要提取Python中的第10行数据,可以使用以下几种方法:使用文件读写操作、使用Pandas库、或者使用Numpy库。在处理文本文件时,可以使用标准文件操作来读取指定行、对于大规模数据处理,Pandas库是一个强大的工具、Numpy库也提供了便捷的方法来处理数组和矩阵。下面将详细解释这三种方法,并且展示每种方法的具体实现代码。
一、使用标准文件操作读取第10行数据
Python的标准库提供了文件操作的基本功能,可以很方便地读取和操作文本文件。我们可以使用 open()
函数打开文件,并使用 readlines()
方法读取所有行,然后提取第10行数据。
def read_10th_line(file_path):
with open(file_path, 'r') as file:
lines = file.readlines()
if len(lines) >= 10:
return lines[9].strip() # 索引从0开始,第10行是索引9
else:
return None
file_path = 'example.txt'
tenth_line = read_10th_line(file_path)
print(f'The 10th line is: {tenth_line}')
二、使用Pandas库读取第10行数据
Pandas是Python中非常强大的数据处理和分析库,特别适用于处理结构化数据。可以使用 read_csv()
或 read_excel()
等方法来读取文件,并通过行索引来提取第10行数据。
import pandas as pd
def read_10th_line_csv(file_path):
df = pd.read_csv(file_path)
if len(df) >= 10:
return df.iloc[9] # 索引从0开始,第10行是索引9
else:
return None
file_path = 'example.csv'
tenth_line = read_10th_line_csv(file_path)
print(f'The 10th line is: {tenth_line}')
三、使用Numpy库读取第10行数据
Numpy是Python中用于科学计算的库,提供了对大型多维数组和矩阵的支持。对于矩阵形式的数据,可以使用 numpy.loadtxt()
或 numpy.genfromtxt()
方法读取文件,并通过索引提取第10行数据。
import numpy as np
def read_10th_line_numpy(file_path):
data = np.loadtxt(file_path, delimiter=',')
if data.shape[0] >= 10:
return data[9] # 索引从0开始,第10行是索引9
else:
return None
file_path = 'example.csv'
tenth_line = read_10th_line_numpy(file_path)
print(f'The 10th line is: {tenth_line}')
四、使用迭代器读取第10行数据
对于大文件,如果不想将文件全部加载到内存中,可以使用迭代器逐行读取文件,这样可以避免内存占用过高的问题。
def read_10th_line_iterator(file_path):
with open(file_path, 'r') as file:
for i, line in enumerate(file):
if i == 9: # 索引从0开始,第10行是索引9
return line.strip()
return None
file_path = 'example.txt'
tenth_line = read_10th_line_iterator(file_path)
print(f'The 10th line is: {tenth_line}')
五、处理大数据文件
在处理大数据文件时,尽量避免一次性读取整个文件,因为这可能会导致内存溢出。这时候可以考虑使用 pandas
的 chunksize
参数,分块读取文件。
import pandas as pd
def read_10th_line_large_csv(file_path):
chunk_size = 1000 # 每次读取1000行
chunk_iter = pd.read_csv(file_path, chunksize=chunk_size)
total_lines = 0
for chunk in chunk_iter:
if total_lines + len(chunk) >= 10:
return chunk.iloc[9 - total_lines]
total_lines += len(chunk)
return None
file_path = 'large_example.csv'
tenth_line = read_10th_line_large_csv(file_path)
print(f'The 10th line is: {tenth_line}')
六、处理Excel文件
对于Excel文件,可以使用 pandas
中的 read_excel
方法来读取数据,并提取第10行数据。
import pandas as pd
def read_10th_line_excel(file_path, sheet_name='Sheet1'):
df = pd.read_excel(file_path, sheet_name=sheet_name)
if len(df) >= 10:
return df.iloc[9] # 索引从0开始,第10行是索引9
else:
return None
file_path = 'example.xlsx'
tenth_line = read_10th_line_excel(file_path)
print(f'The 10th line is: {tenth_line}')
七、总结
在Python中提取第10行数据的方法有很多,选择哪种方法取决于具体的需求和数据文件类型。对于简单的文本文件,标准文件操作已经足够、对于结构化数据,Pandas库提供了强大的数据处理能力、对于科学计算和数组操作,Numpy库是一个理想的选择。在处理大数据文件时,使用迭代器或分块读取的方法可以有效地避免内存溢出问题。
希望这篇文章能帮助你在Python中灵活地提取第10行数据,并为你的数据处理工作提供一些有用的参考。
相关问答FAQs:
如何在Python中读取特定行的数据?
在Python中,可以使用多种方法读取特定行的数据。例如,使用内置的open()
函数结合readlines()
方法,可以将文件中的所有行加载到一个列表中,然后通过索引直接访问所需的行。以下是一个示例代码,读取文件中的第10行数据:
with open('文件名.txt', 'r') as file:
lines = file.readlines()
tenth_line = lines[9] # 索引从0开始,9对应第10行
print(tenth_line)
在大型文件中提取第10行数据的方法有哪些?
处理大型文件时,使用readlines()
可能会消耗过多内存。可以逐行读取文件,直到找到第10行。在这种情况下,使用enumerate()
函数会非常方便。示例代码如下:
with open('文件名.txt', 'r') as file:
for index, line in enumerate(file):
if index == 9: # 找到第10行
print(line)
break
有什么库可以帮助提取文件中的特定行数据?
除了使用内置函数,Python还有一些强大的库可以帮助实现这一功能。例如,使用pandas
库,您可以轻松地读取文件并提取特定行。首先,需要安装pandas
库,然后可以使用如下代码:
import pandas as pd
data = pd.read_csv('文件名.csv') # 或者使用read_excel()读取Excel文件
tenth_row = data.iloc[9] # 提取第10行
print(tenth_row)
这种方法不仅能提取特定行,还可以进行数据分析和处理。
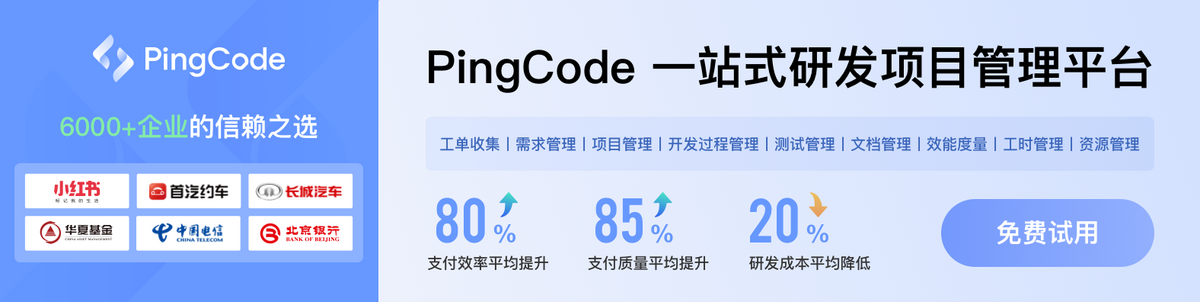