Python实现日期做大事记的方法有多种,使用字典数据结构、使用数据库、结合日历模块
详细描述:使用字典数据结构是一种简单且方便的方法。你可以使用字典存储日期和事件,其中日期作为键,事件作为值。这种方法适合于较小规模的数据存储和快速实现。举个例子,如果你想存储某个日期发生的事件,可以这样做:
events = {
"2023-10-01": "National Day",
"2023-12-25": "Christmas",
"2024-01-01": "New Year"
}
添加新事件
events["2024-02-14"] = "Valentine's Day"
打印某个日期的事件
print(events["2024-02-14"])
一、使用字典数据结构
使用字典来实现日期做大事记非常直观和简单。字典允许快速查找和更新事件,适合处理小规模的日期和事件数据。
创建字典
首先,我们需要创建一个字典来存储日期和事件。每个日期作为键,对应的事件作为值。
events = {
"2023-10-01": "National Day",
"2023-12-25": "Christmas",
"2024-01-01": "New Year"
}
添加事件
要向字典中添加新事件,可以直接使用日期作为键,并将事件描述作为值。
events["2024-02-14"] = "Valentine's Day"
检索和更新事件
可以通过日期键来检索和更新事件。例如,检索某个日期的事件:
print(events["2024-02-14"]) # 输出: Valentine's Day
更新某个日期的事件:
events["2024-02-14"] = "Updated Valentine's Day"
删除事件
如果某个事件不再需要,可以从字典中删除对应的键值对:
del events["2024-02-14"]
二、使用数据库
对于更大规模的数据存储和复杂查询,使用数据库是更好的选择。Python中可以使用SQLite、MySQL、PostgreSQL等数据库来管理日期和事件。
使用SQLite数据库
SQLite是一个轻量级的数据库,适合嵌入式应用和小型项目。我们可以使用SQLite来存储和查询日期事件。
安装SQLite
首先,确保你已经安装了SQLite。可以通过pip安装SQLite的Python库:
pip install sqlite3
创建数据库和表
创建一个SQLite数据库,并创建一个表来存储日期和事件。
import sqlite3
连接到SQLite数据库(如果数据库不存在,会自动创建)
conn = sqlite3.connect('events.db')
创建一个游标对象
cursor = conn.cursor()
创建表
cursor.execute('''
CREATE TABLE IF NOT EXISTS events (
date TEXT PRIMARY KEY,
event TEXT NOT NULL
)
''')
提交更改并关闭连接
conn.commit()
conn.close()
添加事件
向数据库中添加新事件:
def add_event(date, event):
conn = sqlite3.connect('events.db')
cursor = conn.cursor()
cursor.execute('INSERT INTO events (date, event) VALUES (?, ?)', (date, event))
conn.commit()
conn.close()
添加一个事件
add_event("2024-02-14", "Valentine's Day")
检索事件
从数据库中检索某个日期的事件:
def get_event(date):
conn = sqlite3.connect('events.db')
cursor = conn.cursor()
cursor.execute('SELECT event FROM events WHERE date = ?', (date,))
event = cursor.fetchone()
conn.close()
if event:
return event[0]
else:
return None
检索事件
print(get_event("2024-02-14"))
更新事件
更新数据库中某个日期的事件:
def update_event(date, new_event):
conn = sqlite3.connect('events.db')
cursor = conn.cursor()
cursor.execute('UPDATE events SET event = ? WHERE date = ?', (new_event, date))
conn.commit()
conn.close()
更新事件
update_event("2024-02-14", "Updated Valentine's Day")
删除事件
从数据库中删除某个事件:
def delete_event(date):
conn = sqlite3.connect('events.db')
cursor = conn.cursor()
cursor.execute('DELETE FROM events WHERE date = ?', (date,))
conn.commit()
conn.close()
删除事件
delete_event("2024-02-14")
三、结合日历模块
Python的calendar
模块可以用于生成日历,并结合日期事件进行管理。这个方法适合需要生成日历视图并显示事件的场景。
生成日历
使用calendar
模块生成某个月的日历:
import calendar
生成2024年2月的日历
cal = calendar.TextCalendar(calendar.SUNDAY)
print(cal.formatmonth(2024, 2))
结合事件
可以结合日期事件,生成带有事件的日历。这里我们将之前的事件数据结合到日历中。
def generate_event_calendar(year, month, events):
cal = calendar.TextCalendar(calendar.SUNDAY)
month_calendar = cal.formatmonth(year, month).split('\n')
event_days = {date.split('-')[2]: event for date, event in events.items() if date.startswith(f"{year}-{month:02d}")}
for i, week in enumerate(month_calendar):
if i > 1: # Skip the header lines
days = week.split()
new_week = []
for day in days:
if day in event_days:
new_week.append(f"{day}*") # Mark event days with *
else:
new_week.append(day)
month_calendar[i] = ' '.join(new_week)
return '\n'.join(month_calendar)
添加一些事件
events = {
"2024-02-14": "Valentine's Day",
"2024-02-20": "Some Other Event"
}
生成带有事件的日历
print(generate_event_calendar(2024, 2, events))
这样,我们就可以生成一个带有标记的日历,其中包含我们添加的日期事件。
四、综合示例
最后,我们可以结合以上方法,创建一个更复杂的日期大事记管理系统。这个系统可以支持多种操作,包括添加、检索、更新和删除事件,同时可以生成带有事件标记的日历。
import sqlite3
import calendar
数据库操作
def create_db():
conn = sqlite3.connect('events.db')
cursor = conn.cursor()
cursor.execute('''
CREATE TABLE IF NOT EXISTS events (
date TEXT PRIMARY KEY,
event TEXT NOT NULL
)
''')
conn.commit()
conn.close()
def add_event(date, event):
conn = sqlite3.connect('events.db')
cursor = conn.cursor()
cursor.execute('INSERT INTO events (date, event) VALUES (?, ?)', (date, event))
conn.commit()
conn.close()
def get_event(date):
conn = sqlite3.connect('events.db')
cursor = conn.cursor()
cursor.execute('SELECT event FROM events WHERE date = ?', (date,))
event = cursor.fetchone()
conn.close()
if event:
return event[0]
else:
return None
def update_event(date, new_event):
conn = sqlite3.connect('events.db')
cursor = conn.cursor()
cursor.execute('UPDATE events SET event = ? WHERE date = ?', (new_event, date))
conn.commit()
conn.close()
def delete_event(date):
conn = sqlite3.connect('events.db')
cursor = conn.cursor()
cursor.execute('DELETE FROM events WHERE date = ?', (date,))
conn.commit()
conn.close()
def generate_event_calendar(year, month):
conn = sqlite3.connect('events.db')
cursor = conn.cursor()
cursor.execute('SELECT date, event FROM events WHERE date LIKE ?', (f'{year}-{month:02d}-%',))
events = cursor.fetchall()
conn.close()
cal = calendar.TextCalendar(calendar.SUNDAY)
month_calendar = cal.formatmonth(year, month).split('\n')
event_days = {date.split('-')[2]: event for date, event in events}
for i, week in enumerate(month_calendar):
if i > 1: # Skip the header lines
days = week.split()
new_week = []
for day in days:
if day in event_days:
new_week.append(f"{day}*") # Mark event days with *
else:
new_week.append(day)
month_calendar[i] = ' '.join(new_week)
return '\n'.join(month_calendar)
创建数据库
create_db()
添加一些事件
add_event("2024-02-14", "Valentine's Day")
add_event("2024-02-20", "Some Other Event")
生成带有事件的日历
print(generate_event_calendar(2024, 2))
通过以上代码,我们实现了一个完整的日期大事记管理系统,支持事件的增删改查,并能生成带有事件标记的日历。这种方法不仅适合处理大量的事件数据,还能提供良好的用户体验。
相关问答FAQs:
如何在Python中创建一个大事记程序?
要创建一个大事记程序,您可以使用Python的字典或列表来存储日期和相关事件。可以定义一个函数来添加事件,并使用日期格式(如YYYY-MM-DD)作为键来保持其唯一性。还可以实现一个搜索功能,以便根据日期查找特定事件。
Python支持哪些日期格式?
Python的datetime
模块支持多种日期格式,包括但不限于YYYY-MM-DD, DD/MM/YYYY和MM-DD-YYYY。您可以使用strptime
方法将字符串转换为datetime
对象,从而方便地进行日期比较和格式化。
如何处理用户输入的日期以避免错误?
处理用户输入的日期时,可以使用异常处理机制来捕获格式错误。通过使用try-except
块来验证输入的日期是否符合预期格式。此外,使用datetime
模块中的datetime.strptime()
方法可以确保输入的日期是有效的,例如,防止用户输入不存在的日期(如2023-02-30)。
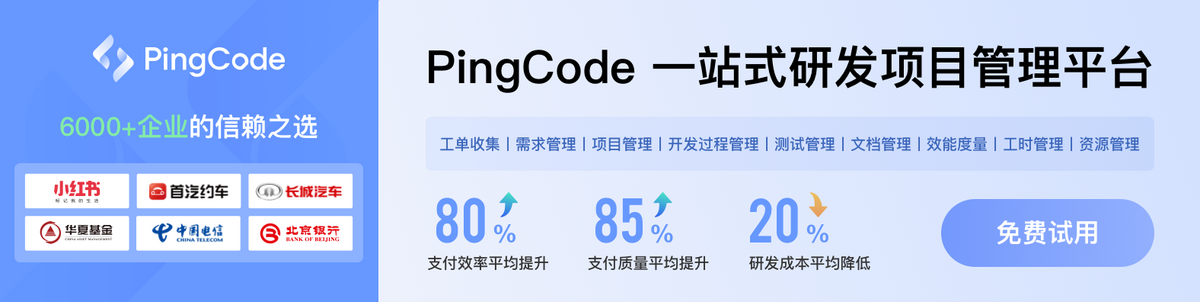