使用Python爬取游戏网站的方法有很多,主要包括:选择合适的爬虫库、分析网页结构、发送请求获取网页内容、解析并提取所需数据、处理反爬机制。 本文将详细介绍如何使用Python爬取游戏网站,并提供一步步的指导。
一、选择合适的爬虫库
Python有许多强大的爬虫库,如BeautifulSoup、Scrapy、Requests、Selenium等。每个库都有其独特的功能和适用场景。对于初学者来说,BeautifulSoup和Requests是比较容易上手的组合,而Scrapy则更适合复杂的爬虫任务。Selenium则适用于处理需要模拟浏览器行为的动态网页。
Requests库用于发送HTTP请求,BeautifulSoup用于解析HTML内容。
import requests
from bs4 import BeautifulSoup
url = "https://example.com"
response = requests.get(url)
soup = BeautifulSoup(response.content, "html.parser")
二、分析网页结构
在进行网页爬取之前,必须对目标网站的结构进行分析。这包括查看网页的HTML源代码,确定所需数据所在的标签和属性。可以使用浏览器的开发者工具(如Chrome的F12功能)来查看网页结构。
例如,假设我们需要爬取一个游戏网站的游戏列表,每个游戏的名称和链接。
<div class="game-list">
<a href="/game1" class="game-title">Game 1</a>
<a href="/game2" class="game-title">Game 2</a>
<a href="/game3" class="game-title">Game 3</a>
</div>
三、发送请求获取网页内容
使用Requests库发送HTTP请求,获取网页内容。可以通过URL、请求头等参数进行定制。
url = "https://example.com/games"
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"
}
response = requests.get(url, headers=headers)
注意设置请求头,避免被网站的反爬机制检测到。
四、解析并提取所需数据
使用BeautifulSoup解析HTML内容,并提取所需的数据。根据分析的网页结构,使用合适的选择器提取数据。
soup = BeautifulSoup(response.content, "html.parser")
games = soup.find_all("a", class_="game-title")
for game in games:
name = game.text
link = game["href"]
print(f"Game Name: {name}, Link: {link}")
通过标签名称和属性选择器,可以精准地提取所需数据。
五、处理反爬机制
很多网站都有反爬机制,如IP封禁、验证码、动态加载内容等。处理这些机制需要更多的技巧和工具。
- 设置请求头和代理: 模拟浏览器请求,使用代理IP避免被封禁。
proxies = {
"http": "http://10.10.1.10:3128",
"https": "http://10.10.1.10:1080",
}
response = requests.get(url, headers=headers, proxies=proxies)
- 处理动态内容: 使用Selenium模拟浏览器行为,获取动态加载的内容。
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("https://example.com/games")
page_source = driver.page_source
soup = BeautifulSoup(page_source, "html.parser")
继续解析提取数据
driver.quit()
通过Selenium可以模拟用户的浏览器操作,处理需要JavaScript渲染的动态内容。
六、存储爬取的数据
爬取到的数据可以存储到数据库、文件等。常用的存储方式包括CSV文件、JSON文件、SQLite数据库等。
import csv
with open("games.csv", "w", newline="") as csvfile:
fieldnames = ["name", "link"]
writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
writer.writeheader()
for game in games:
writer.writerow({"name": game.text, "link": game["href"]})
通过CSV文件存储数据,方便后续的数据分析和处理。
七、完整示例
以下是一个完整的示例代码,用于爬取一个游戏网站的游戏列表,并存储到CSV文件中。
import requests
from bs4 import BeautifulSoup
import csv
url = "https://example.com/games"
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3"
}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.content, "html.parser")
games = soup.find_all("a", class_="game-title")
with open("games.csv", "w", newline="") as csvfile:
fieldnames = ["name", "link"]
writer = csv.DictWriter(csvfile, fieldnames=fieldnames)
writer.writeheader()
for game in games:
writer.writerow({"name": game.text, "link": game["href"]})
通过上述步骤,可以使用Python爬取游戏网站,提取所需的数据并进行存储。根据具体需求,调整和扩展代码,以满足不同的爬取需求。
八、提高爬虫效率和稳定性
- 多线程和异步爬取: 使用多线程或异步IO技术,提高爬取效率。
import threading
def fetch_game_data(url):
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.content, "html.parser")
games = soup.find_all("a", class_="game-title")
for game in games:
print(f"Game Name: {game.text}, Link: {game['href']}")
urls = ["https://example.com/games/page1", "https://example.com/games/page2"]
threads = []
for url in urls:
thread = threading.Thread(target=fetch_game_data, args=(url,))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
- 错误处理和重试机制: 处理网络请求中的错误,并设置重试机制,提高爬虫的稳定性。
import time
from requests.exceptions import RequestException
def fetch_with_retries(url, retries=3):
for _ in range(retries):
try:
response = requests.get(url, headers=headers)
response.raise_for_status()
return response
except RequestException as e:
print(f"Error: {e}. Retrying...")
time.sleep(2)
return None
url = "https://example.com/games"
response = fetch_with_retries(url)
if response:
soup = BeautifulSoup(response.content, "html.parser")
# 继续处理数据
九、遵守法律法规和网站的robots.txt协议
在进行网页爬取时,必须遵守相关法律法规和网站的robots.txt协议。不要滥用爬虫技术,避免对网站造成负担或侵害网站的利益。
在爬取之前,可以检查网站的robots.txt文件,了解爬取规则。
url = "https://example.com/robots.txt"
response = requests.get(url)
print(response.text)
通过阅读robots.txt文件,可以了解哪些页面允许爬取,哪些页面禁止爬取,并遵守这些规则。
十、定期维护和更新爬虫
网页结构和内容会不断变化,爬虫代码也需要定期维护和更新。定期检查目标网站的变化,更新爬虫代码,以确保数据的准确性和完整性。
通过日志记录和监控,及时发现问题并进行修复。
import logging
logging.basicConfig(filename="crawler.log", level=logging.INFO)
logging.info("Starting crawler")
try:
# 爬虫代码
logging.info("Crawling completed successfully")
except Exception as e:
logging.error(f"Error occurred: {e}")
通过日志记录,可以跟踪爬虫的运行状态,发现并解决问题。
总结
使用Python爬取游戏网站涉及多个步骤,包括选择爬虫库、分析网页结构、发送请求、解析数据、处理反爬机制、存储数据、提高效率和稳定性、遵守法律法规、定期维护和更新。通过合理的设计和实现,可以高效地从目标网站中提取所需数据。希望本文的详细介绍和示例代码能帮助你更好地理解和掌握网页爬取技术。
相关问答FAQs:
如何选择合适的Python库来爬取游戏网站?
在爬取游戏网站时,选择合适的Python库至关重要。常用的库包括Requests和Beautiful Soup,前者用于发送HTTP请求,后者则用于解析HTML文档。如果需要处理动态加载的内容,可以考虑使用Selenium或Scrapy,这些库能够更好地处理JavaScript生成的页面。根据具体网站的结构和需求,选择最适合的工具会使爬取过程更加顺利。
在爬取游戏网站时需要注意哪些法律和道德问题?
在进行数据爬取时,必须遵循网站的robots.txt文件和相关法律法规。确保不违反网站的使用条款,避免对服务器造成过大压力。此外,尊重用户隐私和数据使用政策也非常重要。如果只是为了个人学习或研究,通常影响较小,但商业用途时则需更加谨慎。
如何处理爬取过程中遇到的反爬虫机制?
许多游戏网站会实施反爬虫措施,以保护其内容和用户数据。应对这些机制的方法包括使用代理服务器、修改请求头信息、增加请求间隔时间以及模拟用户行为等。通过这些技术手段,可以有效降低被封禁的风险,确保爬取工作顺利进行。
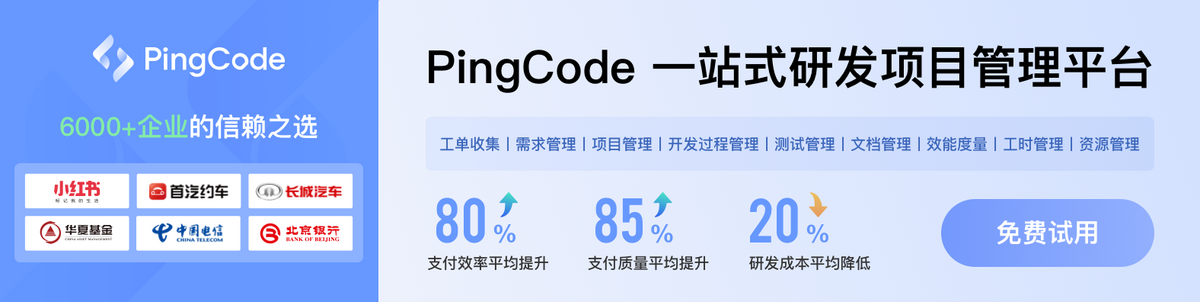