在Python中,编写电子邮件地址的步骤包括:使用适当的库、设置SMTP服务器、编写邮件内容、发送邮件。 其中,使用适当的库是最为基础且关键的一步。Python提供了多个库来处理电子邮件的发送和接收,如smtplib、email等。接下来,我们将详细介绍如何在Python中编写电子邮件地址并发送邮件。
一、使用适当的库
在Python中,最常用的库是smtplib
和email
。smtplib
用于连接SMTP服务器并发送邮件,email
库则用于构建邮件内容。以下是使用这些库的基本示例:
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
设置邮件信息
sender_email = "your_email@example.com"
receiver_email = "receiver_email@example.com"
password = "your_password"
创建邮件内容
message = MIMEMultipart("alternative")
message["Subject"] = "Test Email"
message["From"] = sender_email
message["To"] = receiver_email
编写邮件正文
text = """\
Hi,
This is a test email from Python."""
part = MIMEText(text, "plain")
message.attach(part)
发送邮件
with smtplib.SMTP_SSL("smtp.example.com", 465) as server:
server.login(sender_email, password)
server.sendmail(sender_email, receiver_email, message.as_string())
二、设置SMTP服务器
SMTP(Simple Mail Transfer Protocol)服务器是发送电子邮件的核心。不同的电子邮件服务提供商有不同的SMTP服务器地址和端口号。例如,Gmail的SMTP服务器是smtp.gmail.com
,端口号是465。要发送邮件,首先需要设置和连接到SMTP服务器:
smtp_server = "smtp.example.com" # SMTP服务器地址
port = 465 # 端口号
sender_email = "your_email@example.com"
password = "your_password"
连接到SMTP服务器
with smtplib.SMTP_SSL(smtp_server, port) as server:
server.login(sender_email, password)
# 后续发送邮件的步骤
三、编写邮件内容
邮件内容可以是简单的文本,也可以是HTML格式的内容。使用email.mime
模块中的MIMEText
和MIMEMultipart
类,可以轻松构建邮件内容。以下是编写HTML格式邮件内容的示例:
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
message = MIMEMultipart("alternative")
message["Subject"] = "Test Email"
message["From"] = sender_email
message["To"] = receiver_email
编写HTML邮件正文
html = """\
<html>
<body>
<p>Hi,<br>
This is a <b>test email</b> from Python.<br>
<a href="http://www.example.com">Visit our website</a>
</p>
</body>
</html>
"""
part = MIMEText(html, "html")
message.attach(part)
四、发送邮件
一旦邮件内容构建完成并连接到SMTP服务器,就可以发送邮件了。以下是发送邮件的完整示例代码:
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
设置邮件信息
sender_email = "your_email@example.com"
receiver_email = "receiver_email@example.com"
password = "your_password"
创建邮件内容
message = MIMEMultipart("alternative")
message["Subject"] = "Test Email"
message["From"] = sender_email
message["To"] = receiver_email
编写邮件正文
text = """\
Hi,
This is a test email from Python."""
html = """\
<html>
<body>
<p>Hi,<br>
This is a <b>test email</b> from Python.<br>
<a href="http://www.example.com">Visit our website</a>
</p>
</body>
</html>
"""
part1 = MIMEText(text, "plain")
part2 = MIMEText(html, "html")
message.attach(part1)
message.attach(part2)
发送邮件
with smtplib.SMTP_SSL("smtp.example.com", 465) as server:
server.login(sender_email, password)
server.sendmail(sender_email, receiver_email, message.as_string())
五、处理发送错误
在发送邮件的过程中,可能会遇到各种错误,例如连接失败、登录失败或发送失败等。可以使用try-except
块来处理这些错误:
try:
with smtplib.SMTP_SSL("smtp.example.com", 465) as server:
server.login(sender_email, password)
server.sendmail(sender_email, receiver_email, message.as_string())
print("Email sent successfully")
except smtplib.SMTPException as e:
print(f"Failed to send email: {e}")
六、添加附件
有时需要在邮件中添加附件,可以使用email.mime
模块中的MIMEBase
类来实现。以下是添加附件的示例代码:
from email.mime.base import MIMEBase
from email import encoders
创建邮件内容
message = MIMEMultipart()
message["Subject"] = "Email with Attachment"
message["From"] = sender_email
message["To"] = receiver_email
编写邮件正文
body = "This is an email with attachment sent from Python."
message.attach(MIMEText(body, "plain"))
添加附件
filename = "document.pdf"
with open(filename, "rb") as attachment:
part = MIMEBase("application", "octet-stream")
part.set_payload(attachment.read())
编码为base64
encoders.encode_base64(part)
part.add_header(
"Content-Disposition",
f"attachment; filename= {filename}",
)
message.attach(part)
发送邮件
try:
with smtplib.SMTP_SSL("smtp.example.com", 465) as server:
server.login(sender_email, password)
server.sendmail(sender_email, receiver_email, message.as_string())
print("Email sent successfully with attachment")
except smtplib.SMTPException as e:
print(f"Failed to send email: {e}")
七、使用HTML模板
在一些情况下,邮件内容可能非常复杂,直接在代码中编写HTML不太方便。可以使用HTML模板引擎,例如Jinja2,来生成邮件内容。以下是使用Jinja2生成邮件内容的示例:
from jinja2 import Environment, FileSystemLoader
加载模板
env = Environment(loader=FileSystemLoader('templates'))
template = env.get_template('email_template.html')
渲染模板
html_content = template.render(name="John Doe", link="http://www.example.com")
创建邮件内容
message = MIMEMultipart("alternative")
message["Subject"] = "Email with HTML Template"
message["From"] = sender_email
message["To"] = receiver_email
编写邮件正文
part = MIMEText(html_content, "html")
message.attach(part)
发送邮件
try:
with smtplib.SMTP_SSL("smtp.example.com", 465) as server:
server.login(sender_email, password)
server.sendmail(sender_email, receiver_email, message.as_string())
print("Email sent successfully with HTML template")
except smtplib.SMTPException as e:
print(f"Failed to send email: {e}")
八、安全性和隐私
在处理电子邮件和密码时,必须注意安全性和隐私。以下是一些安全建议:
- 使用环境变量:不要在代码中硬编码电子邮件和密码,使用环境变量来存储这些敏感信息。
- 加密连接:始终使用SSL/TLS加密连接来发送邮件,防止数据在传输过程中被窃取。
- 应用密码:使用电子邮件服务提供商提供的应用密码,而不是使用账户的主密码。
示例代码:
import os
从环境变量中读取电子邮件和密码
sender_email = os.getenv("SENDER_EMAIL")
password = os.getenv("EMAIL_PASSWORD")
其他代码保持不变
九、发送批量邮件
有时需要发送批量邮件,可以在代码中循环发送。以下是发送批量邮件的示例代码:
receiver_emails = ["email1@example.com", "email2@example.com", "email3@example.com"]
for receiver_email in receiver_emails:
# 创建邮件内容
message = MIMEMultipart("alternative")
message["Subject"] = "Test Email"
message["From"] = sender_email
message["To"] = receiver_email
# 编写邮件正文
text = """\
Hi,
This is a test email from Python."""
part = MIMEText(text, "plain")
message.attach(part)
# 发送邮件
try:
with smtplib.SMTP_SSL("smtp.example.com", 465) as server:
server.login(sender_email, password)
server.sendmail(sender_email, receiver_email, message.as_string())
print(f"Email sent successfully to {receiver_email}")
except smtplib.SMTPException as e:
print(f"Failed to send email to {receiver_email}: {e}")
十、处理回复邮件
有时需要处理回复邮件,可以使用imaplib
库来接收和读取邮件。以下是接收和读取邮件的示例代码:
import imaplib
import email
连接到IMAP服务器
mail = imaplib.IMAP4_SSL("imap.example.com")
mail.login(sender_email, password)
选择收件箱
mail.select("inbox")
搜索所有未读邮件
status, messages = mail.search(None, '(UNSEEN)')
获取邮件ID列表
mail_ids = messages[0].split()
for mail_id in mail_ids:
status, msg_data = mail.fetch(mail_id, '(RFC822)')
raw_email = msg_data[0][1]
msg = email.message_from_bytes(raw_email)
# 解析邮件内容
email_subject = msg["subject"]
email_from = msg["from"]
email_body = msg.get_payload(decode=True).decode()
print(f"From: {email_from}")
print(f"Subject: {email_subject}")
print(f"Body: {email_body}")
关闭连接
mail.logout()
通过以上步骤,您可以在Python中轻松编写电子邮件地址并发送邮件。希望这些示例和技巧能帮助您更好地处理电子邮件任务。
相关问答FAQs:
如何在Python中验证电子邮件地址的格式?
在Python中,可以使用正则表达式(re模块)来验证电子邮件地址的格式。通过定义一个合适的正则表达式,可以检查输入的邮箱是否符合标准格式,例如是否包含“@”符号及有效的域名部分。示例代码如下:
import re
def is_valid_email(email):
pattern = r'^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$'
return re.match(pattern, email) is not None
print(is_valid_email("example@mail.com")) # 输出: True
在Python中如何发送带附件的电子邮件?
要在Python中发送带附件的电子邮件,可以使用smtplib和email模块。首先创建邮件对象,然后添加附件,最后通过SMTP服务器发送邮件。以下是一个简单的示例:
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.base import MIMEBase
from email import encoders
def send_email_with_attachment(to_email, subject, body, filename):
from_email = "your_email@example.com"
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
msg.attach(MIMEText(body, 'plain'))
with open(filename, 'rb') as attachment:
part = MIMEBase('application', 'octet-stream')
part.set_payload(attachment.read())
encoders.encode_base64(part)
part.add_header('Content-Disposition', f'attachment; filename={filename}')
msg.attach(part)
with smtplib.SMTP('smtp.example.com', 587) as server:
server.starttls()
server.login(from_email, "your_password")
server.send_message(msg)
send_email_with_attachment("recipient@example.com", "Subject", "Email body", "file.txt")
如何在Python中使用SMTP发送电子邮件?
使用Python的smtplib模块,可以轻松发送电子邮件。需要连接SMTP服务器,登录邮箱帐号,然后构建邮件内容并发送。以下是实现的基本步骤:
- 导入smtplib模块。
- 创建SMTP对象并连接到邮件服务器。
- 登录你的邮箱帐号。
- 构建邮件内容,包括发件人、收件人、主题和正文。
- 使用sendmail方法发送邮件。
- 关闭SMTP连接。示例代码如下:
import smtplib
from email.mime.text import MIMEText
def send_email(to_email, subject, body):
from_email = "your_email@example.com"
msg = MIMEText(body)
msg['Subject'] = subject
msg['From'] = from_email
msg['To'] = to_email
with smtplib.SMTP('smtp.example.com', 587) as server:
server.starttls()
server.login(from_email, "your_password")
server.sendmail(from_email, to_email, msg.as_string())
send_email("recipient@example.com", "Test Subject", "Hello, this is a test email.")
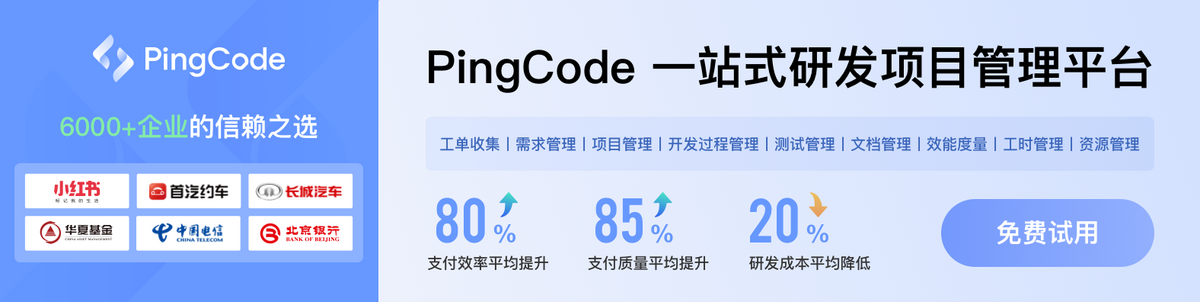