入来添加、删除和修改题目。
1. 命令行交互
以下是一个简单的命令行交互示例:
def main():
file_path = 'question_bank.json'
question_bank = load_question_bank(file_path)
while True:
print("1. View Questions")
print("2. Add Question")
print("3. Delete Question")
print("4. Update Question")
print("5. Exit")
choice = input("Enter your choice: ")
if choice == '1':
for question in question_bank:
print(question)
elif choice == '2':
content = input("Enter question content: ")
q_type = input("Enter question type (multiple_choice/short_answer): ")
difficulty = input("Enter question difficulty (easy/medium/hard): ")
if q_type == 'multiple_choice':
options = input("Enter options separated by commas: ").split(',')
answer = input("Enter correct answer: ")
question = {
"id": len(question_bank) + 1,
"content": content,
"options": options,
"answer": answer,
"type": q_type,
"difficulty": difficulty
}
else:
answer = input("Enter correct answer: ")
question = {
"id": len(question_bank) + 1,
"content": content,
"answer": answer,
"type": q_type,
"difficulty": difficulty
}
question_bank = add_question(question_bank, question)
elif choice == '3':
question_id = int(input("Enter question ID to delete: "))
question_bank = delete_question(question_bank, question_id)
elif choice == '4':
question_id = int(input("Enter question ID to update: "))
content = input("Enter new question content: ")
q_type = input("Enter new question type (multiple_choice/short_answer): ")
difficulty = input("Enter new question difficulty (easy/medium/hard): ")
if q_type == 'multiple_choice':
options = input("Enter new options separated by commas: ").split(',')
answer = input("Enter new correct answer: ")
updated_question = {
"id": question_id,
"content": content,
"options": options,
"answer": answer,
"type": q_type,
"difficulty": difficulty
}
else:
answer = input("Enter new correct answer: ")
updated_question = {
"id": question_id,
"content": content,
"answer": answer,
"type": q_type,
"difficulty": difficulty
}
question_bank = update_question(question_bank, question_id, updated_question)
elif choice == '5':
with open(file_path, 'w') as file:
json.dump(question_bank, file)
break
else:
print("Invalid choice. Please try again.")
if __name__ == "__main__":
main()
五、扩展功能
除了基本功能,还可以扩展题库的功能,例如:
- 分类浏览题目:按题目类型或难度分类浏览题目。
- 随机抽取题目:从题库中随机抽取一定数量的题目。
- 答题模式:模拟考试,用户可以回答题目并获取评分。
1. 分类浏览题目
def view_questions_by_type(question_bank, q_type):
for question in question_bank:
if question['type'] == q_type:
print(question)
def view_questions_by_difficulty(question_bank, difficulty):
for question in question_bank:
if question['difficulty'] == difficulty:
print(question)
2. 随机抽取题目
import random
def get_random_questions(question_bank, num_questions):
return random.sample(question_bank, num_questions)
3. 答题模式
def quiz_mode(question_bank):
score = 0
total = len(question_bank)
for question in question_bank:
print(question['content'])
if question['type'] == 'multiple_choice':
for i, option in enumerate(question['options']):
print(f"{i+1}. {option}")
answer = input("Enter your answer: ")
if question['options'][int(answer)-1] == question['answer']:
score += 1
else:
answer = input("Enter your answer: ")
if answer == question['answer']:
score += 1
print(f"Your score is {score}/{total}")
通过以上步骤,我们可以使用Python制作一个功能齐全的题库。这个题库不仅可以存储和管理题目,还可以提供分类浏览、随机抽取和答题模式等功能,极大地方便了用户的使用。
相关问答FAQs:
如何开始使用Python创建一个题库?
要开始创建题库,您需要安装Python和相关库,如SQLite或Pandas。接着,您可以设计一个数据库架构,决定题目、选项及正确答案等字段。通过编写Python代码,您可以实现题目的添加、删除和查询功能。
可以使用哪些数据结构来存储题库中的题目?
在Python中,可以使用字典、列表或自定义类来存储题目。字典可以方便地将题目与其选项和答案关联,而列表则适合存储多个题目。使用自定义类可以让您更灵活地管理题目的属性和方法。
如何让题库支持多种题型?
为了支持多种题型,您可以在题库中添加一个字段,用于标识题目的类型,如选择题、填空题或判断题。根据不同题型的要求,您可以设计不同的数据结构和处理逻辑,从而实现题库的多样性。
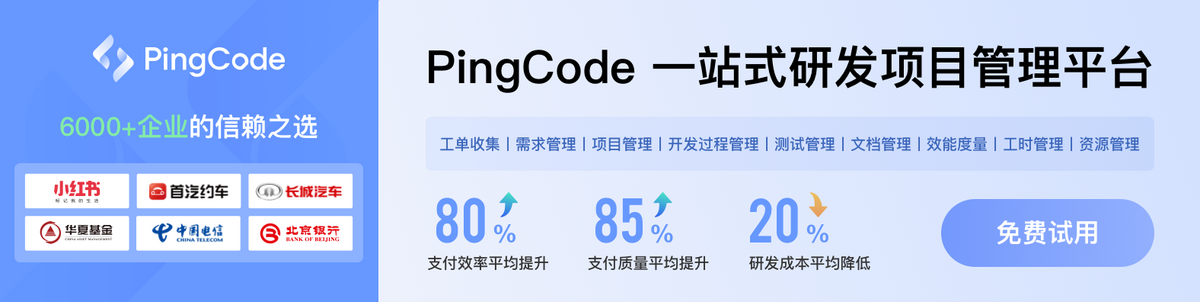