Python爬虫找到标签的属性值的步骤、利用BeautifulSoup解析HTML、使用XPath定位标签、通过正则表达式匹配属性值,这些都是实现这一任务的关键。下面,我们将详细探讨每一个步骤,并提供示例代码来帮助你更好地理解和应用这些技术。
一、利用BeautifulSoup解析HTML
BeautifulSoup是Python中一个非常流行的库,用于从HTML和XML文件中提取数据。它提供了一些简单的、直观的语法来导航、搜索和修改解析树。
要使用BeautifulSoup解析HTML,你需要首先安装并导入BeautifulSoup库。你可以使用以下命令安装BeautifulSoup:
pip install beautifulsoup4
然后,你可以使用以下代码解析HTML文件:
from bs4 import BeautifulSoup
html_doc = """
<html>
<head>
<title>The Dormouse's story</title>
</head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
</body>
</html>
"""
soup = BeautifulSoup(html_doc, 'html.parser')
解析完HTML后,你可以使用BeautifulSoup提供的方法找到标签,并提取其属性值。以下是一些示例:
- 查找所有链接并打印其href属性:
for link in soup.find_all('a'):
print(link.get('href'))
- 查找带有特定类的标签并打印其文本内容:
for tag in soup.find_all(class_='sister'):
print(tag.string)
二、使用XPath定位标签
XPath是一种用于在XML文档中定位节点的语言。它提供了一种非常强大的方式来导航和选择HTML文档中的节点。在Python中,你可以使用lxml库来处理XPath。
要使用lxml,你需要首先安装并导入lxml库。你可以使用以下命令安装lxml:
pip install lxml
然后,你可以使用以下代码解析HTML文件并使用XPath定位标签:
from lxml import html
html_doc = """
<html>
<head>
<title>The Dormouse's story</title>
</head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
</body>
</html>
"""
tree = html.fromstring(html_doc)
解析完HTML后,你可以使用XPath表达式来定位标签并提取其属性值。以下是一些示例:
- 查找所有链接并打印其href属性:
links = tree.xpath('//a/@href')
for link in links:
print(link)
- 查找带有特定类的标签并打印其文本内容:
texts = tree.xpath('//a[@class="sister"]/text()')
for text in texts:
print(text)
三、通过正则表达式匹配属性值
正则表达式是一种用于匹配文本模式的工具。在Python中,你可以使用re模块来处理正则表达式。
要使用正则表达式匹配属性值,你需要首先导入re模块。以下是一些示例代码:
import re
html_doc = """
<html>
<head>
<title>The Dormouse's story</title>
</head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
</body>
</html>
"""
查找所有href属性
hrefs = re.findall(r'href="(.*?)"', html_doc)
for href in hrefs:
print(href)
查找带有特定类的标签并打印其文本内容
texts = re.findall(r'<a.*?class="sister".*?>(.*?)</a>', html_doc)
for text in texts:
print(text)
通过以上三种方法,你可以轻松找到标签的属性值,并提取你需要的数据。根据具体的需求和HTML结构选择合适的方法,可以提高你的爬虫效率和准确性。
四、解析复杂HTML结构
在实际应用中,HTML结构可能会非常复杂,这时我们需要结合多种方法来解析HTML并提取数据。
- 结合BeautifulSoup和正则表达式
有时,HTML结构可能会非常混乱,这时可以结合BeautifulSoup和正则表达式来提取数据。例如:
from bs4 import BeautifulSoup
import re
html_doc = """
<html>
<head>
<title>The Dormouse's story</title>
</head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
</body>
</html>
"""
soup = BeautifulSoup(html_doc, 'html.parser')
使用BeautifulSoup找到所有链接
links = soup.find_all('a', class_='sister')
使用正则表达式提取每个链接的id属性
for link in links:
match = re.search(r'id="(.*?)"', str(link))
if match:
print(match.group(1))
- 结合lxml和正则表达式
你也可以结合lxml和正则表达式来处理复杂的HTML结构。例如:
from lxml import html
import re
html_doc = """
<html>
<head>
<title>The Dormouse's story</title>
</head>
<body>
<p class="title"><b>The Dormouse's story</b></p>
<p class="story">Once upon a time there were three little sisters; and their names were
<a href="http://example.com/elsie" class="sister" id="link1">Elsie</a>,
<a href="http://example.com/lacie" class="sister" id="link2">Lacie</a> and
<a href="http://example.com/tillie" class="sister" id="link3">Tillie</a>;
and they lived at the bottom of a well.</p>
<p class="story">...</p>
</body>
</html>
"""
tree = html.fromstring(html_doc)
使用XPath找到所有链接
links = tree.xpath('//a[@class="sister"]')
使用正则表达式提取每个链接的id属性
for link in links:
match = re.search(r'id="(.*?)"', html.tostring(link).decode('utf-8'))
if match:
print(match.group(1))
五、处理动态加载的内容
有时,网页内容是通过JavaScript动态加载的,这种情况下,单纯的HTML解析可能无法获取到所有的数据。这时可以使用Selenium等工具来处理动态加载的内容。
Selenium是一个自动化测试工具,可以用来模拟浏览器行为,并抓取动态加载的内容。要使用Selenium,你需要首先安装并导入Selenium库:
pip install selenium
然后,你可以使用以下代码来抓取动态加载的内容:
from selenium import webdriver
from selenium.webdriver.common.by import By
使用Chrome浏览器
driver = webdriver.Chrome()
打开网页
driver.get('http://example.com')
等待页面加载完成
driver.implicitly_wait(10)
查找所有链接并打印其href属性
links = driver.find_elements(By.TAG_NAME, 'a')
for link in links:
print(link.get_attribute('href'))
关闭浏览器
driver.quit()
通过以上步骤,你可以处理各种复杂的HTML结构和动态加载的内容,提取你需要的数据。无论是使用BeautifulSoup、lxml还是Selenium,都可以根据具体的需求和网页结构选择合适的工具和方法,提高爬虫的效率和准确性。
六、处理反爬虫机制
在实际应用中,很多网站都有反爬虫机制,比如通过检测用户代理、IP地址、请求频率等来阻止爬虫。这时,我们需要采取一些策略来绕过这些反爬虫机制。
- 设置用户代理
通过设置用户代理,可以模拟浏览器访问网页,从而绕过一些简单的反爬虫机制。以下是一些示例代码:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com'
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.content, 'html.parser')
查找所有链接并打印其href属性
for link in soup.find_all('a'):
print(link.get('href'))
- 使用代理IP
通过使用代理IP,可以隐藏真实IP地址,从而绕过一些基于IP地址的反爬虫机制。以下是一些示例代码:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com'
proxies = {
'http': 'http://10.10.10.10:8080',
'https': 'https://10.10.10.10:8080',
}
response = requests.get(url, proxies=proxies)
soup = BeautifulSoup(response.content, 'html.parser')
查找所有链接并打印其href属性
for link in soup.find_all('a'):
print(link.get('href'))
- 控制请求频率
通过控制请求频率,可以避免被网站检测到爬虫行为。可以使用time模块来设置请求间隔时间。以下是一些示例代码:
import requests
from bs4 import BeautifulSoup
import time
url = 'http://example.com'
for i in range(10):
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
# 查找所有链接并打印其href属性
for link in soup.find_all('a'):
print(link.get('href'))
# 设置请求间隔时间
time.sleep(2)
通过以上策略,可以有效绕过一些常见的反爬虫机制,提高爬虫的稳定性和成功率。
七、处理复杂的反爬虫机制
对于一些复杂的反爬虫机制,比如通过JavaScript检测用户行为、使用验证码等,我们可以采取更高级的策略来绕过这些机制。
- 使用Selenium模拟浏览器行为
Selenium可以模拟真实的浏览器行为,从而绕过一些基于JavaScript的反爬虫机制。以下是一些示例代码:
from selenium import webdriver
from selenium.webdriver.common.by import By
使用Chrome浏览器
driver = webdriver.Chrome()
打开网页
driver.get('http://example.com')
等待页面加载完成
driver.implicitly_wait(10)
查找所有链接并打印其href属性
links = driver.find_elements(By.TAG_NAME, 'a')
for link in links:
print(link.get_attribute('href'))
关闭浏览器
driver.quit()
- 使用打码平台处理验证码
对于一些需要输入验证码的网站,可以使用打码平台来自动识别和输入验证码。以下是一些示例代码:
from selenium import webdriver
from selenium.webdriver.common.by import By
使用Chrome浏览器
driver = webdriver.Chrome()
打开网页
driver.get('http://example.com')
等待页面加载完成
driver.implicitly_wait(10)
查找验证码图片并保存
captcha_image = driver.find_element(By.ID, 'captcha_image')
captcha_image.screenshot('captcha.png')
使用打码平台识别验证码
captcha_code = recognize_captcha('captcha.png')
输入验证码
captcha_input = driver.find_element(By.ID, 'captcha_input')
captcha_input.send_keys(captcha_code)
提交表单
submit_button = driver.find_element(By.ID, 'submit_button')
submit_button.click()
查找所有链接并打印其href属性
links = driver.find_elements(By.TAG_NAME, 'a')
for link in links:
print(link.get_attribute('href'))
关闭浏览器
driver.quit()
通过以上步骤,可以处理各种复杂的反爬虫机制,提高爬虫的成功率和稳定性。无论是使用BeautifulSoup、lxml、Selenium还是其他工具,都可以根据具体的需求和网页结构选择合适的工具和方法,提高爬虫的效率和准确性。
相关问答FAQs:
如何使用Python爬虫提取网页中的特定标签属性值?
在使用Python爬虫提取网页数据时,可以使用库如BeautifulSoup和Requests来获取和解析网页内容。通过BeautifulSoup,你可以轻松找到特定标签并提取其属性值。比如,使用find()
或find_all()
方法定位标签,然后通过标签对象访问属性,例如tag['attribute_name']
获取属性值。
使用爬虫时如何处理动态加载的内容?
许多网页使用JavaScript动态加载内容,这可能使得传统的爬虫工具难以获取数据。在这种情况下,可以使用Selenium等工具模拟浏览器行为,等待页面加载完成后再提取所需的标签属性值。Selenium允许你执行JavaScript并与页面进行交互,从而获取动态生成的数据。
在爬虫过程中如何避免被网站封禁?
为了避免被网站封禁,建议使用一些策略,如设置合理的请求间隔,使用代理IP和更改User-Agent。在爬虫代码中,可以随机设置请求头,模仿正常用户的访问行为。此外,遵循robots.txt文件中的规则也是非常重要的,确保你的爬虫行为符合网站的规定,从而减少被封禁的风险。
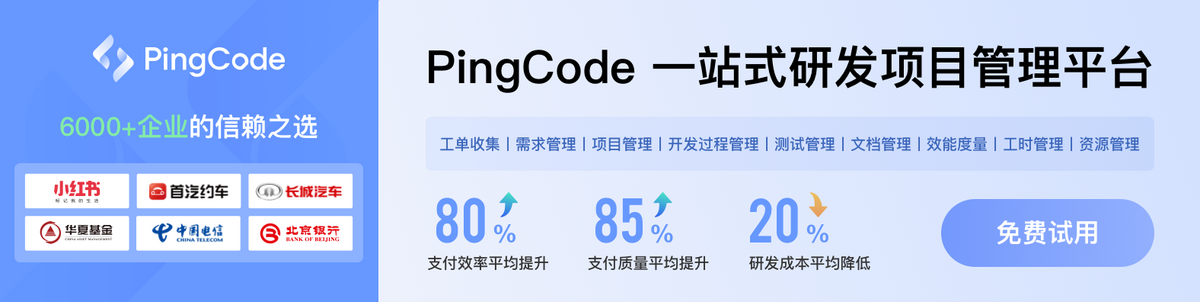