要在Python中画出相交的圆,可以使用多个库,如matplotlib、pyplot和shapely,其中matplotlib和pyplot是最常用的。首先,我们将简要介绍如何使用这些库,然后详细描述如何实现这一目标。
使用matplotlib和pyplot、引入库、定义圆的参数。这些步骤将帮助你绘制出相交的圆。下面我们将详细描述如何使用这些库来绘制相交的圆。
一、引入库
在使用matplotlib和pyplot绘制相交的圆之前,首先需要引入必要的库。我们将使用以下库:
matplotlib
: 用于绘制图形。pyplot
: 是matplotlib的一部分,提供了更简单的接口。numpy
: 用于进行数值计算。
import matplotlib.pyplot as plt
import numpy as np
二、定义圆的参数
为了绘制圆,我们需要定义圆的中心和半径。我们可以使用圆的方程来实现这一点。圆的方程为:
[ (x – h)^2 + (y – k)^2 = r^2 ]
其中 (h, k)
是圆的中心,r
是半径。
circle1 = {'center': (1, 1), 'radius': 1}
circle2 = {'center': (2, 1), 'radius': 1}
三、绘制圆
我们将使用matplotlib的pyplot
模块来绘制圆。我们可以使用以下步骤来实现这一目标:
- 创建一个新的图形。
- 添加圆到图形中。
- 显示图形。
fig, ax = plt.subplots()
创建圆
circle1_plot = plt.Circle(circle1['center'], circle1['radius'], edgecolor='b', facecolor='none')
circle2_plot = plt.Circle(circle2['center'], circle2['radius'], edgecolor='r', facecolor='none')
添加圆到图形中
ax.add_patch(circle1_plot)
ax.add_patch(circle2_plot)
设置图形的范围
ax.set_xlim(0, 3)
ax.set_ylim(0, 2)
显示图形
plt.gca().set_aspect('equal', adjustable='box')
plt.show()
在上面的代码中,我们创建了两个圆,并将它们添加到图形中。我们还设置了图形的范围,以确保圆完全显示。
四、计算圆的交点
如果你需要计算圆的交点,可以使用以下步骤:
- 计算两个圆的距离。
- 使用几何方法计算交点。
下面是计算圆的交点的代码:
def find_intersection(circle1, circle2):
x1, y1 = circle1['center']
x2, y2 = circle2['center']
r1 = circle1['radius']
r2 = circle2['radius']
d = np.sqrt((x2 - x1)<strong>2 + (y2 - y1)</strong>2)
if d > r1 + r2:
return None
elif d < abs(r1 - r2):
return None
a = (r1<strong>2 - r2</strong>2 + d2) / (2 * d)
h = np.sqrt(r1<strong>2 - a</strong>2)
x3 = x1 + a * (x2 - x1) / d
y3 = y1 + a * (y2 - y1) / d
intersection1 = (x3 + h * (y2 - y1) / d, y3 - h * (x2 - x1) / d)
intersection2 = (x3 - h * (y2 - y1) / d, y3 + h * (x2 - x1) / d)
return intersection1, intersection2
intersections = find_intersection(circle1, circle2)
print(intersections)
这段代码计算了两个圆的交点,并打印出结果。如果两个圆没有交点,函数将返回None
。
五、绘制交点
我们可以使用pyplot
模块绘制交点。以下是绘制交点的代码:
fig, ax = plt.subplots()
创建圆
circle1_plot = plt.Circle(circle1['center'], circle1['radius'], edgecolor='b', facecolor='none')
circle2_plot = plt.Circle(circle2['center'], circle2['radius'], edgecolor='r', facecolor='none')
添加圆到图形中
ax.add_patch(circle1_plot)
ax.add_patch(circle2_plot)
绘制交点
if intersections:
for point in intersections:
ax.plot(point[0], point[1], 'go')
设置图形的范围
ax.set_xlim(0, 3)
ax.set_ylim(0, 2)
显示图形
plt.gca().set_aspect('equal', adjustable='box')
plt.show()
在上面的代码中,我们绘制了交点,并将其标记为绿色点。
六、总结
通过以上步骤,我们可以使用Python中的matplotlib
和pyplot
库绘制相交的圆。我们还可以计算圆的交点,并将其绘制在图形中。以下是完整的代码示例:
import matplotlib.pyplot as plt
import numpy as np
circle1 = {'center': (1, 1), 'radius': 1}
circle2 = {'center': (2, 1), 'radius': 1}
def find_intersection(circle1, circle2):
x1, y1 = circle1['center']
x2, y2 = circle2['center']
r1 = circle1['radius']
r2 = circle2['radius']
d = np.sqrt((x2 - x1)<strong>2 + (y2 - y1)</strong>2)
if d > r1 + r2:
return None
elif d < abs(r1 - r2):
return None
a = (r1<strong>2 - r2</strong>2 + d2) / (2 * d)
h = np.sqrt(r1<strong>2 - a</strong>2)
x3 = x1 + a * (x2 - x1) / d
y3 = y1 + a * (y2 - y1) / d
intersection1 = (x3 + h * (y2 - y1) / d, y3 - h * (x2 - x1) / d)
intersection2 = (x3 - h * (y2 - y1) / d, y3 + h * (x2 - x1) / d)
return intersection1, intersection2
intersections = find_intersection(circle1, circle2)
print(intersections)
fig, ax = plt.subplots()
创建圆
circle1_plot = plt.Circle(circle1['center'], circle1['radius'], edgecolor='b', facecolor='none')
circle2_plot = plt.Circle(circle2['center'], circle2['radius'], edgecolor='r', facecolor='none')
添加圆到图形中
ax.add_patch(circle1_plot)
ax.add_patch(circle2_plot)
绘制交点
if intersections:
for point in intersections:
ax.plot(point[0], point[1], 'go')
设置图形的范围
ax.set_xlim(0, 3)
ax.set_ylim(0, 2)
显示图形
plt.gca().set_aspect('equal', adjustable='box')
plt.show()
通过这种方式,我们可以轻松地在Python中绘制相交的圆,并标记它们的交点。这些步骤提供了一个清晰的方法来处理圆的绘图和交点计算,可以帮助你更好地理解和应用这些技术。
相关问答FAQs:
如何使用Python绘制相交圆的图形?
可以使用Python的Matplotlib库来绘制相交的圆。首先,确保已经安装了Matplotlib库。接下来,您可以定义圆的参数(如中心坐标和半径),并使用Circle
类将其添加到图形中。通过设置适当的坐标轴范围和图形比例,您可以清晰地展示这些圆的交点。
在相交圆的图中,如何标记交点?
为标记交点,可以通过计算两个圆的交点坐标来实现。这通常涉及到解方程。找到交点后,可以在图中使用plt.scatter()
方法将其标记出来,选择合适的颜色和样式,以便与圆的颜色形成对比,使交点更加明显。
绘制相交圆时,有哪些参数可以调整以改善视觉效果?
在绘制相交圆时,可以调整多个参数来增强视觉效果。例如,您可以改变圆的颜色、线条宽度和透明度,以确保它们在相交时不会混淆。此外,设置背景颜色和坐标轴标签也能使图形更具吸引力和易于理解。
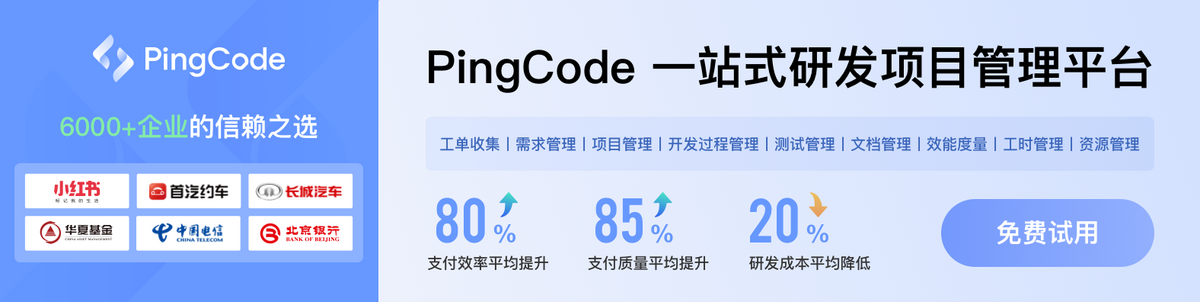