编写Python用例判断按钮居中,可以通过以下步骤:使用Selenium定位按钮元素、获取按钮和其父容器的宽度、计算按钮是否居中。 具体步骤如下:
- 使用Selenium定位按钮元素:通过XPath、CSS选择器或其他定位方式找到按钮元素。
- 获取按钮和其父容器的宽度:使用Selenium的
get_attribute
方法获取按钮和其父容器的宽度。 - 计算按钮是否居中:通过比较按钮和其父容器的宽度,判断按钮是否居中。
详细描述:首先,需要使用Selenium库,这个库可以帮助我们自动化测试Web应用程序。我们可以使用Selenium的WebDriver来定位按钮元素,并使用get_attribute方法获取按钮和其父容器的宽度。然后,通过计算按钮相对于其父容器的位置和宽度,可以判断按钮是否居中。计算方法是比较按钮的左右边距是否相等或在一个容许的误差范围内。
接下来,我们将详细介绍如何实现这些步骤。
一、安装Selenium
在开始之前,确保已安装Selenium库,可以使用pip进行安装:
pip install selenium
二、导入必要的库和配置WebDriver
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
初始化WebDriver
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
三、打开网页并定位按钮元素
# 打开目标网页
driver.get("http://example.com")
定位按钮元素
button = driver.find_element(By.XPATH, "//button[@id='targetButton']")
四、获取按钮和父容器的宽度
# 获取按钮的宽度
button_width = button.size['width']
获取按钮父容器的宽度
parent_container = button.find_element(By.XPATH, "..")
parent_width = parent_container.size['width']
五、计算按钮是否居中
# 获取按钮的左边距
button_left_margin = button.location['x'] - parent_container.location['x']
计算按钮的右边距
button_right_margin = parent_width - (button_left_margin + button_width)
判断按钮是否居中
tolerance = 5 # 容许误差值
is_centered = abs(button_left_margin - button_right_margin) <= tolerance
print("按钮是否居中:", is_centered)
六、完整代码示例
from selenium import webdriver
from selenium.webdriver.common.by import By
from selenium.webdriver.chrome.service import Service
from webdriver_manager.chrome import ChromeDriverManager
初始化WebDriver
driver = webdriver.Chrome(service=Service(ChromeDriverManager().install()))
打开目标网页
driver.get("http://example.com")
定位按钮元素
button = driver.find_element(By.XPATH, "//button[@id='targetButton']")
获取按钮的宽度
button_width = button.size['width']
获取按钮父容器的宽度
parent_container = button.find_element(By.XPATH, "..")
parent_width = parent_container.size['width']
获取按钮的左边距
button_left_margin = button.location['x'] - parent_container.location['x']
计算按钮的右边距
button_right_margin = parent_width - (button_left_margin + button_width)
判断按钮是否居中
tolerance = 5 # 容许误差值
is_centered = abs(button_left_margin - button_right_margin) <= tolerance
print("按钮是否居中:", is_centered)
关闭WebDriver
driver.quit()
七、详细解释
1. 初始化WebDriver
使用webdriver.Chrome
初始化WebDriver,并打开目标网页。这里使用webdriver_manager
库来自动管理ChromeDriver的下载和更新。
2. 定位按钮元素
通过XPath定位按钮元素。你可以根据实际情况选择其他定位方式,如CSS选择器、ID等。
3. 获取按钮和父容器的宽度
通过size
属性获取按钮和其父容器的宽度。size
属性返回一个字典,包含元素的宽度和高度。
4. 获取按钮的左边距
通过location
属性获取按钮的左边距。location
属性返回一个字典,包含元素的x和y坐标。通过计算按钮和父容器的x坐标差值,可以得到按钮的左边距。
5. 计算按钮的右边距
通过父容器的宽度减去按钮的左边距和宽度,可以得到按钮的右边距。
6. 判断按钮是否居中
通过比较按钮的左边距和右边距是否相等或在一个容许的误差范围内,可以判断按钮是否居中。这里使用abs
函数计算左边距和右边距的差值,并与容许误差值进行比较。
八、总结
通过上述步骤,我们可以编写Python用例判断按钮是否居中。关键步骤包括使用Selenium定位按钮元素、获取按钮和其父容器的宽度、计算按钮是否居中。 这种方法可以帮助我们自动化测试Web应用程序中的按钮布局,确保按钮在页面上正确居中。
这种方法不仅适用于按钮,还可以应用于其他需要居中的元素,如图片、文本框等。通过合理设置容许误差值,可以适应不同的布局要求,提高测试用例的适用性和鲁棒性。
此外,在实际应用中,我们可能还需要处理更多复杂的情况,如父容器包含多个子元素、按钮具有不同的CSS样式等。通过灵活运用Selenium和Python,我们可以编写更加复杂和健壮的测试用例,确保Web应用程序的布局和功能符合预期。
相关问答FAQs:
如何在Python中使用自动化测试框架判断按钮是否居中?
在Python中,可以使用Selenium或Pytest等自动化测试框架来编写用例。通过获取按钮的位置信息和页面宽度,可以计算按钮是否居中。具体步骤包括:1. 导入必要的库;2. 初始化浏览器驱动;3. 定位按钮元素;4. 获取按钮的位置信息和宽度;5. 计算居中条件并进行断言。
使用Selenium判断按钮居中的具体代码示例是什么?
在Selenium中,可以使用以下代码段来判断按钮是否居中:
from selenium import webdriver
driver = webdriver.Chrome()
driver.get("你的网页地址")
button = driver.find_element_by_id("按钮的ID")
button_location = button.location['x']
button_width = button.size['width']
page_width = driver.execute_script("return document.body.scrollWidth")
is_centered = (button_location + (button_width / 2)) == (page_width / 2)
assert is_centered, "按钮未居中"
driver.quit()
该代码获取按钮的位置信息并检查其是否在页面的中心位置。
判断按钮居中的用例还需要考虑哪些因素?
在编写用例时,除了判断按钮的水平居中外,还应考虑响应式设计对不同屏幕尺寸的影响。确保按钮在不同分辨率下也能保持居中。此外,若页面有其他元素,可能需要考虑它们对按钮位置的影响,确保测试覆盖全面。
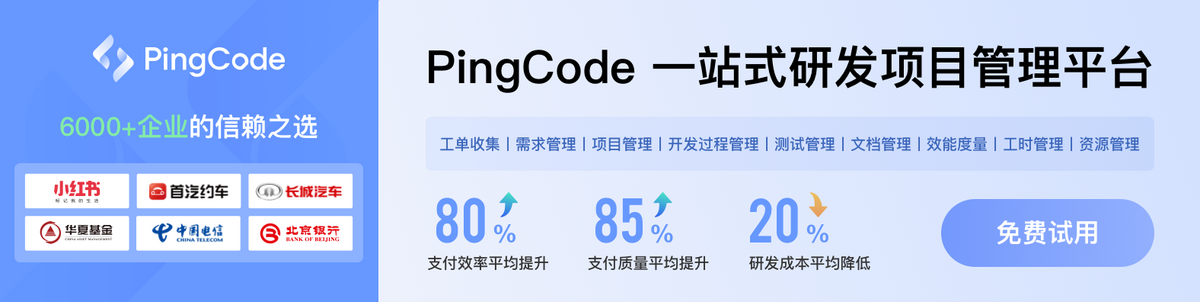