在Python中查找数组中是否存在某个元素的方法有多种:使用in关键字、使用for循环遍历、使用列表推导式、使用内置函数index()、使用set数据结构。 其中,使用in
关键字是最简单和最常用的方法。比如:if element in array:
会返回True或False。详细讲解:in
关键字是最简洁的方式,但如果需要找到元素的位置,可以使用index()
方法,该方法会返回元素的索引。
下面将详细介绍Python中查找数组元素的各种方法:
一、使用in
关键字
这是最简单和最直观的方法,用于判断元素是否存在于数组中:
array = [1, 2, 3, 4, 5]
element = 3
if element in array:
print(f"{element} is in the array.")
else:
print(f"{element} is not in the array.")
在这个例子中,in
关键字直接检查element
是否在array
中,并返回布尔值。这种方法的优点是简洁明了,适合快速判断元素存在性。
二、使用for
循环遍历
如果需要对数组进行某种操作或者进一步处理,可以使用for
循环进行遍历查找:
array = [1, 2, 3, 4, 5]
element = 3
found = False
for item in array:
if item == element:
found = True
break
if found:
print(f"{element} is in the array.")
else:
print(f"{element} is not in the array.")
这种方法适合在查找过程中需要进行其他操作的场景。例如,可以在找到元素时立即进行某种处理。
三、使用列表推导式
列表推导式是一种简洁的方式来创建列表,同时也可以用来查找元素:
array = [1, 2, 3, 4, 5]
element = 3
found = [item for item in array if item == element]
if found:
print(f"{element} is in the array.")
else:
print(f"{element} is not in the array.")
列表推导式方法优雅且高效,适合在对列表进行过滤和查找的同时进行其他操作。
四、使用内置函数index()
如果需要找到元素的索引,可以使用index()
函数:
array = [1, 2, 3, 4, 5]
element = 3
try:
index = array.index(element)
print(f"{element} is in the array at index {index}.")
except ValueError:
print(f"{element} is not in the array.")
index()
方法在找到元素时会返回其索引,但如果元素不存在,则会引发ValueError
异常。因此,使用try
和except
块来处理这种情况。
五、使用set
数据结构
如果数组包含大量元素,且查找操作频繁,可以考虑使用set
数据结构。set
具有快速查找特性:
array = [1, 2, 3, 4, 5]
element = 3
array_set = set(array)
if element in array_set:
print(f"{element} is in the array.")
else:
print(f"{element} is not in the array.")
set
的查找时间复杂度为O(1),适合大规模数据的查找。
六、使用numpy
库
对于数值数组,尤其是大型数组,可以使用numpy
库,该库提供了高效的数组操作:
import numpy as np
array = np.array([1, 2, 3, 4, 5])
element = 3
if element in array:
print(f"{element} is in the array.")
else:
print(f"{element} is not in the array.")
numpy
数组操作速度快,适合处理大量数值数据。
七、使用any()
函数结合生成器表达式
如果需要高效判断,可以使用any()
函数:
array = [1, 2, 3, 4, 5]
element = 3
found = any(item == element for item in array)
if found:
print(f"{element} is in the array.")
else:
print(f"{element} is not in the array.")
any()
函数与生成器表达式结合使用,能够在找到第一个匹配项时立即停止迭代,提升性能。
八、使用filter()
函数
filter()
函数也可以用来查找元素:
array = [1, 2, 3, 4, 5]
element = 3
found = list(filter(lambda x: x == element, array))
if found:
print(f"{element} is in the array.")
else:
print(f"{element} is not in the array.")
filter()
函数返回一个迭代器,适合进一步处理过滤后的结果。
九、组合使用多种方法
在实际应用中,可能需要组合使用多种方法。例如,在大数组中进行快速查找,同时记录元素出现的位置:
array = [1, 2, 3, 4, 5, 3, 2, 1]
element = 3
indices = [i for i, x in enumerate(array) if x == element]
if indices:
print(f"{element} is in the array at indices {indices}.")
else:
print(f"{element} is not in the array.")
这种方法结合了列表推导式和enumerate()
函数,能够高效地找到所有匹配项的位置。
十、查找多种元素
有时需要查找多个元素,可以使用集合操作:
array = [1, 2, 3, 4, 5]
elements = {2, 3}
found_elements = elements.intersection(array)
if found_elements:
print(f"Found elements: {found_elements}")
else:
print("No elements found.")
这种方法使用集合的交集操作,适合查找多个元素。
十一、查找复杂数据结构中的元素
在处理复杂数据结构(如嵌套列表或字典)时,可以使用递归函数:
def find_element(data, target):
if isinstance(data, list):
for item in data:
if find_element(item, target):
return True
elif isinstance(data, dict):
for key, value in data.items():
if find_element(key, target) or find_element(value, target):
return True
else:
return data == target
return False
data = [1, 2, [3, 4], {'a': 5, 'b': 6}]
element = 3
if find_element(data, element):
print(f"{element} is in the data structure.")
else:
print(f"{element} is not in the data structure.")
这种方法使用递归函数,能够处理任意复杂的数据结构。
十二、查找自定义对象
如果数组包含自定义对象,可以使用__eq__()
方法进行比较:
class MyObject:
def __init__(self, value):
self.value = value
def __eq__(self, other):
return self.value == other.value
array = [MyObject(1), MyObject(2), MyObject(3)]
element = MyObject(3)
if element in array:
print(f"Object with value {element.value} is in the array.")
else:
print(f"Object with value {element.value} is not in the array.")
这种方法需要在自定义类中实现__eq__()
方法,以便进行对象比较。
十三、查找和替换元素
有时查找元素后需要进行替换,可以结合list
的索引操作:
array = [1, 2, 3, 4, 5]
element = 3
new_element = 9
try:
index = array.index(element)
array[index] = new_element
print(f"Replaced {element} with {new_element} in the array.")
except ValueError:
print(f"{element} is not in the array.")
这种方法适合需要查找并替换元素的场景。
十四、结合正则表达式查找
在处理字符串数组时,可以结合正则表达式进行查找:
import re
array = ['apple', 'banana', 'cherry']
pattern = re.compile(r'ban')
found = [item for item in array if pattern.search(item)]
if found:
print(f"Found items: {found}")
else:
print("No items found.")
正则表达式提供了强大的字符串匹配功能,适合复杂模式的查找。
十五、总结
在Python中查找数组中存在的元素可以通过多种方法实现,选择合适的方法取决于具体的应用场景和性能要求。使用in
关键字是最简单的方式,适合快速判断元素存在性;使用index()
方法可以获取元素索引;使用set
数据结构适合大规模数据的高效查找;numpy
库适合处理数值数组;结合正则表达式可以处理复杂字符串查找。
希望本文提供的方法和示例能够帮助您在不同的应用场景中高效地查找数组中的元素。
相关问答FAQs:
如何使用Python查找数组中的特定元素?
要在Python中查找数组中的特定元素,可以使用in
关键字或list.index()
方法。如果你只是想检查元素是否存在,可以使用element in array
的形式。如果想获取元素的索引,可以使用array.index(element)
,但请注意,如果元素不存在,会抛出一个ValueError
。
Python中的数组与列表有什么区别?
在Python中,数组通常指的是使用array
模块或numpy
库创建的数组,而列表是Python内置的一个数据结构。数组更适合进行数值计算,提供了更多的功能和性能优化,而列表则更灵活,可以存储不同类型的元素。根据你的需求选择合适的结构可以提高程序的效率。
如何处理查找中不存在的元素?
在查找数组中不存在的元素时,可以使用in
关键字进行检查,避免直接使用index()
方法。通过条件判断可以有效防止抛出错误。例如,可以使用如下代码:if element in array: index = array.index(element)
,这样可以确保在查找索引之前,元素确实存在于数组中。
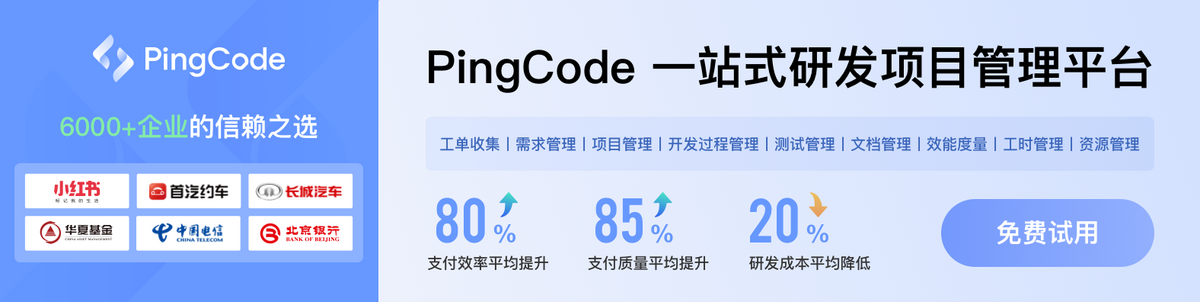