使用Python写一个网页,可以使用Web框架、使用Flask、使用Django、使用HTML模板、使用CSS样式、使用JavaScript。 其中,使用Flask框架是最常见的方法之一,因为它简单易用且功能强大。接下来,我将详细描述如何使用Flask创建一个基本的网页。
一、安装Flask
首先,你需要安装Flask。你可以使用以下命令通过pip进行安装:
pip install Flask
二、创建项目结构
接下来,你需要创建一个项目结构。一个典型的Flask项目结构如下:
my_flask_app/
├── app.py
├── templates/
│ └── index.html
└── static/
├── css/
│ └── style.css
└── js/
└── script.js
三、编写Flask应用
在app.py
文件中,你需要编写Flask应用的基本代码:
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
return render_template('index.html')
if __name__ == '__main__':
app.run(debug=True)
四、创建HTML模板
在templates
目录中,创建一个名为index.html
的文件。这个文件将作为网页的基本模板:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My Flask App</title>
<link rel="stylesheet" href="{{ url_for('static', filename='css/style.css') }}">
</head>
<body>
<h1>Welcome to My Flask App</h1>
<p>This is a basic example of a Flask app.</p>
<script src="{{ url_for('static', filename='js/script.js') }}"></script>
</body>
</html>
五、添加CSS样式
在static/css
目录中,创建一个名为style.css
的文件。这个文件将包含网页的CSS样式:
body {
font-family: Arial, sans-serif;
background-color: #f0f0f0;
color: #333;
}
h1 {
color: #007BFF;
}
六、添加JavaScript
在static/js
目录中,创建一个名为script.js
的文件。这个文件将包含网页的JavaScript代码:
document.addEventListener('DOMContentLoaded', function() {
console.log('JavaScript is working!');
});
七、运行Flask应用
最后,在终端中运行以下命令,启动Flask应用:
python app.py
打开浏览器,访问http://127.0.0.1:5000/
,你将看到你的网页。
八、扩展功能
到目前为止,你已经创建了一个基本的Flask网页。接下来可以考虑添加更多的功能和页面。
1、添加更多路由
你可以在app.py
中添加更多的路由来处理不同的URL。例如:
@app.route('/about')
def about():
return render_template('about.html')
然后在templates
目录中创建一个名为about.html
的文件:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>About</title>
<link rel="stylesheet" href="{{ url_for('static', filename='css/style.css') }}">
</head>
<body>
<h1>About Page</h1>
<p>This is the about page.</p>
<script src="{{ url_for('static', filename='js/script.js') }}"></script>
</body>
</html>
2、使用模板继承
模板继承是Flask的强大功能之一。它允许你创建一个基本的模板,并在多个页面中重用它。例如,创建一个名为base.html
的基本模板:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>{% block title %}My Flask App{% endblock %}</title>
<link rel="stylesheet" href="{{ url_for('static', filename='css/style.css') }}">
</head>
<body>
<header>
<h1>{% block header %}Welcome to My Flask App{% endblock %}</h1>
</header>
<main>
{% block content %}{% endblock %}
</main>
<script src="{{ url_for('static', filename='js/script.js') }}"></script>
</body>
</html>
然后在其他HTML文件中继承这个模板:
{% extends "base.html" %}
{% block title %}Home{% endblock %}
{% block header %}Home Page{% endblock %}
{% block content %}
<p>This is the home page content.</p>
{% endblock %}
3、处理表单提交
Flask允许你轻松处理表单提交。首先,在HTML模板中创建一个表单:
<form action="/submit" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<button type="submit">Submit</button>
</form>
然后在app.py
中添加一个路由来处理表单提交:
from flask import request
@app.route('/submit', methods=['POST'])
def submit():
name = request.form['name']
return f'Thank you, {name}!'
九、使用Flask扩展
Flask有许多扩展,可以帮助你添加更多的功能。以下是一些常用的Flask扩展:
1、Flask-WTF
Flask-WTF扩展使得表单处理变得更加容易。首先,通过pip安装Flask-WTF:
pip install Flask-WTF
然后在app.py
中使用Flask-WTF:
from flask_wtf import FlaskForm
from wtforms import StringField, SubmitField
from wtforms.validators import DataRequired
from flask import Flask, render_template, redirect, url_for
app = Flask(__name__)
app.config['SECRET_KEY'] = 'your_secret_key'
class NameForm(FlaskForm):
name = StringField('Name', validators=[DataRequired()])
submit = SubmitField('Submit')
@app.route('/', methods=['GET', 'POST'])
def home():
form = NameForm()
if form.validate_on_submit():
return redirect(url_for('thank_you', name=form.name.data))
return render_template('index.html', form=form)
@app.route('/thank_you/<name>')
def thank_you(name):
return f'Thank you, {name}!'
if __name__ == '__main__':
app.run(debug=True)
在index.html
中添加表单渲染:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My Flask App</title>
<link rel="stylesheet" href="{{ url_for('static', filename='css/style.css') }}">
</head>
<body>
<h1>Welcome to My Flask App</h1>
<form method="post">
{{ form.hidden_tag() }}
<p>
{{ form.name.label }}<br>
{{ form.name(size=32) }}<br>
{% for error in form.name.errors %}
<span style="color: red;">[{{ error }}]</span>
{% endfor %}
</p>
<p>{{ form.submit() }}</p>
</form>
<script src="{{ url_for('static', filename='js/script.js') }}"></script>
</body>
</html>
十、调试和部署
1、调试模式
在开发过程中,你可以启用调试模式,这样你可以看到错误消息,并在代码发生变化时自动重新加载应用:
if __name__ == '__main__':
app.run(debug=True)
2、部署到生产环境
在准备部署到生产环境时,记得关闭调试模式,并使用一个真正的Web服务器,例如Gunicorn:
pip install gunicorn
gunicorn -w 4 app:app
十一、总结
通过以上步骤,你已经了解了如何使用Python和Flask创建一个基本的网页。你可以进一步扩展应用,添加更多功能,例如数据库支持、用户认证和授权等。Flask的简洁和灵活性使得它成为Python Web开发的一个优秀选择。
相关问答FAQs:
如何使用Python创建一个简单的网页?
要使用Python创建一个简单的网页,您可以借助Flask或Django等框架。Flask是一个轻量级框架,非常适合初学者。您需要安装Flask,通过创建一个Python脚本,设置路由并返回HTML内容,便可以快速生成网页。例如,您可以定义一个路由,当访问特定URL时,返回一个简单的HTML页面。
使用Python开发网页时,推荐哪些框架?
对于网页开发,Flask和Django是两个非常流行的选择。Flask适合快速开发和小型项目,而Django则提供了更多的功能和结构,适合大型应用程序。根据项目需求和个人熟悉程度,您可以选择合适的框架。还有其他选择,如FastAPI和Pyramid,它们也适合不同的应用场景。
如何在Python网页中添加样式和交互功能?
可以通过HTML和CSS来为Python生成的网页添加样式。您可以将CSS文件链接到HTML中,使网页更加美观。同时,使用JavaScript可以实现交互功能,例如表单验证、动态内容更新等。将这些技术结合在一起,可以提升用户体验,创建更具吸引力和互动性的网页。
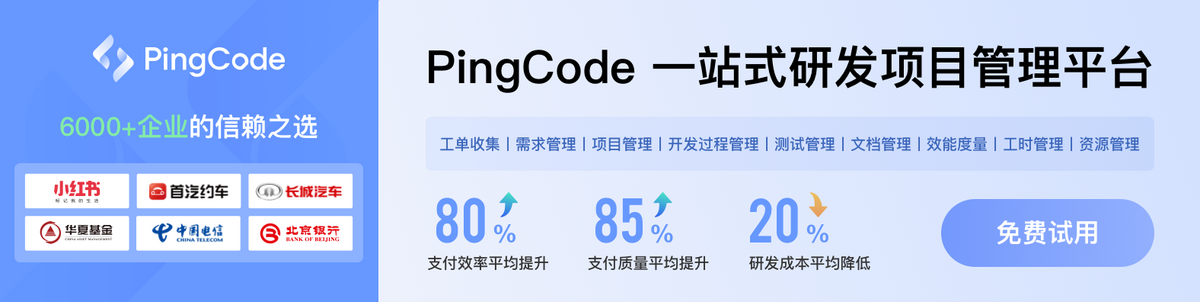