使用Python可以通过多种方式来实现语录循环十次的功能,主要方法包括使用for
循环、while
循环等。下面将详细介绍使用for
循环的方式。
一、for
循环实现语录循环十次
for
循环是一种简单且直观的方法,可以通过设置循环次数来实现语录的重复打印。以下是具体的实现步骤:
1、基础的for
循环
在Python中,for
循环可以通过range
函数来控制循环的次数。假设有一个语录字符串,我们希望它循环打印十次,可以这样实现:
quote = "The only limit to our realization of tomorrow is our doubts of today."
for i in range(10):
print(quote)
这个代码块中,range(10)
生成一个从0到9的数字序列,for
循环会遍历这个序列,每次循环都会执行一次print(quote)
,从而实现语录循环十次。
2、添加编号
为了更清楚地看到每次循环的结果,我们可以在打印语录时添加编号:
quote = "The only limit to our realization of tomorrow is our doubts of today."
for i in range(10):
print(f"{i+1}: {quote}")
这里使用了Python的f-string来格式化字符串,{i+1}
用于显示当前的循环次数。
二、while
循环实现语录循环十次
除了for
循环,while
循环也是一种常见的循环控制方式。它通过判断条件是否满足来决定是否继续循环。以下是具体实现:
1、基础的while
循环
quote = "The only limit to our realization of tomorrow is our doubts of today."
count = 0
while count < 10:
print(quote)
count += 1
在这个代码块中,count
变量初始化为0,每次循环后增加1,当count
达到10时,条件count < 10
不再满足,循环结束。
2、添加编号
同样,我们可以在while
循环中添加编号:
quote = "The only limit to our realization of tomorrow is our doubts of today."
count = 0
while count < 10:
print(f"{count + 1}: {quote}")
count += 1
三、使用函数实现语录循环十次
将循环逻辑封装到函数中,可以提高代码的可复用性和可读性。以下是具体实现:
1、使用for
循环的函数
def print_quote_n_times(quote, n):
for i in range(n):
print(f"{i + 1}: {quote}")
quote = "The only limit to our realization of tomorrow is our doubts of today."
print_quote_n_times(quote, 10)
2、使用while
循环的函数
def print_quote_n_times(quote, n):
count = 0
while count < n:
print(f"{count + 1}: {quote}")
count += 1
quote = "The only limit to our realization of tomorrow is our doubts of today."
print_quote_n_times(quote, 10)
四、使用列表和循环结合实现多条语录循环十次
如果有多条语录需要循环打印,可以使用列表和循环结合的方式实现。以下是具体实现:
quotes = [
"The only limit to our realization of tomorrow is our doubts of today.",
"Do not wait to strike till the iron is hot; but make it hot by striking.",
"Great minds discuss ideas; average minds discuss events; small minds discuss people."
]
for quote in quotes:
for i in range(10):
print(f"{i + 1}: {quote}")
在这个代码块中,外层for
循环遍历每一条语录,内层for
循环实现单条语录的十次打印。
五、使用itertools
模块实现循环
Python的itertools
模块提供了强大的迭代器工具,可以简化循环操作。以下是具体实现:
import itertools
quote = "The only limit to our realization of tomorrow is our doubts of today."
for i, _ in zip(range(10), itertools.repeat(quote)):
print(f"{i + 1}: {quote}")
在这个代码块中,itertools.repeat(quote)
生成一个无限重复的迭代器,通过zip(range(10), ...)
限制只循环十次。
六、总结
以上介绍了多种实现Python语录循环十次的方法,包括for
循环、while
循环、函数封装、列表结合循环、以及使用itertools
模块。这些方法各有优劣,选择具体实现方式时,可以根据实际需求和代码风格进行调整。无论使用哪种方法,关键在于理解循环控制的基本原理,确保代码逻辑清晰、可读性强。
相关问答FAQs:
如何使用Python循环输出语录?
可以使用Python中的for
循环来实现语录的循环输出。例如,您可以将语录存储在一个字符串变量中,然后使用for
循环打印该语录十次。以下是一个简单的示例代码:
quote = "生活就像骑自行车,要保持平衡就得不断前行。"
for _ in range(10):
print(quote)
是否可以使用其他循环结构实现相同的效果?
当然可以,除了for
循环,您还可以使用while
循环来实现相同的功能。您可以通过一个计数器变量来控制循环的次数。以下是使用while
循环的示例:
quote = "生活就像骑自行车,要保持平衡就得不断前行。"
count = 0
while count < 10:
print(quote)
count += 1
如何使输出的语录更具吸引力?
可以通过添加时间间隔或改变输出格式来让语录的输出更加吸引人。您可以使用time
模块中的sleep
函数来在每次输出之间添加延迟。以下是一个示例:
import time
quote = "生活就像骑自行车,要保持平衡就得不断前行。"
for _ in range(10):
print(quote)
time.sleep(1) # 每次输出之间暂停1秒
使用不同的打印样式或颜色,也可以使输出更具吸引力。
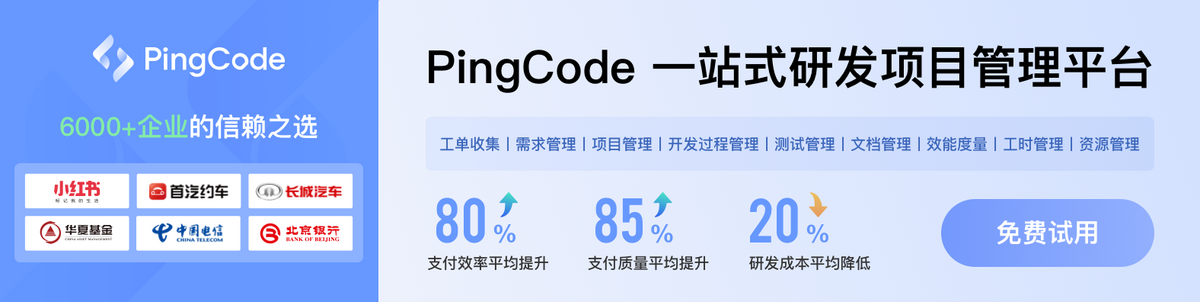