Python输出字符串个数有几种方法、使用内置函数count()、使用for循环、使用collections库中的Counter类。下面我将详细解释其中一种方法:使用内置函数count(),因为它最简单且高效。
使用内置函数count()可以快速统计字符串中某个字符或子字符串的出现次数。它的语法是:str.count(sub, start= 0,end=len(string))
。其中,sub
是要统计的子字符串,start
和end
是可选参数,表示统计范围。默认情况下,统计整个字符串中的子字符串个数。
# 示例代码
text = "hello world"
char_count = text.count('o')
print(f"'o' 在字符串中出现了 {char_count} 次")
在上面的示例中,字符串 "hello world" 中字符 'o' 出现了2次,count()
函数直接给出了结果。
一、使用内置函数count()
Python 提供了许多内置函数,其中 count()
是用于统计字符串中某个字符或子字符串出现次数的一个非常方便的函数。它不仅简洁,而且高效,特别适用于需要快速解决问题的场景。
1、count() 的基本用法
count()
函数的基本用法非常简单。它接受三个参数,其中前两个是必须的,最后一个是可选的。
sub
:要统计的子字符串。start
:可选参数,开始统计的位置,默认为0。end
:可选参数,结束统计的位置,默认为字符串的末尾。
text = "hello world, hello Python"
substring = "hello"
count = text.count(substring)
print(f"子字符串 '{substring}' 在字符串中出现了 {count} 次")
2、统计字符出现次数
有时我们不仅需要统计子字符串,还需要统计单个字符的出现次数。这在处理文本分析、数据清洗等任务中非常常见。
text = "hello world"
char = 'o'
char_count = text.count(char)
print(f"字符 '{char}' 在字符串中出现了 {char_count} 次")
3、指定统计范围
count()
函数还允许我们指定统计的范围,这在处理长文本时非常有用。例如,我们只想统计字符串的前半部分或某一特定区间内的子字符串出现次数。
text = "hello world, hello Python"
substring = "hello"
count = text.count(substring, 0, 12)
print(f"子字符串 '{substring}' 在字符串的前12个字符中出现了 {count} 次")
二、使用for循环
虽然 count()
函数非常方便,但在某些场景下,我们可能需要自定义统计逻辑。这时,使用 for
循环是一个很好的选择。通过循环遍历字符串,我们可以对每个字符进行判断,从而实现字符或子字符串的统计。
1、统计单个字符
对于统计单个字符出现次数,我们可以使用一个计数器在遍历字符串时累加。
text = "hello world"
char = 'o'
count = 0
for c in text:
if c == char:
count += 1
print(f"字符 '{char}' 在字符串中出现了 {count} 次")
2、统计子字符串
统计子字符串稍微复杂一些,因为我们需要检查每一个可能的起始位置,并判断从该位置开始的子字符串是否匹配目标子字符串。
text = "hello world, hello Python"
substring = "hello"
count = 0
sub_len = len(substring)
for i in range(len(text) - sub_len + 1):
if text[i:i + sub_len] == substring:
count += 1
print(f"子字符串 '{substring}' 在字符串中出现了 {count} 次")
三、使用collections库中的Counter类
collections
库中的 Counter
类提供了一个高级的数据结构,用于计数可哈希对象。它不仅可以用于字符串,还可以用于列表等其他可迭代对象。Counter
类的使用相对复杂,但功能强大,适用于更复杂的数据统计需求。
1、基本用法
Counter
类的基本用法非常简单。我们可以直接将一个字符串传递给 Counter
类,它会返回一个字典,键是字符,值是字符出现的次数。
from collections import Counter
text = "hello world"
counter = Counter(text)
print(counter)
2、统计单个字符
通过 Counter
类,我们可以非常方便地统计单个字符的出现次数。只需访问返回的字典即可。
char = 'o'
char_count = counter[char]
print(f"字符 '{char}' 在字符串中出现了 {char_count} 次")
3、统计子字符串
统计子字符串需要一些额外的处理。我们可以通过滑动窗口的方式,将字符串分割成多个子字符串,然后使用 Counter
统计。
substring = "hello"
sub_len = len(substring)
substrings = [text[i:i + sub_len] for i in range(len(text) - sub_len + 1)]
substring_counter = Counter(substrings)
substring_count = substring_counter[substring]
print(f"子字符串 '{substring}' 在字符串中出现了 {substring_count} 次")
四、使用正则表达式
对于更复杂的字符串匹配和统计需求,正则表达式是一个强大的工具。Python 的 re
模块提供了丰富的正则表达式操作函数,可以用于匹配、搜索、替换等操作。
1、基本用法
使用 re
模块中的 findall()
函数,可以非常方便地统计字符串中匹配某个模式的子字符串出现次数。
import re
text = "hello world, hello Python"
pattern = "hello"
matches = re.findall(pattern, text)
count = len(matches)
print(f"子字符串 '{pattern}' 在字符串中出现了 {count} 次")
2、复杂模式匹配
正则表达式的强大之处在于它可以匹配复杂的模式。例如,我们可以使用正则表达式统计某种特定结构的子字符串。
pattern = r"\bhello\b"
matches = re.findall(pattern, text)
count = len(matches)
print(f"子字符串 '{pattern}' 在字符串中出现了 {count} 次")
五、使用字符串分割和长度差异
通过字符串分割和长度差异的方法,我们可以间接实现对子字符串的统计。虽然这种方法不如前几种方法直观,但在某些特定场景下非常有效。
1、分割字符串
我们可以使用 split()
函数将字符串按子字符串分割,得到的结果列表长度减去1,即为子字符串的出现次数。
text = "hello world, hello Python"
substring = "hello"
parts = text.split(substring)
count = len(parts) - 1
print(f"子字符串 '{substring}' 在字符串中出现了 {count} 次")
2、长度差异
我们还可以通过计算字符串替换前后的长度差异,来间接统计子字符串的出现次数。
text = "hello world, hello Python"
substring = "hello"
replaced_text = text.replace(substring, "")
count = (len(text) - len(replaced_text)) // len(substring)
print(f"子字符串 '{substring}' 在字符串中出现了 {count} 次")
六、使用递归方法
递归方法在很多编程问题中都能找到应用,统计字符串出现次数也不例外。虽然递归方法实现起来相对复杂,但在一些特定场景下非常有效。
1、统计单个字符
通过递归方法,我们可以实现对单个字符的统计。递归方法的基本思想是,将问题分解为规模更小的子问题,然后通过递归调用解决这些子问题。
def count_char_recursive(text, char, index=0):
if index == len(text):
return 0
if text[index] == char:
return 1 + count_char_recursive(text, char, index + 1)
else:
return count_char_recursive(text, char, index + 1)
text = "hello world"
char = 'o'
count = count_char_recursive(text, char)
print(f"字符 '{char}' 在字符串中出现了 {count} 次")
2、统计子字符串
统计子字符串的递归方法稍微复杂一些,因为我们需要在每一个可能的起始位置进行匹配判断。
def count_substring_recursive(text, substring, index=0):
sub_len = len(substring)
if index > len(text) - sub_len:
return 0
if text[index:index + sub_len] == substring:
return 1 + count_substring_recursive(text, substring, index + sub_len)
else:
return count_substring_recursive(text, substring, index + 1)
text = "hello world, hello Python"
substring = "hello"
count = count_substring_recursive(text, substring)
print(f"子字符串 '{substring}' 在字符串中出现了 {count} 次")
七、使用字符串方法实现
除了上述方法,Python 字符串类还提供了其他一些方法,可以用于实现对子字符串的统计。这些方法虽然不如 count()
直接,但在某些场景下非常有用。
1、find() 方法
find()
方法返回子字符串在字符串中的最低索引,如果子字符串不在字符串中,则返回 -1。我们可以使用 find()
方法循环查找子字符串,直到找不到为止。
text = "hello world, hello Python"
substring = "hello"
count = 0
index = text.find(substring)
while index != -1:
count += 1
index = text.find(substring, index + 1)
print(f"子字符串 '{substring}' 在字符串中出现了 {count} 次")
2、index() 方法
index()
方法与 find()
方法类似,但如果子字符串不在字符串中,会引发 ValueError
。我们可以使用 try...except
结构来处理这种情况。
text = "hello world, hello Python"
substring = "hello"
count = 0
index = 0
while True:
try:
index = text.index(substring, index)
count += 1
index += 1
except ValueError:
break
print(f"子字符串 '{substring}' 在字符串中出现了 {count} 次")
八、使用第三方库
除了 Python 标准库中的方法,我们还可以使用一些第三方库来实现对字符串的统计。这些库通常提供了更高层次的抽象和更强大的功能,适用于处理复杂的文本数据。
1、使用 nltk
库
nltk
库是自然语言处理领域的一个重要工具,它提供了丰富的文本处理功能。我们可以使用 nltk
库实现对子字符串的统计。
import nltk
text = "hello world, hello Python"
substring = "hello"
tokens = nltk.word_tokenize(text)
count = tokens.count(substring)
print(f"子字符串 '{substring}' 在字符串中出现了 {count} 次")
2、使用 pandas
库
pandas
库是数据科学领域的一个重要工具,它提供了强大的数据处理功能。我们可以使用 pandas
库实现对子字符串的统计。
import pandas as pd
text = "hello world, hello Python"
substring = "hello"
series = pd.Series(list(text))
count = series.str.contains(substring).sum()
print(f"子字符串 '{substring}' 在字符串中出现了 {count} 次")
九、总结
通过本文的详细介绍,我们了解了多种在 Python 中统计字符串或子字符串出现次数的方法。这些方法各有优缺点,适用于不同的应用场景。无论是使用内置函数、循环、正则表达式、递归方法,还是使用第三方库,都能帮助我们高效地完成字符串统计任务。
选择合适的方法进行字符串统计,不仅能提高代码的可读性和维护性,还能提升程序的执行效率。希望通过本文的学习,能够为大家在实际开发中提供有价值的参考。
相关问答FAQs:
如何在Python中计算字符串的长度?
在Python中,可以使用内置的len()
函数来计算字符串的长度。这个函数接受一个字符串作为参数,并返回该字符串中字符的数量。例如,len("Hello, World!")
会输出13,因为这句话包含13个字符,包括空格和标点符号。
我可以使用哪些方法来统计字符串中某个特定字符的出现次数?
可以使用字符串对象的count()
方法来统计特定字符或子字符串在字符串中出现的次数。比如,"banana".count("a")
会返回3,因为字母"a"在“banana”中出现了三次。这种方法非常适合快速分析文本内容。
在Python中,如何处理多行字符串以计算总字符数?
如果需要处理多行字符串,可以先将字符串分割成单独的行,然后对每一行使用len()
函数进行计算。可以通过splitlines()
方法将字符串按行分割,再通过循环遍历每一行来获取总字符数。例如:
text = """Hello
World"""
total_length = sum(len(line) for line in text.splitlines())
这段代码将返回11,计算了所有行中的字符总数。
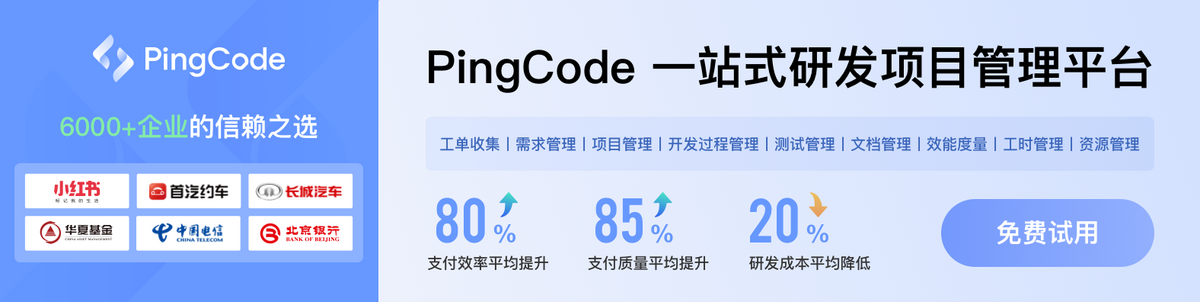