在Python编程中,制作静态钟表的关键步骤包括:设计钟表的界面、绘制钟表的刻度、绘制指针、设置时间、使用图形库。 其中,选择合适的图形库可以使绘制和显示变得更加方便和高效。接下来将详细描述如何使用Python和图形库来实现一个静态钟表。
一、选择图形库
在Python中,有多种图形库可以用来绘制静态钟表,其中最常用的是Matplotlib和Tkinter。Matplotlib是一种强大的绘图库,适合用于创建各种类型的图表和图形。Tkinter是Python的标准GUI库,适合用于创建用户界面和简单的图形。本文将使用Matplotlib来绘制静态钟表。
二、绘制钟表界面
- 导入必要的库:
首先,我们需要导入Matplotlib库以及其他必要的模块。
import matplotlib.pyplot as plt
import numpy as np
import math
- 设置绘图参数:
我们需要设置绘图的参数,例如图形的大小、背景颜色等。
fig, ax = plt.subplots(figsize=(6, 6), facecolor='white')
ax.set_facecolor('lightgray')
ax.set_aspect('equal')
ax.axis('off')
三、绘制钟表刻度
- 绘制时钟圆圈:
使用Matplotlib绘制一个代表时钟的圆圈。
circle = plt.Circle((0.5, 0.5), 0.4, edgecolor='black', facecolor='none', lw=2)
ax.add_artist(circle)
- 绘制时刻度和分刻度:
使用循环来绘制时刻度和分刻度。
for i in range(60):
angle = i * 6
x_outer = 0.5 + 0.4 * math.cos(math.radians(angle))
y_outer = 0.5 + 0.4 * math.sin(math.radians(angle))
if i % 5 == 0:
x_inner = 0.5 + 0.35 * math.cos(math.radians(angle))
y_inner = 0.5 + 0.35 * math.sin(math.radians(angle))
ax.plot([x_inner, x_outer], [y_inner, y_outer], color='black', lw=2)
else:
x_inner = 0.5 + 0.375 * math.cos(math.radians(angle))
y_inner = 0.5 + 0.375 * math.sin(math.radians(angle))
ax.plot([x_inner, x_outer], [y_inner, y_outer], color='black', lw=1)
四、绘制指针
- 绘制时针、分针和秒针:
根据当前时间计算指针的角度,并绘制相应的指针。
from datetime import datetime
now = datetime.now()
hour_angle = (now.hour % 12 + now.minute / 60) * 30
minute_angle = now.minute * 6
second_angle = now.second * 6
def draw_hand(angle, length, width, color):
x_end = 0.5 + length * math.cos(math.radians(angle))
y_end = 0.5 + length * math.sin(math.radians(angle))
ax.plot([0.5, x_end], [0.5, y_end], color=color, lw=width)
draw_hand(hour_angle, 0.2, 6, 'black')
draw_hand(minute_angle, 0.3, 4, 'blue')
draw_hand(second_angle, 0.35, 2, 'red')
五、显示钟表
- 显示绘制的静态钟表:
使用Matplotlib的show方法来显示绘制的钟表。
plt.show()
这样,一个简单的静态钟表就绘制完成了。
六、代码完整示例
以下是上述步骤的完整代码示例:
import matplotlib.pyplot as plt
import numpy as np
import math
from datetime import datetime
fig, ax = plt.subplots(figsize=(6, 6), facecolor='white')
ax.set_facecolor('lightgray')
ax.set_aspect('equal')
ax.axis('off')
circle = plt.Circle((0.5, 0.5), 0.4, edgecolor='black', facecolor='none', lw=2)
ax.add_artist(circle)
for i in range(60):
angle = i * 6
x_outer = 0.5 + 0.4 * math.cos(math.radians(angle))
y_outer = 0.5 + 0.4 * math.sin(math.radians(angle))
if i % 5 == 0:
x_inner = 0.5 + 0.35 * math.cos(math.radians(angle))
y_inner = 0.5 + 0.35 * math.sin(math.radians(angle))
ax.plot([x_inner, x_outer], [y_inner, y_outer], color='black', lw=2)
else:
x_inner = 0.5 + 0.375 * math.cos(math.radians(angle))
y_inner = 0.5 + 0.375 * math.sin(math.radians(angle))
ax.plot([x_inner, x_outer], [y_inner, y_outer], color='black', lw=1)
now = datetime.now()
hour_angle = (now.hour % 12 + now.minute / 60) * 30
minute_angle = now.minute * 6
second_angle = now.second * 6
def draw_hand(angle, length, width, color):
x_end = 0.5 + length * math.cos(math.radians(angle))
y_end = 0.5 + length * math.sin(math.radians(angle))
ax.plot([0.5, x_end], [0.5, y_end], color=color, lw=width)
draw_hand(hour_angle, 0.2, 6, 'black')
draw_hand(minute_angle, 0.3, 4, 'blue')
draw_hand(second_angle, 0.35, 2, 'red')
plt.show()
通过以上步骤,我们成功地用Python和Matplotlib绘制了一个静态钟表。这个静态钟表显示了当前的时间,并且可以通过调整代码中的参数来改变钟表的外观,例如更改背景颜色、刻度颜色、指针颜色等。这样,不仅掌握了如何绘制静态钟表,还可以根据自己的需求进行定制和调整。
相关问答FAQs:
如何使用Python创建一个简单的静态钟表?
在Python中,可以使用图形库如Tkinter或Pygame来创建静态钟表。通过绘制时钟的表盘、指针和数字,可以实现一个简单的静态钟表效果。Tkinter提供了一个便捷的方式来绘制图形,只需设置画布并使用绘图功能来绘制时钟的各个部分。
静态钟表的设计需要考虑哪些元素?
在设计静态钟表时,重要的元素包括钟表的外形(如圆形表盘)、时针、分针和秒针的长度和位置,以及数字或刻度的标记。选择合适的颜色和字体样式也能够提升钟表的美观度和可读性。
如何在静态钟表中显示当前时间?
为了在静态钟表中显示当前时间,可以使用Python的datetime模块获取当前时间。通过将获取的小时、分钟和秒数转换为适当的角度,可以计算指针的位置,从而在钟表上准确表示当前的时间。尽管是静态的,更新显示时间的机制可以通过定时刷新来实现。
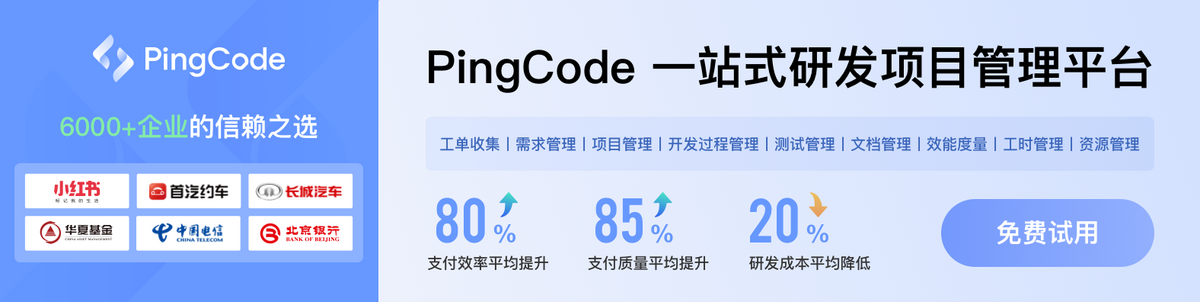