在Python 2.7中创建类可以通过使用class关键字、定义类的属性和方法、使用__init__方法进行初始化。 其中,__init__方法是一个特殊的方法,用于在创建类的实例时进行初始化。接下来,我们将详细描述如何在Python 2.7中创建类,并展示类的各种特性和用法。
一、类的定义与初始化
在Python 2.7中,类的定义非常简单。我们使用class关键字后跟类的名称,然后定义类的属性和方法。__init__方法是类的构造函数,用于初始化对象的属性。
class MyClass:
def __init__(self, attribute1, attribute2):
self.attribute1 = attribute1
self.attribute2 = attribute2
def display_attributes(self):
print("Attribute 1:", self.attribute1)
print("Attribute 2:", self.attribute2)
在这个示例中,我们定义了一个名为MyClass的类,其中包含两个属性attribute1和attribute2,以及一个方法display_attributes用于打印这些属性。
二、类的实例化
创建类的实例非常简单,只需调用类的名称并传递必要的参数给__init__方法。
# 创建类的实例
my_instance = MyClass("value1", "value2")
调用实例的方法
my_instance.display_attributes()
三、类的继承
继承是面向对象编程的重要特性。通过继承,一个类可以继承另一个类的属性和方法。继承的基本语法如下:
class ParentClass:
def __init__(self, parent_attribute):
self.parent_attribute = parent_attribute
def display_parent_attribute(self):
print("Parent Attribute:", self.parent_attribute)
class ChildClass(ParentClass):
def __init__(self, parent_attribute, child_attribute):
ParentClass.__init__(self, parent_attribute)
self.child_attribute = child_attribute
def display_child_attribute(self):
print("Child Attribute:", self.child_attribute)
在这个示例中,ChildClass继承了ParentClass,并且扩展了一个新的属性和方法。
四、多态性
多态性是面向对象编程的另一个重要特性,允许不同的类对相同的消息作出响应。在Python中,多态性通过方法重写实现。
class Animal:
def sound(self):
raise NotImplementedError("Subclasses should implement this method.")
class Dog(Animal):
def sound(self):
return "Bark"
class Cat(Animal):
def sound(self):
return "Meow"
使用多态性
def make_sound(animal):
print(animal.sound())
dog = Dog()
cat = Cat()
make_sound(dog) # 输出: Bark
make_sound(cat) # 输出: Meow
五、类的属性和方法
在Python 2.7中,类的属性和方法可以分为实例属性、类属性、实例方法和类方法。
- 实例属性和实例方法:实例属性是通过__init__方法定义的,而实例方法是通过第一个参数为self的方法定义的。
class ExampleClass:
def __init__(self, value):
self.instance_attribute = value
def instance_method(self):
return self.instance_attribute
- 类属性和类方法:类属性是直接在类内部定义的,而类方法则使用@classmethod装饰器并通过cls参数传递。
class ExampleClass:
class_attribute = "class value"
def __init__(self, value):
self.instance_attribute = value
@classmethod
def class_method(cls):
return cls.class_attribute
六、静态方法
静态方法使用@staticmethod装饰器定义,不需要传递self或cls参数。它们通常用于封装类内部的实用函数。
class ExampleClass:
@staticmethod
def static_method(value):
return value * 2
七、私有属性和方法
在Python中,通过在属性或方法名前加双下划线实现私有化。私有属性和方法只能在类内部访问,不能被类外部直接访问。
class ExampleClass:
def __init__(self, value):
self.__private_attribute = value
def __private_method(self):
return self.__private_attribute
def public_method(self):
return self.__private_method()
八、类的魔法方法
魔法方法是Python中的特殊方法,用于实现类的特定行为,如算术运算、比较运算等。常见的魔法方法包括__str__、repr、add、__eq__等。
class ExampleClass:
def __init__(self, value):
self.value = value
def __str__(self):
return "ExampleClass with value: {}".format(self.value)
def __add__(self, other):
return ExampleClass(self.value + other.value)
def __eq__(self, other):
return self.value == other.value
九、类的装饰器
类装饰器可以用于增强类的功能。常见的类装饰器包括@property、@classmethod和@staticmethod。
class ExampleClass:
def __init__(self, value):
self._value = value
@property
def value(self):
return self._value
@value.setter
def value(self, new_value):
self._value = new_value
十、示例总结
以下是一个综合示例,展示了如何在Python 2.7中创建和使用类。
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def display_info(self):
print("Name:", self.name)
print("Age:", self.age)
class Employee(Person):
def __init__(self, name, age, employee_id):
Person.__init__(self, name, age)
self.employee_id = employee_id
def display_info(self):
Person.display_info(self)
print("Employee ID:", self.employee_id)
创建实例并调用方法
person = Person("Alice", 30)
employee = Employee("Bob", 40, "E123")
person.display_info()
employee.display_info()
通过以上示例和解释,相信你已经掌握了在Python 2.7中创建类的基本方法和技巧。希望这些内容能够帮助你在实际编程中更好地使用Python类。
相关问答FAQs:
如何在Python 2.7中定义一个简单的类?
在Python 2.7中,创建一个简单的类非常容易。可以使用class
关键字来定义类,并且可以在类中定义方法和属性。例如:
class MyClass:
def __init__(self, name):
self.name = name
def greet(self):
return "Hello, " + self.name
上述代码定义了一个MyClass
,它有一个初始化方法和一个问候方法。
类中的属性和方法如何使用?
在Python 2.7中,可以通过实例化类并访问其属性和方法来使用类。例如:
my_instance = MyClass("Alice")
print(my_instance.greet()) # 输出: Hello, Alice
这样,通过创建类的实例my_instance
,就可以调用类中的方法,并访问属性。
如何在Python 2.7中继承一个类?
类的继承可以让你创建一个新的类,该类可以继承父类的属性和方法。以下是一个简单的继承示例:
class Animal:
def speak(self):
return "Animal speaks"
class Dog(Animal):
def speak(self):
return "Woof!"
在这个例子中,Dog
类继承了Animal
类,重写了speak
方法。可以通过实例化Dog
类来调用这个方法。
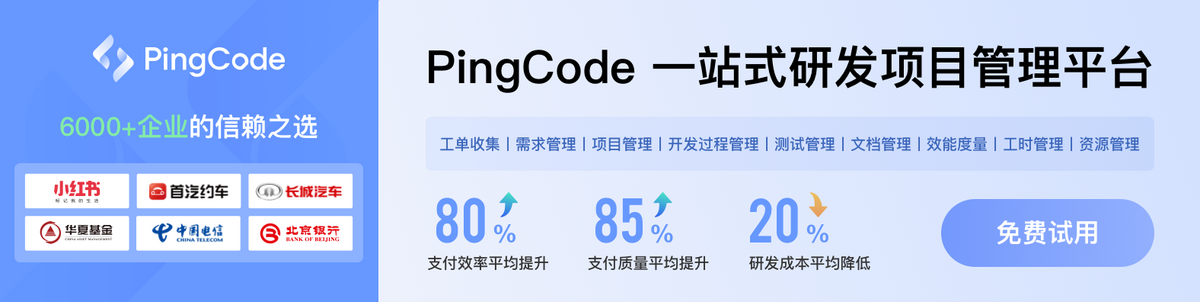