在Python中统计某一类字符的方法有很多种,包括使用内置函数、正则表达式、循环等。以下是一些常用的方法:使用字符串方法count()、使用循环遍历字符串、使用正则表达式等。
使用字符串方法count()是最简单的方法之一,它可以快速统计字符串中某个字符出现的次数。使用循环遍历字符串可以更加灵活地统计不同条件下的字符。使用正则表达式可以匹配更复杂的字符模式,适用于更高级的需求。下面将详细介绍这些方法及其使用场景。
一、使用字符串方法count()
字符串方法count()
是Python内置的一个方法,可以统计字符串中某个字符或子字符串出现的次数。这种方法非常简洁和高效。
string = "hello world"
char = 'o'
count = string.count(char)
print(f"'{char}' appears {count} times in the string.")
在上面的例子中,我们统计了字符'o'在字符串"hello world"中出现的次数。输出结果是:'o' appears 2 times in the string.
二、使用循环遍历字符串
使用循环遍历字符串可以更加灵活地统计不同条件下的字符。例如,我们可以统计元音字母的出现次数。
string = "hello world"
vowels = "aeiou"
count = 0
for char in string:
if char in vowels:
count += 1
print(f"There are {count} vowels in the string.")
在这个例子中,我们遍历字符串中的每个字符,并检查它是否是元音。如果是,则计数器count
加1。最终输出结果是:There are 3 vowels in the string.
三、使用正则表达式
正则表达式(regex)是一个强大的工具,可以用于匹配复杂的字符模式。Python的re
模块提供了对正则表达式的支持。
import re
string = "hello world"
pattern = r'[aeiou]'
matches = re.findall(pattern, string)
count = len(matches)
print(f"There are {count} vowels in the string.")
在这个例子中,我们使用正则表达式[aeiou]
来匹配所有的元音字母。re.findall()
函数返回所有匹配的字符,len()
函数计算匹配的数量。最终输出结果是:There are 3 vowels in the string.
四、统计不同类型的字符
有时候,我们需要统计不同类型的字符,例如字母、数字、空格等。下面是一个示例,统计字符串中的字母和数字的数量。
string = "hello world 123"
letters = 0
digits = 0
for char in string:
if char.isalpha():
letters += 1
elif char.isdigit():
digits += 1
print(f"There are {letters} letters and {digits} digits in the string.")
在这个例子中,我们使用isalpha()
方法检查字符是否是字母,使用isdigit()
方法检查字符是否是数字。最终输出结果是:There are 10 letters and 3 digits in the string.
五、使用collections.Counter
collections
模块中的Counter
类是一个字典子类,用于计数可哈希对象。它可以方便地统计字符出现的次数。
from collections import Counter
string = "hello world"
counter = Counter(string)
print(counter)
在这个例子中,Counter
会统计字符串中每个字符出现的次数,并以字典的形式返回结果。输出结果是:Counter({'l': 3, 'o': 2, 'h': 1, 'e': 1, ' ': 1, 'w': 1, 'r': 1, 'd': 1})
六、统计特定条件下的字符
我们还可以根据特定条件统计字符,例如统计大写字母、小写字母等。
string = "Hello World"
uppercase = 0
lowercase = 0
for char in string:
if char.isupper():
uppercase += 1
elif char.islower():
lowercase += 1
print(f"There are {uppercase} uppercase and {lowercase} lowercase letters in the string.")
在这个例子中,我们使用isupper()
方法检查字符是否是大写字母,使用islower()
方法检查字符是否是小写字母。最终输出结果是:There are 2 uppercase and 8 lowercase letters in the string.
七、统计字符频率并排序
有时候,我们不仅需要统计字符的数量,还需要按频率对字符进行排序。
from collections import Counter
string = "hello world"
counter = Counter(string)
sorted_counter = sorted(counter.items(), key=lambda item: item[1], reverse=True)
for char, count in sorted_counter:
print(f"'{char}': {count} times")
在这个例子中,我们首先使用Counter
统计字符出现的次数,然后使用sorted()
函数按频率降序排序。最终输出结果是每个字符及其出现的次数,按频率从高到低排列。
八、统计字符类型比例
在某些应用场景中,我们可能需要统计字符类型的比例,例如字母、数字、空格等占总字符数的比例。
string = "hello world 123"
total_chars = len(string)
letters = sum(c.isalpha() for c in string)
digits = sum(c.isdigit() for c in string)
spaces = sum(c.isspace() for c in string)
letter_ratio = letters / total_chars
digit_ratio = digits / total_chars
space_ratio = spaces / total_chars
print(f"Letters: {letter_ratio:.2%}, Digits: {digit_ratio:.2%}, Spaces: {space_ratio:.2%}")
在这个例子中,我们使用列表生成式和内置方法分别统计字母、数字和空格的数量,然后计算它们占总字符数的比例。最终输出结果是每种字符类型的比例。
九、统计多种字符类型
我们还可以统计多种字符类型,例如统计字符串中的元音、辅音、数字和其他字符。
string = "hello world 123"
vowels = "aeiou"
vowel_count = 0
consonant_count = 0
digit_count = 0
other_count = 0
for char in string:
if char in vowels:
vowel_count += 1
elif char.isalpha():
consonant_count += 1
elif char.isdigit():
digit_count += 1
else:
other_count += 1
print(f"Vowels: {vowel_count}, Consonants: {consonant_count}, Digits: {digit_count}, Others: {other_count}")
在这个例子中,我们分别统计了元音、辅音、数字和其他字符的数量。最终输出结果是每种字符类型的数量。
十、综合示例
最后,我们可以将以上方法结合起来,创建一个综合示例,统计字符串中的各种字符类型及其频率。
import re
from collections import Counter
def analyze_string(string):
total_chars = len(string)
letters = sum(c.isalpha() for c in string)
digits = sum(c.isdigit() for c in string)
spaces = sum(c.isspace() for c in string)
vowels = sum(c in 'aeiou' for c in string)
consonants = letters - vowels
other_chars = total_chars - letters - digits - spaces
letter_ratio = letters / total_chars
digit_ratio = digits / total_chars
space_ratio = spaces / total_chars
other_ratio = other_chars / total_chars
counter = Counter(string)
sorted_counter = sorted(counter.items(), key=lambda item: item[1], reverse=True)
print(f"Total characters: {total_chars}")
print(f"Letters: {letters} ({letter_ratio:.2%})")
print(f"Digits: {digits} ({digit_ratio:.2%})")
print(f"Spaces: {spaces} ({space_ratio:.2%})")
print(f"Vowels: {vowels}")
print(f"Consonants: {consonants}")
print(f"Other characters: {other_chars} ({other_ratio:.2%})")
print("\nCharacter frequencies:")
for char, count in sorted_counter:
print(f"'{char}': {count} times")
string = "Hello World 123"
analyze_string(string)
在这个综合示例中,我们统计了字符串中的总字符数、字母、数字、空格、元音、辅音和其他字符的数量及其比例,并按频率输出每个字符的出现次数。最终输出结果非常详细,适用于更复杂的字符分析需求。
通过以上方法,我们可以轻松统计Python中某一类字符的数量,并根据不同需求进行灵活应用。希望这些方法对你有所帮助。
相关问答FAQs:
如何在Python中统计特定字符的出现次数?
在Python中,可以使用字符串的count()
方法来统计特定字符的出现次数。例如,如果你想统计字符'a'在字符串中的次数,可以使用以下代码:
my_string = "banana"
count_a = my_string.count('a')
print(count_a) # 输出 3
这种方法简单易用,适合处理小型字符串。
是否可以在Python中统计多个字符的出现次数?
当然可以!可以通过循环遍历字符列表,并使用count()
方法来统计每个字符的出现次数。以下是一个示例:
my_string = "banana"
characters = ['a', 'b', 'n']
counts = {char: my_string.count(char) for char in characters}
print(counts) # 输出 {'a': 3, 'b': 1, 'n': 2}
这种方式能够高效地处理多个字符的统计需求。
在处理大字符串时,如何提高统计字符的效率?
对于较大的字符串,使用collections.Counter
是一个不错的选择。它能够快速统计所有字符的出现频率。示例代码如下:
from collections import Counter
my_string = "banana"
counter = Counter(my_string)
print(counter) # 输出 Counter({'a': 3, 'b': 1, 'n': 2})
这种方法不仅高效,而且可以方便地获取每个字符的频率,适合处理大量数据。
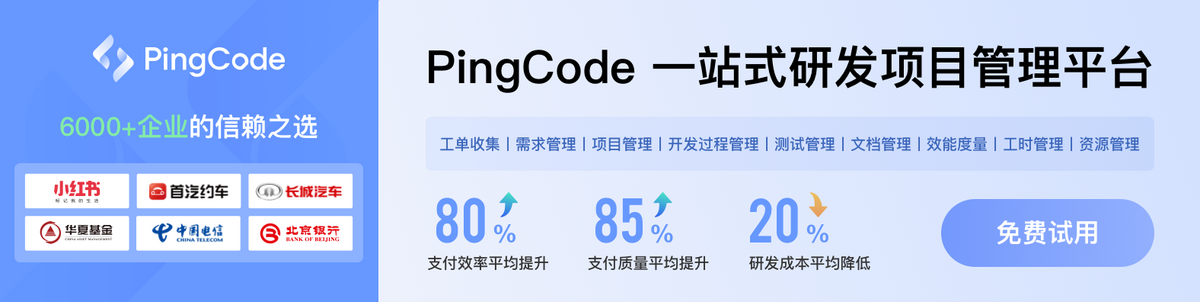