在Python中,字符串可以通过单引号、双引号、三重引号表示。例如:'Hello'、"Hello"、'''Hello'''、"""Hello"""。单引号和双引号基本上是等价的、三重引号可用于表示多行字符串和包含引号的字符串、字符串是Python中的不可变类型。我们可以详细探讨单引号和双引号的互换性、三重引号的用途以及字符串的不可变性。
一、单引号和双引号
Python中的单引号和双引号可以互换使用,这使得在需要嵌套引号时变得更加方便。比如:
single_quote = 'Hello, World!'
double_quote = "Hello, World!"
nested_quote = 'He said, "Hello, World!"'
在上面的例子中,single_quote
和double_quote
表示相同的字符串内容。而nested_quote
展示了在字符串中嵌套使用引号的技巧。通过这种方式,可以避免使用转义字符,从而提高代码的可读性。
二、三重引号
三重引号(即三个连续的单引号或双引号)可以用于表示多行字符串或包含引号的字符串。比如:
multi_line = '''This is a string
that spans multiple
lines.'''
quote_in_string = """She said, "It's a beautiful day!" """
在上面的例子中,multi_line
是一个跨多行的字符串,而quote_in_string
展示了如何在字符串中嵌入单引号和双引号。三重引号不仅提高了代码的可读性,还提供了方便的方式来处理长文本。
三、字符串的不可变性
在Python中,字符串是不可变类型。这意味着一旦创建,字符串的内容就不能被修改。任何对字符串的修改操作都会创建一个新的字符串对象。比如:
original = "Hello"
modified = original + ", World!"
在上面的例子中,original
字符串保持不变,而modified
是一个新的字符串对象,其内容是original
和, World!
的组合。字符串的不可变性有助于提高程序的稳定性和安全性,因为它避免了意外修改共享字符串的风险。
四、字符串操作
Python提供了丰富的字符串操作函数和方法。以下是一些常用的字符串操作:
- 字符串拼接
可以使用+
操作符来拼接字符串:
str1 = "Hello"
str2 = "World"
result = str1 + ", " + str2 + "!"
print(result) # 输出:Hello, World!
- 字符串格式化
Python支持多种字符串格式化方法,包括旧式的%
格式化、新式的str.format()
方法和f-strings(格式化字符串字面量):
name = "Alice"
age = 30
旧式格式化
old_style = "Name: %s, Age: %d" % (name, age)
新式格式化
new_style = "Name: {}, Age: {}".format(name, age)
f-strings
f_string = f"Name: {name}, Age: {age}"
print(old_style) # 输出:Name: Alice, Age: 30
print(new_style) # 输出:Name: Alice, Age: 30
print(f_string) # 输出:Name: Alice, Age: 30
- 字符串切片
可以使用切片操作从字符串中提取子字符串:
text = "Hello, World!"
slice1 = text[0:5] # 'Hello'
slice2 = text[7:] # 'World!'
slice3 = text[-6:] # 'World!'
print(slice1) # 输出:Hello
print(slice2) # 输出:World!
print(slice3) # 输出:World!
- 字符串查找和替换
可以使用str.find()
和str.replace()
方法进行字符串查找和替换:
text = "Hello, World!"
index = text.find("World") # 返回 7
new_text = text.replace("World", "Python") # 'Hello, Python!'
print(index) # 输出:7
print(new_text) # 输出:Hello, Python!
- 字符串拆分和合并
可以使用str.split()
和str.join()
方法进行字符串拆分和合并:
text = "Hello, World!"
words = text.split(", ") # ['Hello', 'World!']
joined = " ".join(words) # 'Hello World!'
print(words) # 输出:['Hello', 'World!']
print(joined) # 输出:Hello World!
六、字符串的其他重要方法
- 去除空白
str.strip()
、str.lstrip()
和str.rstrip()
方法可以用来去除字符串两端的空白字符:
text = " Hello, World! "
stripped = text.strip() # 'Hello, World!'
left_stripped = text.lstrip() # 'Hello, World! '
right_stripped = text.rstrip() # ' Hello, World!'
print(stripped) # 输出:Hello, World!
print(left_stripped) # 输出:Hello, World!
print(right_stripped) # 输出: Hello, World!
- 字符串大小写转换
str.upper()
、str.lower()
、str.title()
和str.capitalize()
方法可以用来转换字符串的大小写:
text = "hello, world!"
upper = text.upper() # 'HELLO, WORLD!'
lower = text.lower() # 'hello, world!'
title = text.title() # 'Hello, World!'
capitalized = text.capitalize() # 'Hello, world!'
print(upper) # 输出:HELLO, WORLD!
print(lower) # 输出:hello, world!
print(title) # 输出:Hello, World!
print(capitalized) # 输出:Hello, world!
- 检查字符串内容
str.startswith()
、str.endswith()
和str.isdigit()
方法可以用来检查字符串的内容:
text = "Hello, World!"
starts_with_hello = text.startswith("Hello") # True
ends_with_world = text.endswith("World!") # True
is_digit = text.isdigit() # False
print(starts_with_hello) # 输出:True
print(ends_with_world) # 输出:True
print(is_digit) # 输出:False
七、字符串的编码和解码
在处理文本数据时,编码和解码是非常重要的。Python提供了str.encode()
和bytes.decode()
方法来进行编码和解码操作:
text = "Hello, World!"
encoded = text.encode("utf-8") # b'Hello, World!'
decoded = encoded.decode("utf-8") # 'Hello, World!'
print(encoded) # 输出:b'Hello, World!'
print(decoded) # 输出:Hello, World!
八、字符串与其他数据类型的转换
Python提供了多种方法将其他数据类型转换为字符串,或将字符串转换为其他数据类型:
- 将数字转换为字符串
可以使用str()
函数将数字转换为字符串:
number = 123
text = str(number) # '123'
print(text) # 输出:123
- 将字符串转换为数字
可以使用int()
和float()
函数将字符串转换为整数和浮点数:
text = "123"
number = int(text) # 123
floating_number = float(text) # 123.0
print(number) # 输出:123
print(floating_number) # 输出:123.0
- 将列表转换为字符串
可以使用str()
函数或str.join()
方法将列表转换为字符串:
lst = [1, 2, 3]
text = str(lst) # '[1, 2, 3]'
joined = ", ".join(map(str, lst)) # '1, 2, 3'
print(text) # 输出:[1, 2, 3]
print(joined) # 输出:1, 2, 3
- 将字符串转换为列表
可以使用list()
函数或str.split()
方法将字符串转换为列表:
text = "Hello, World!"
lst = list(text) # ['H', 'e', 'l', 'l', 'o', ',', ' ', 'W', 'o', 'r', 'l', 'd', '!']
split_lst = text.split(", ") # ['Hello', 'World!']
print(lst) # 输出:['H', 'e', 'l', 'l', 'o', ',', ' ', 'W', 'o', 'r', 'l', 'd', '!']
print(split_lst) # 输出:['Hello', 'World!']
九、字符串的常用库
Python提供了许多内置库和第三方库来处理字符串操作。以下是一些常用的库:
- re(正则表达式)
re
库提供了强大的正则表达式功能,用于复杂的字符串匹配和替换操作:
import re
text = "The quick brown fox jumps over the lazy dog."
pattern = r"\b\w{3}\b" # 匹配所有三个字符的单词
matches = re.findall(pattern, text)
print(matches) # 输出:['The', 'fox', 'the', 'dog']
- textwrap
textwrap
库提供了方便的文本包装和填充功能:
import textwrap
text = "The quick brown fox jumps over the lazy dog."
wrapped = textwrap.fill(text, width=20)
print(wrapped)
输出:
The quick brown fox
jumps over the lazy
dog.
- string
string
库提供了一些有用的常量和函数,例如字母表、数字和标点符号:
import string
letters = string.ascii_letters # 'abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ'
digits = string.digits # '0123456789'
punctuation = string.punctuation # '!"#$%&\'()*+,-./:;<=>?@[\\]^_`{|}~'
print(letters) # 输出:abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ
print(digits) # 输出:0123456789
print(punctuation) # 输出:!"#$%&'()*+,-./:;<=>?@[\\]^_`{|}~
十、实践示例
为了更好地理解Python中的字符串操作,我们可以通过一些实践示例来展示如何在实际应用中使用这些功能。
- 处理文本文件
假设我们有一个文本文件,需要读取其中的内容并进行一些处理:
filename = "example.txt"
读取文件内容
with open(filename, "r") as file:
content = file.read()
去除空白行
lines = content.split("\n")
non_empty_lines = [line for line in lines if line.strip() != ""]
将处理后的内容写回文件
with open(filename, "w") as file:
file.write("\n".join(non_empty_lines))
- 生成随机字符串
在一些应用中,我们可能需要生成随机字符串,例如验证码或密码:
import random
import string
def generate_random_string(length=8):
characters = string.ascii_letters + string.digits
return ''.join(random.choice(characters) for _ in range(length))
random_string = generate_random_string()
print(random_string) # 输出一个随机字符串,例如:'a1B2c3D4'
- 解析CSV文件
CSV(逗号分隔值)文件是常见的数据交换格式,我们可以使用Python字符串操作来解析CSV文件:
filename = "example.csv"
读取CSV文件内容
with open(filename, "r") as file:
content = file.read()
解析CSV内容
lines = content.split("\n")
header = lines[0].split(",")
data = [line.split(",") for line in lines[1:] if line.strip() != ""]
打印解析后的数据
print("Header:", header)
print("Data:", data)
- 处理JSON字符串
JSON(JavaScript对象表示法)是一种常见的数据交换格式,我们可以使用Python字符串操作来处理JSON字符串:
import json
json_string = '{"name": "Alice", "age": 30, "city": "New York"}'
解析JSON字符串
data = json.loads(json_string)
修改数据
data["age"] = 31
将数据转换为JSON字符串
modified_json_string = json.dumps(data)
print(modified_json_string) # 输出:{"name": "Alice", "age": 31, "city": "New York"}
通过这些实践示例,我们可以更好地理解和掌握Python中的字符串操作。这些操作在处理文本数据、生成随机字符串、解析文件内容等方面都非常实用。希望通过本文的介绍,你能够更熟练地使用Python进行字符串操作,提升编程效率和代码质量。
相关问答FAQs:
在Python中,如何定义一个字符串?
在Python中,字符串可以使用单引号(')或双引号(")来定义。例如,my_string = 'Hello, World!'
或 my_string = "Hello, World!"
都是有效的字符串定义方式。选择哪种引号通常取决于字符串内容中是否包含引号。
如何在Python中处理多行字符串?
对于多行字符串,可以使用三重引号(''' 或 """)来定义。这使得字符串可以跨越多行,而不需要使用换行符。例如:
my_multiline_string = '''这是第一行
这是第二行
这是第三行'''
这样的定义方式使得代码更具可读性。
在Python中,如何对字符串进行操作和修改?
Python提供了丰富的字符串方法来处理字符串。例如,可以使用len()
函数获取字符串长度,使用str.lower()
或str.upper()
方法转换大小写,使用str.replace(old, new)
方法替换字符。还可以使用切片(slicing)来提取字符串的一部分,比如my_string[0:5]
将返回字符串的前五个字符。通过这些方法,用户可以灵活地对字符串进行各种操作。
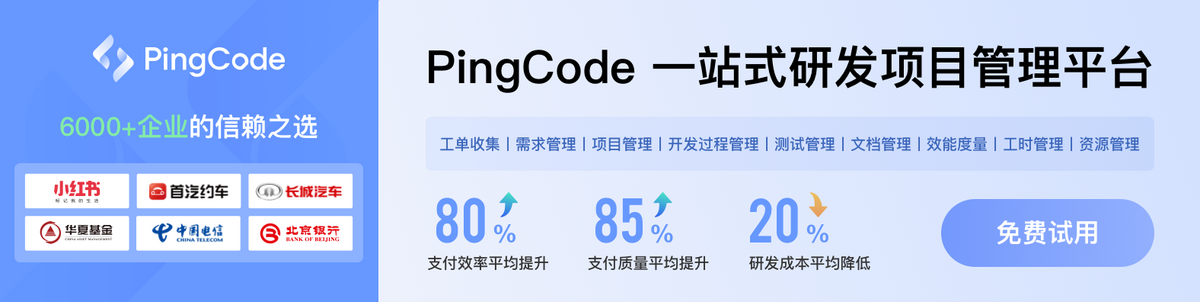