search("python中shell如何运行程序设计")
在 Python 中,可以通过多种方式在 Shell 中运行程序设计:使用交互式解释器、编写脚本文件、调用 Shell 命令、集成 Shell 脚本等。 其中,使用交互式解释器 是最直接的方式,适合测试代码片段和调试。以下将详细介绍这种方法。
使用交互式解释器
Python 提供了交互式解释器(也称为 Python Shell),允许用户逐行输入代码并立即执行,适用于快速测试和调试。要使用交互式解释器:
-
启动 Python Shell:在命令行界面输入
python
或python3
,然后按回车键,即可进入 Python Shell,显示>>>
提示符。 -
输入代码:在
>>>
提示符后输入 Python 代码,并按回车键执行。例如,输入print("Hello, World!")
,然后按回车键,屏幕上将显示Hello, World!
。 -
多行代码:对于多行代码,如定义函数或类,可以在每行末尾输入回车键,直到完成整个代码块。例如:
>>> def greet(name):
... print(f"Hello, {name}!")
...
>>> greet("Alice")
Hello, Alice!
-
退出 Shell:在 Windows 系统中,按
Ctrl + Z
,然后按回车键;在 Unix 或 Linux 系统中,按Ctrl + D
,即可退出 Python Shell。
编写和运行脚本文件
对于更复杂的程序设计,编写脚本文件是更好的选择。步骤如下:
-
创建脚本文件:使用文本编辑器创建一个扩展名为
.py
的文件,例如script.py
。 -
编写代码:在文件中输入 Python 代码。例如:
def greet(name):
print(f"Hello, {name}!")
if __name__ == "__main__":
greet("Alice")
-
运行脚本:在命令行界面,导航到脚本文件所在的目录,输入
python script.py
或python3 script.py
,然后按回车键,即可运行脚本。
在 Python 中调用 Shell 命令
在某些情况下,可能需要在 Python 程序中调用 Shell 命令。可以使用 subprocess
模块来实现。
-
导入模块:
import subprocess
-
执行命令:使用
subprocess.run()
函数执行 Shell 命令。例如,列出当前目录下的文件:result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.stdout)
在 Windows 系统中,可以使用
dir
命令:result = subprocess.run(['cmd', '/c', 'dir'], capture_output=True, text=True)
print(result.stdout)
-
处理输出和错误:
subprocess.run()
返回一个CompletedProcess
对象,包含命令的输出和错误信息。可以通过stdout
和stderr
属性访问。result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
if result.returncode == 0:
print("Command succeeded:")
print(result.stdout)
else:
print("Command failed:")
print(result.stderr)
在 Shell 脚本中调用 Python 代码
有时,需要在 Shell 脚本中调用 Python 代码。可以直接在 Shell 脚本中嵌入 Python 代码,或调用独立的 Python 脚本。
-
嵌入 Python 代码:在 Shell 脚本中使用
python -c
选项执行 Python 代码。例如:#!/bin/bash
python -c 'print("Hello from Python!")'
-
调用独立的 Python 脚本:编写一个 Python 脚本文件,然后在 Shell 脚本中调用。例如:
#!/bin/bash
python script.py
集成 Python 和 Shell 的最佳实践
在实际开发中,Python 和 Shell 的集成使用可以提高效率,但需要注意以下几点:
-
安全性:在使用
subprocess
模块调用 Shell 命令时,避免使用shell=True
,以防止 Shell 注入攻击。# 不推荐的做法
subprocess.run('ls -l', shell=True)
推荐的做法
subprocess.run(['ls', '-l'])
-
错误处理:在调用外部命令时,必须处理可能的错误。例如,检查返回码或捕获异常。
try:
result = subprocess.run(['ls', '-l'], check=True)
except subprocess.CalledProcessError as e:
print(f"Command failed with return code {e.returncode}")
-
跨平台兼容性:注意不同操作系统之间的命令差异,编写兼容性的代码。例如,列出目录内容的命令在 Unix/Linux 系统中是
ls
,而在 Windows 系统中是dir
。import os
import subprocess
if os.name == 'nt':
# Windows 系统
command = ['cmd', '/c', 'dir']
else:
# Unix/Linux 系统
command = ['ls', '-l']
result = subprocess.run(command, capture_output=True, text=True)
print(result.stdout)
-
性能考虑:频繁调用外部命令可能影响性能。在可能的情况下,优先使用 Python 内置功能或库函数。例如,使用 Python 的
os
模块操作文件和目录,而不是调用 Shell 命令。import os
使用 Python 内置函数获取当前目录内容
files = os.listdir('.')
for file in files:
print(file)
-
日志记录:在自动化脚本中,记录执行的命令和结果有助于调试和维护。可以使用 Python 的
logging
模块记录相关信息。import logging
import subprocess
logging.basicConfig(level=logging.INFO)
command = ['ls', '-l']
logging.info(f"Running command: {' '.join(command)}")
result
相关问答FAQs:
如何在Python中调用Shell命令运行程序?
在Python中,可以使用内置的subprocess
模块来调用Shell命令。通过subprocess.run()
或subprocess.Popen()
等方法,可以在Python脚本中执行Shell命令并获取输出。示例代码如下:
import subprocess
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.stdout)
这种方法允许你运行任何Shell命令,并获取其标准输出和错误信息。
在Python中如何处理Shell命令的输出?
处理Shell命令的输出可以通过subprocess.run()
中的capture_output=True
参数来实现。这使得你能够获取命令的标准输出和标准错误。通过result.stdout
和result.stderr
可以访问这些输出信息,进而进行进一步的处理或分析。
在Python中如何捕获Shell命令的错误信息?
使用subprocess.run()
时,可以通过检查返回的returncode
来判断Shell命令是否成功执行。如果返回值不为零,则表示出现了错误。同时,stderr
属性可以用来获取错误信息,例如:
if result.returncode != 0:
print("Error occurred:", result.stderr)
这样,你可以有效地捕获并处理Shell命令执行过程中的任何错误。
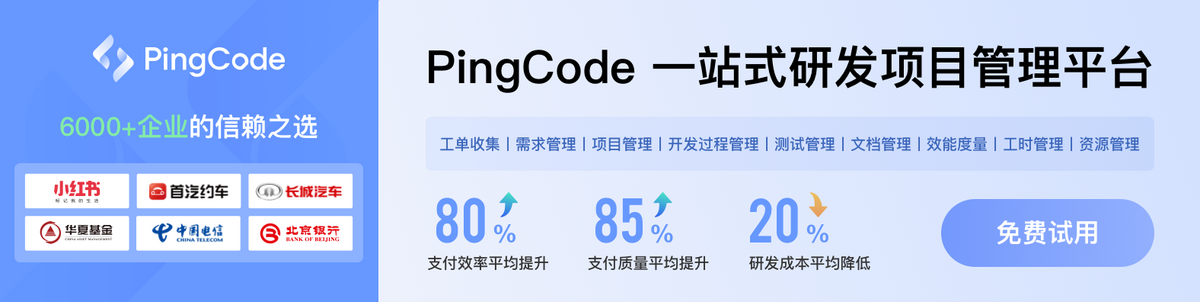