在Python中去除字符串的空格可以通过多种方法实现,例如使用strip()方法、replace()方法、re模块等。这些方法各有不同的应用场景和优缺点,其中最常用的是strip()方法、replace()方法、split()和join()方法的组合。strip()方法用于去除字符串两端的空格,replace()方法用于替换字符串中的所有空格,split()和join()方法则用于去除字符串中的所有空格。下面将详细介绍这些方法及其应用。
一、使用strip()方法
strip()方法主要用于去除字符串两端的空格。这是一个简单且高效的方法,适用于需要去除字符串开始和结束位置空格的场景。
text = " Hello, World! "
cleaned_text = text.strip()
print(cleaned_text) # 输出:Hello, World!
strip()方法还可以去除指定字符,只需在括号内传入需要去除的字符即可。
text = "###Hello, World!###"
cleaned_text = text.strip('#')
print(cleaned_text) # 输出:Hello, World!
二、使用replace()方法
replace()方法用于替换字符串中的所有空格,适用于需要去除字符串中间及两端所有空格的场景。
text = " H e l l o , W o r l d ! "
cleaned_text = text.replace(" ", "")
print(cleaned_text) # 输出:Hello,World!
replace()方法不仅可以替换空格,还可以替换其他字符。只需在第一个参数传入需要替换的字符,第二个参数传入替换后的字符即可。
text = "Hello, World!"
cleaned_text = text.replace("o", "0")
print(cleaned_text) # 输出:Hell0, W0rld!
三、使用split()和join()方法组合
split()和join()方法组合使用,可以去除字符串中的所有空格。split()方法将字符串拆分成单词列表,join()方法将其重新组合成字符串。
text = " H e l l o , W o r l d ! "
cleaned_text = "".join(text.split())
print(cleaned_text) # 输出:Hello,World!
这种方法适用于需要去除所有空格并保持其他字符不变的场景。
四、使用正则表达式去除空格
re模块提供了更强大的字符串处理能力,可以使用正则表达式去除空格。这种方法适用于复杂的字符串处理场景。
import re
text = " H e l l o , W o r l d ! "
cleaned_text = re.sub(r'\s+', '', text)
print(cleaned_text) # 输出:Hello,World!
re.sub()方法可以通过正则表达式匹配并替换字符串中的字符。r'\s+'表示匹配一个或多个空格字符。
五、去除指定位置的空格
在某些情况下,可能只需要去除字符串中的特定位置的空格。例如,只去除字符串开始位置的空格,或者只去除字符串结束位置的空格。
去除字符串开始位置的空格
text = " Hello, World! "
cleaned_text = text.lstrip()
print(cleaned_text) # 输出:Hello, World!
lstrip()方法用于去除字符串开始位置的空格。
去除字符串结束位置的空格
text = " Hello, World! "
cleaned_text = text.rstrip()
print(cleaned_text) # 输出: Hello, World!
rstrip()方法用于去除字符串结束位置的空格。
六、去除多行字符串中的空格
对于多行字符串,可以使用splitlines()方法将字符串按行拆分,然后使用前面介绍的方法去除空格。
text = """
Hello,
World!
"""
cleaned_lines = [line.strip() for line in text.splitlines()]
cleaned_text = "\n".join(cleaned_lines)
print(cleaned_text)
输出:
Hello,
World!
七、性能比较
在处理大字符串时,性能可能是一个需要考虑的重要因素。不同方法的性能可能会有所不同。一般来说,strip()方法和replace()方法的性能较高,因为它们是内置方法,经过了优化。而使用正则表达式的re.sub()方法则可能相对较慢,因为其需要进行正则表达式的解析和匹配。
可以通过以下代码进行简单的性能测试:
import time
text = " " * 1000 + "Hello, World!" + " " * 1000
测试strip()方法
start = time.time()
for _ in range(100000):
cleaned_text = text.strip()
end = time.time()
print("strip()方法耗时:", end - start)
测试replace()方法
start = time.time()
for _ in range(100000):
cleaned_text = text.replace(" ", "")
end = time.time()
print("replace()方法耗时:", end - start)
测试split()和join()方法
start = time.time()
for _ in range(100000):
cleaned_text = "".join(text.split())
end = time.time()
print("split()和join()方法耗时:", end - start)
测试re.sub()方法
import re
start = time.time()
for _ in range(100000):
cleaned_text = re.sub(r'\s+', '', text)
end = time.time()
print("re.sub()方法耗时:", end - start)
不同方法的性能可能会因字符串的长度和内容而有所不同。一般来说,strip()方法和replace()方法在大多数情况下性能较优。
八、总结
在Python中去除字符串的空格可以使用多种方法,包括strip()方法、replace()方法、split()和join()方法的组合、正则表达式等。strip()方法适用于去除字符串两端的空格,replace()方法适用于去除字符串中的所有空格,split()和join()方法的组合适用于去除字符串中的所有空格并保持其他字符不变,正则表达式适用于复杂的字符串处理场景。在选择方法时,可以根据具体需求和性能考虑进行选择。
相关问答FAQs:
在Python中如何去除字符串前后的空格?
可以使用字符串的strip()
方法来去除字符串开头和结尾的空格。例如:
my_string = " Hello, World! "
cleaned_string = my_string.strip()
print(cleaned_string) # 输出: "Hello, World!"
此方法也可以去除其他指定字符,传入字符参数即可。
如何去除Python字符串中的所有空格?
如果希望删除字符串中所有的空格,可以使用replace()
方法。示例如下:
my_string = "Hello, World!"
no_spaces = my_string.replace(" ", "")
print(no_spaces) # 输出: "Hello,World!"
这种方式会将所有的空格替换为空字符串。
在Python中如何去除字符串中间的多余空格?
要去掉字符串中间的多余空格,可以结合使用split()
和join()
方法。此方法会将字符串按空格分割成多个部分,并再以单个空格连接起来。示例代码如下:
my_string = "Hello, World! How are you?"
single_spaced = ' '.join(my_string.split())
print(single_spaced) # 输出: "Hello, World! How are you?"
这种方式可以有效地清理多余的空格,保持字符串的整洁性。
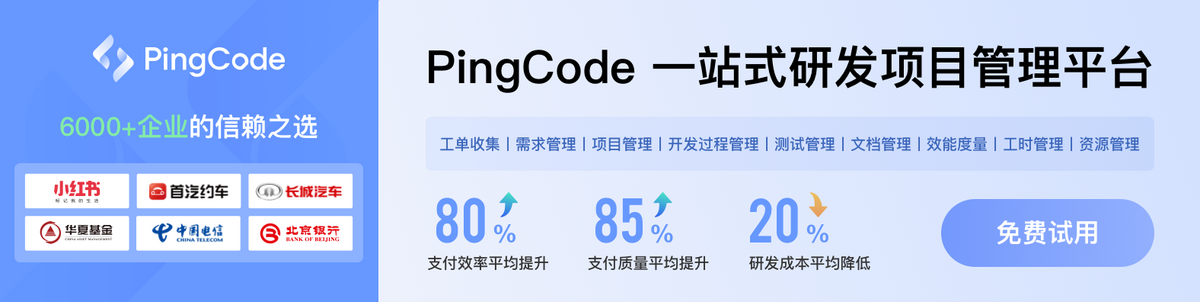