如何用Python读取SQL文件是否存在
用Python读取SQL文件是否存在的步骤包括:使用合适的数据库连接库、构建SQL查询、执行SQL查询、处理查询结果。 下面将详细描述如何实现这些步骤。
一、使用合适的数据库连接库
Python提供了许多数据库连接库,这些库支持不同类型的数据库。常见的库包括MySQL的mysql-connector-python
、PostgreSQL的psycopg2
、SQLite的sqlite3
等。选择适合你的数据库类型的库,并确保安装了相关库。
安装所需的库:
pip install mysql-connector-python psycopg2 sqlite3
二、构建SQL查询
构建一个SQL查询来检查SQL文件是否存在。假设我们要检查的SQL文件包含创建表的语句,我们可以通过查询数据库中的表信息来确认表是否存在。以下是一个简单的SQL查询示例:
SELECT table_name FROM information_schema.tables WHERE table_name = 'your_table_name';
三、执行SQL查询
使用Python连接到数据库并执行SQL查询。根据所用的数据库类型,连接和执行查询的代码会有所不同。以下是一些示例代码。
使用MySQL:
import mysql.connector
def check_table_exists(connection, table_name):
cursor = connection.cursor()
cursor.execute(f"SELECT table_name FROM information_schema.tables WHERE table_name = '{table_name}';")
result = cursor.fetchone()
return result is not None
connection = mysql.connector.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
table_exists = check_table_exists(connection, "your_table_name")
print(f"Table exists: {table_exists}")
使用PostgreSQL:
import psycopg2
def check_table_exists(connection, table_name):
cursor = connection.cursor()
cursor.execute(f"SELECT table_name FROM information_schema.tables WHERE table_name = '{table_name}';")
result = cursor.fetchone()
return result is not None
connection = psycopg2.connect(
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
table_exists = check_table_exists(connection, "your_table_name")
print(f"Table exists: {table_exists}")
使用SQLite:
import sqlite3
def check_table_exists(connection, table_name):
cursor = connection.cursor()
cursor.execute(f"SELECT name FROM sqlite_master WHERE type='table' AND name='{table_name}';")
result = cursor.fetchone()
return result is not None
connection = sqlite3.connect("yourdatabase.db")
table_exists = check_table_exists(connection, "your_table_name")
print(f"Table exists: {table_exists}")
四、处理查询结果
在查询执行后,结果将包含表是否存在的信息。根据结果,可以进一步处理逻辑,例如创建表、插入数据等。
总结
通过使用Python读取SQL文件是否存在,可以有效管理和维护数据库。选择适合的数据库连接库、构建并执行SQL查询、处理查询结果是关键步骤。通过详细描述每个步骤,可以帮助开发者更好地理解和实现这一过程。
五、具体应用场景
1、自动化数据库管理
在实际应用中,经常需要自动化管理数据库表。例如,在部署新版本的应用程序时,可能需要创建或更新数据库表。通过编写Python脚本,可以自动检查表是否存在,并根据需要执行相应的操作。
def manage_table(connection, table_name, create_table_sql):
if not check_table_exists(connection, table_name):
cursor = connection.cursor()
cursor.execute(create_table_sql)
connection.commit()
print(f"Table {table_name} created.")
else:
print(f"Table {table_name} already exists.")
Example usage
create_table_sql = """
CREATE TABLE your_table_name (
id INT AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(255) NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
"""
manage_table(connection, "your_table_name", create_table_sql)
2、数据迁移和备份
在进行数据迁移和备份时,检查表是否存在是一个重要步骤。通过Python脚本,可以自动化这个过程,确保数据的完整性和一致性。
def backup_table(connection, table_name, backup_file):
if check_table_exists(connection, table_name):
cursor = connection.cursor()
cursor.execute(f"SELECT * FROM {table_name}")
rows = cursor.fetchall()
with open(backup_file, 'w') as f:
for row in rows:
f.write(','.join(map(str, row)) + '\n')
print(f"Backup of table {table_name} completed.")
else:
print(f"Table {table_name} does not exist.")
Example usage
backup_file = "your_table_name_backup.csv"
backup_table(connection, "your_table_name", backup_file)
六、错误处理和日志记录
在实际应用中,错误处理和日志记录是必不可少的。通过捕获异常和记录日志,可以提高脚本的健壮性和可维护性。
import logging
logging.basicConfig(filename='database_operations.log', level=logging.INFO)
def check_table_exists(connection, table_name):
try:
cursor = connection.cursor()
cursor.execute(f"SELECT table_name FROM information_schema.tables WHERE table_name = '{table_name}';")
result = cursor.fetchone()
return result is not None
except Exception as e:
logging.error(f"Error checking table {table_name}: {e}")
return False
def manage_table(connection, table_name, create_table_sql):
try:
if not check_table_exists(connection, table_name):
cursor = connection.cursor()
cursor.execute(create_table_sql)
connection.commit()
logging.info(f"Table {table_name} created.")
else:
logging.info(f"Table {table_name} already exists.")
except Exception as e:
logging.error(f"Error managing table {table_name}: {e}")
Example usage
manage_table(connection, "your_table_name", create_table_sql)
七、性能优化
对于大规模数据库和高并发应用,性能优化是非常重要的。可以通过以下方法提高性能:
1、使用连接池
连接池可以复用数据库连接,减少连接创建和销毁的开销。许多数据库连接库都支持连接池。
from mysql.connector import pooling
connection_pool = mysql.connector.pooling.MySQLConnectionPool(
pool_name="mypool",
pool_size=5,
host="localhost",
user="yourusername",
password="yourpassword",
database="yourdatabase"
)
connection = connection_pool.get_connection()
manage_table(connection, "your_table_name", create_table_sql)
2、批量操作
对于插入、更新和删除等操作,批量操作可以显著提高性能。以下是一个批量插入数据的示例:
def batch_insert_data(connection, table_name, data):
try:
cursor = connection.cursor()
insert_query = f"INSERT INTO {table_name} (column1, column2) VALUES (%s, %s)"
cursor.executemany(insert_query, data)
connection.commit()
logging.info(f"Batch insert into table {table_name} completed.")
except Exception as e:
logging.error(f"Error batch inserting data into table {table_name}: {e}")
Example usage
data = [(value1, value2), (value3, value4), ...]
batch_insert_data(connection, "your_table_name", data)
八、总结
通过上述步骤,可以使用Python有效地读取SQL文件并检查其是否存在。选择合适的数据库连接库、构建并执行SQL查询、处理查询结果是关键步骤。同时,自动化数据库管理、数据迁移和备份、错误处理和日志记录、性能优化等方面的实践,可以提高脚本的健壮性和效率。在实际应用中,根据具体需求和场景,灵活应用这些方法和技巧,可以更好地管理和维护数据库。
相关问答FAQs:
如何检查一个SQL文件是否存在?
在Python中,可以使用os
模块来检查文件是否存在。通过os.path.exists()
函数,可以轻松判断SQL文件的路径是否有效。例如,您可以编写如下代码:
import os
file_path = 'path/to/your/file.sql'
if os.path.exists(file_path):
print("文件存在")
else:
print("文件不存在")
这种方法简单有效,适用于任何类型的文件。
在读取SQL文件时,如何处理文件不存在的情况?
在读取SQL文件之前,确保使用条件语句来验证文件是否存在。这可以避免因文件缺失而导致的错误。例如,您可以在尝试打开文件之前进行检查,确保程序的稳定性:
if os.path.exists(file_path):
with open(file_path, 'r') as file:
sql_content = file.read()
else:
print("无法读取文件,文件不存在。")
这种做法能够有效地处理文件缺失的情况,确保您的代码不会因为找不到文件而崩溃。
用Python读取SQL文件的最佳实践是什么?
在读取SQL文件时,建议使用上下文管理器(with
语句),这样可以确保文件在使用后能够被正确关闭。此外,处理文件时可以考虑使用异常处理机制,捕捉可能出现的错误。例如:
try:
with open(file_path, 'r') as file:
sql_content = file.read()
except FileNotFoundError:
print("指定的SQL文件未找到。")
except Exception as e:
print(f"发生错误:{e}")
通过这种方式,您可以有效管理文件读取过程中的潜在问题,确保代码的健壮性。
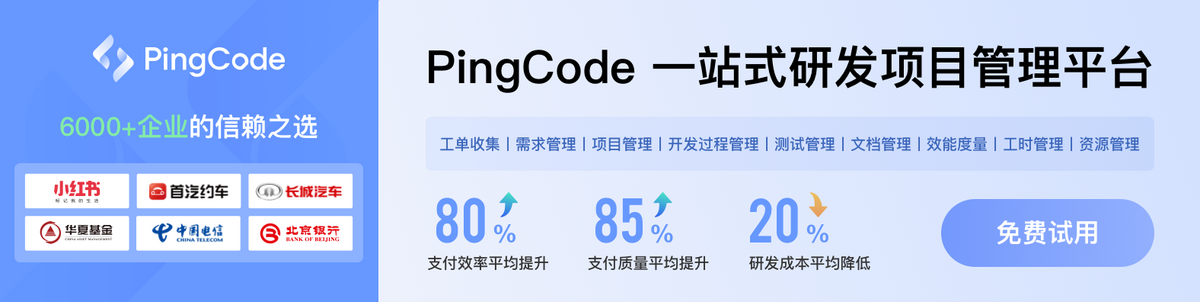